Now write three methods as follows and place them above main:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
(Intro to Java)
- Open up Eclipse and create a new Java class called Salary.java.
- Copy and paste the starter code below into the file.
- NOTE: Do not change the starter code! All you need to do is add to it.
public class Salary {
}
//Write your methods here
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int hours;
double hourly_wage;
double weekly_salary = 0;
double monthly_salary = 0;
double yearly_salary = 0;
System.out.println("This program will calculate your weekly, monthly "
+ "and yearly salary!");
System.out.print("Please enter your hourly wage: ");
hourly_wage = input.nextDouble();
System.out.print("Please enter the number of hours you work each week: ");
hours = input.nextInt();
//call methods below
weekly_salary = //method call goes here
monthly_salary = //method call goes here
yearly_salary = //method call goes here
System.out.printf("You make $%.2f per week\n", weekly_salary);
System.out.printf("You make $%.2f per month\n", monthly_salary);
System.out.printf("You make $%.2f per year\n", yearly_salary);
input.close();
int hours;
double hourly_wage;
double weekly_salary = 0;
double monthly_salary = 0;
double yearly_salary = 0;
System.out.println("This program will calculate your weekly, monthly "
+ "and yearly salary!");
System.out.print("Please enter your hourly wage: ");
hourly_wage = input.nextDouble();
System.out.print("Please enter the number of hours you work each week: ");
hours = input.nextInt();
//call methods below
weekly_salary = //method call goes here
monthly_salary = //method call goes here
yearly_salary = //method call goes here
System.out.printf("You make $%.2f per week\n", weekly_salary);
System.out.printf("You make $%.2f per month\n", monthly_salary);
System.out.printf("You make $%.2f per year\n", yearly_salary);
input.close();
}
}
- Now write three methods as follows and place them above main:
weeklySalary():
- Takes an integer argument for the number of hours worked each week
- Takes a double argument for the hourly wage
- Returns a double for the weekly salary
monthlySalary():
- Takes an integer argument for the number of hours worked each week
- Takes a double argument for the hourly wage
- Calls the weeklySalary() method and multiplies the value it returns by 4 to calculate the monthly salary
- Returns a double for the monthly salary
yearlySalary():
- Takes in an integer argument for the number of hours worked each week
- Takes in a double argument for the hourly wage
- Calls the monthlySalary() method and multiplies the value it returns by 12 to calculate the yearly salary
- Returns a double for the yearly salary
- Assume that you work 12 months a year, without vacations
- After you write each method, add a method call and store the result as the appropriate variable.
weekly_salary = //method call goes here
monthly_salary = //method call goes here
yearly_salary = //method call goes here
monthly_salary = //method call goes here
yearly_salary = //method call goes here
- Now, run your code and verify that it prints the correct values for each salary type.
- When you are finished, upload your file
This program will calculate your weekly, monthly, and yearly salary!
Please enter your hourly wage: 15
Please enter the number of hours you work each week: 40
You make $600.00 per week.
You make $2400.00 per month.
You make $28800.00 per year.
Please enter your hourly wage: 15
Please enter the number of hours you work each week: 40
You make $600.00 per week.
You make $2400.00 per month.
You make $28800.00 per year.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
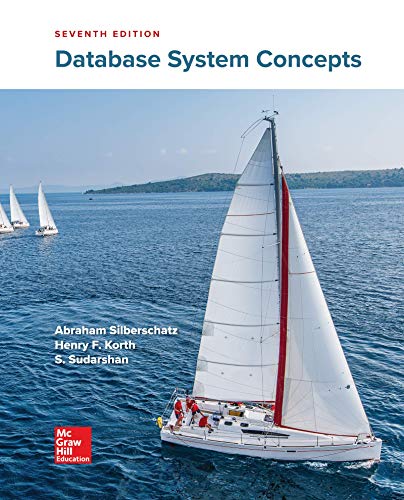
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
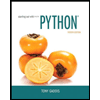
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
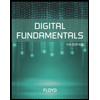
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
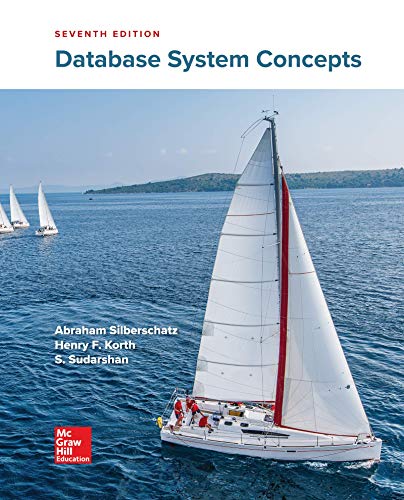
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
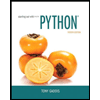
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
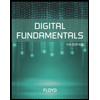
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
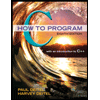
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
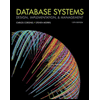
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
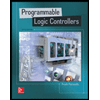
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education