Now implement the mergesort function. In the base case (len(lst)<2), return the list itself. Otherwise, split the list into a left half and a right half recursively and use the above merge() function to combine sub-results. Note: Use integer division // when computing the length for each subproblem. from random import randint def mergesort(lst): """Implementation of the MergeSort algorithm. Precondition: lst is a list containing elements that can be compared. :param lst - a list of comparable elements :resut - a list containing all elements in lst in ascending order.""" pass # your code goes here ## wrapper to return a shuffled list, shuffle() updates the input list def shuffled(lst): result = [elem for elem in lst] ## copy lst shuffle(result) return result tests = [ [ [shuffled([n for n in range(20)])], [n for n in range(20)]], [ [[n for n in range(19,-1,1)]], sorted([n for n in range(19,-1,1)])], [ [[]], []], [ [[n for n in range(21)]], [n for n in range(21)]] ] try: for n,t in enumerate(tests): print('test',n,': ',end='') D = mergesort(*t[0]) if (D!=t[1]): print('no exception raised, but result incorrect') print('INPUT: ',t[0][0]) print('IS: ',D) print('SHOULD:',t[1]) else: print('correct') except: print('Your function raised an exception') raise
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Now implement the mergesort function. In the base case (len(lst)<2), return the list itself. Otherwise, split the list into a left half and a right half recursively and use the above merge() function to combine sub-results. Note: Use integer division // when computing the length for each subproblem.
from random import randint
def mergesort(lst):
"""Implementation of the MergeSort
Precondition: lst is a list containing elements that can be compared.
:param lst - a list of comparable elements
:resut - a list containing all elements in lst in ascending order."""
pass # your code goes here
## wrapper to return a shuffled list, shuffle() updates the input list
def shuffled(lst):
result = [elem for elem in lst] ## copy lst
shuffle(result)
return result
tests = [
[ [shuffled([n for n in range(20)])], [n for n in range(20)]],
[ [[n for n in range(19,-1,1)]], sorted([n for n in range(19,-1,1)])],
[ [[]], []],
[ [[n for n in range(21)]], [n for n in range(21)]]
]
try:
for n,t in enumerate(tests):
print('test',n,': ',end='')
D = mergesort(*t[0])
if (D!=t[1]):
print('no exception raised, but result incorrect')
print('INPUT: ',t[0][0])
print('IS: ',D)
print('SHOULD:',t[1])
else:
print('correct')
except:
print('Your function raised an exception')
raise

Step by step
Solved in 4 steps with 2 images

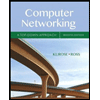
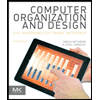
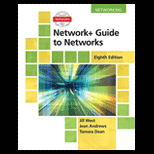
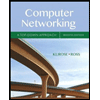
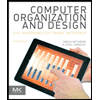
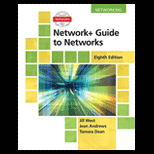
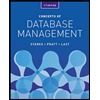
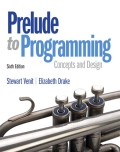
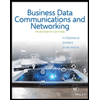