Now, in order to make all of this legal, we must have a way to compensate the musical artists for their hard work and wonderful music. An artist should receive 25 cents each time one of their songs is downloaded. Hence, for each artist, we will keep track of how much money in royalties they have. Add the following attributes to the MusicExchangeCenter class: royalties - a HashMap with the artists' names as the keys and the values are floats representing the total amount of royalties for that artist so far. It should only contain artists who have had songs downloaded. You will update this HashMap later. downloadedSongs - an ArrayList containing all of the songs that have been downloaded. This list will, in general, contain duplicate Song objects. Write a method in the MusicExchangeCenter class called displayRoyalties( that displays the royalties for all artists who have had at least one of their songs downloaded. It should display a two- line header and then one line per artist showing the royalty amount Amount Artist $0.75 $1.50 Sleepfest Clip $0.25 Jaw $0.50 Long Road $0.25 Yeah $0.25 UFO as well as the artist name as follows: In the getSong() method in the MusicExchangeCenter, adjust the code so that if the song is found (i.e., able to be downloaded), then it is added to the downloadedSongs list. Additionally, the royalties for the artist of the song should be updated within this method. Write a method in the MusicExchangeCenter class called uniqueDownloads() that returns (i.e., not displays) a TreeSet of all downloaded Song objects such that the set is sorted alphabetically by song title. There should be no duplicates songs in this set. To add the Song objects to the TreeSet, the Song class will need to implement Comparable and you will need to write the corresponding compareTo(Song s) method in the Song class. Write a method in the MusicExchangeCenter class called songsByPopularity() that returns (i.e., not displays) an ArrayList of Pair objects where the key of the pair is an Integer representing the number of downloads and the value is the Song object. The integer key should represent the number of times that the song was downloaded. The list returned should be sorted in decreasing order according to the number of times the song was downloaded. To sort a list of Pair objects, you can use the following code:
Now, in order to make all of this legal, we must have a way to compensate the musical artists for their hard work and wonderful music. An artist should receive 25 cents each time one of their songs is downloaded. Hence, for each artist, we will keep track of how much money in royalties they have. Add the following attributes to the MusicExchangeCenter class: royalties - a HashMap with the artists' names as the keys and the values are floats representing the total amount of royalties for that artist so far. It should only contain artists who have had songs downloaded. You will update this HashMap later. downloadedSongs - an ArrayList containing all of the songs that have been downloaded. This list will, in general, contain duplicate Song objects. Write a method in the MusicExchangeCenter class called displayRoyalties( that displays the royalties for all artists who have had at least one of their songs downloaded. It should display a two- line header and then one line per artist showing the royalty amount Amount Artist $0.75 $1.50 Sleepfest Clip $0.25 Jaw $0.50 Long Road $0.25 Yeah $0.25 UFO as well as the artist name as follows: In the getSong() method in the MusicExchangeCenter, adjust the code so that if the song is found (i.e., able to be downloaded), then it is added to the downloadedSongs list. Additionally, the royalties for the artist of the song should be updated within this method. Write a method in the MusicExchangeCenter class called uniqueDownloads() that returns (i.e., not displays) a TreeSet of all downloaded Song objects such that the set is sorted alphabetically by song title. There should be no duplicates songs in this set. To add the Song objects to the TreeSet, the Song class will need to implement Comparable and you will need to write the corresponding compareTo(Song s) method in the Song class. Write a method in the MusicExchangeCenter class called songsByPopularity() that returns (i.e., not displays) an ArrayList of Pair objects where the key of the pair is an Integer representing the number of downloads and the value is the Song object. The integer key should represent the number of times that the song was downloaded. The list returned should be sorted in decreasing order according to the number of times the song was downloaded. To sort a list of Pair objects, you can use the following code:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
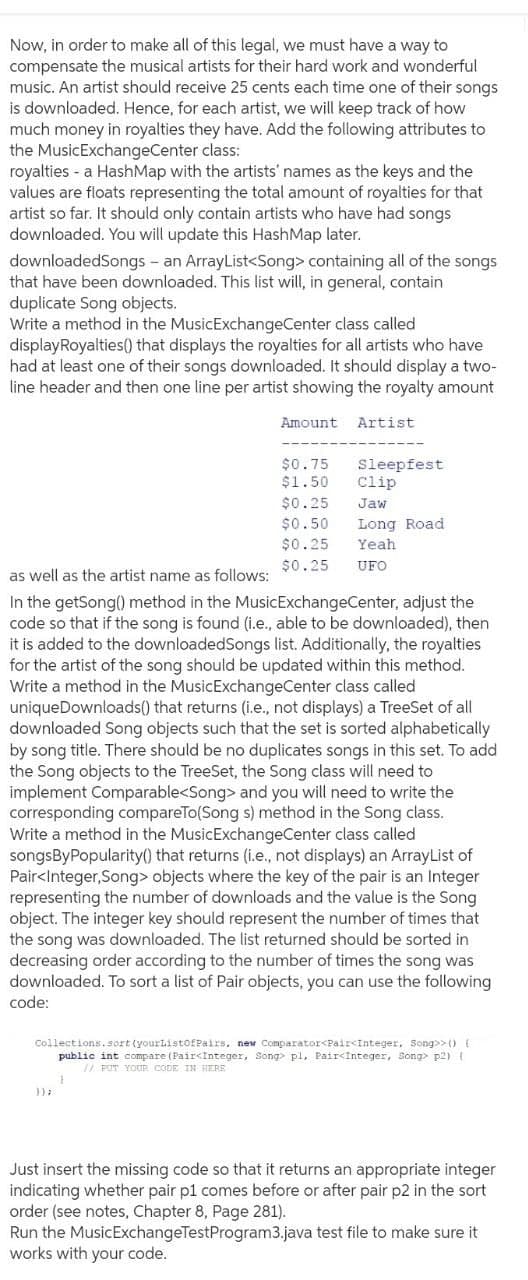
Transcribed Image Text:Now, in order to make all of this legal, we must have a way to
compensate the musical artists for their hard work and wonderful
music. An artist should receive 25 cents each time one of their songs
is downloaded. Hence, for each artist, we will keep track of how
much money in royalties they have. Add the following attributes to
the MusicExchangeCenter class:
royalties - a HashMap with the artists' names as the keys and the
values are floats representing the total amount of royalties for that
artist so far. It should only contain artists who have had songs
downloaded. You will update this HashMap later.
downloadedSongs - an ArrayList<Song> containing all of the songs
that have been downloaded. This list will, in general, contain
duplicate Song objects.
Write a method in the MusicExchangeCenter class called
displayRoyalties(0 that displays the royalties for all artists who have
had at least one of their songs downloaded. It should display a two-
line header and then one line per artist showing the royalty amount
Amount
Artist
$0.75
$1.50
Sleepfest
Clip
$0.25
Jaw
$0.50
Long Road
$0.25
Yeah
$0.25
UFO
as well as the artist name as follows:
In the getSong() method in the MusicExchangeCenter, adjust the
code so that if the song is found (i.e., able to be downloaded), then
it is added to the downloadedSongs list. Additionally, the royalties
for the artist of the song should be updated within this method.
Write a method in the MusicExchangeCenter class called
uniqueDownloads() that returns (i.e., not displays) a TreeSet of all
downloaded Song objects such that the set is sorted alphabetically
by song title. There should be no duplicates songs in this set. To add
the Song objects to the TreeSet, the Song class will need to
implement Comparable<Song> and you will need to write the
corresponding compareTo(Song s) method in the Song class.
Write a method in the MusicExchangeCenter class called
songsByPopularity() that returns (i.e., not displays) an ArrayList of
Pair<Integer,Song> objects where the key of the pair is an Integer
representing the number of downloads and the value is the Song
object. The integer key should represent the number of times that
the song was downloaded. The list returned should be sorted in
decreasing order according to the number of times the song was
downloaded. To sort a list of Pair objects, you can use the following
code:
Collections.sort (yourListofPairs, new Ccmparator<Pair<Integer, Song>>() {
public int compare (Pair<Integer, Song pl, Pair<Integer, Song> p2){
/ PUT YOUR CODE IN HERE
Just insert the missing code so that it returns an appropriate integer
indicating whether pair p1 comes before or after pair p2 in the sort
order (see notes, Chapter 8, Page 281).
Run the MusicExchangeTestProgram3.java test file to make sure it
works with your code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
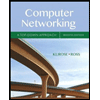
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
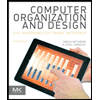
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
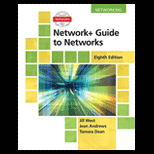
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
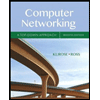
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
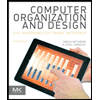
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
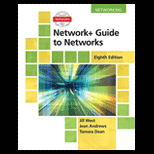
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
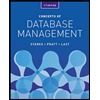
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
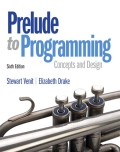
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
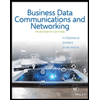
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY