Objective Create a class named Complex to represent complex numbers. Use the main() function and create at least three complex number objects and test all member functions of the Complex class. How to use classes. Create a program named LastnameFirstname19.cpp, that does the following: Note: There is no user input in this assignment. Copy and paste the Complex class definition from the previous assignment to this one. If you did not complete the previous assignment, you will need to create the class definition. Make any corrections to your Complex class based on the feedback provided from Assignment 18. Add the following member functions to the Complex class definition: Additional constructor with 2 parameters Signature: Complex(double realParam, double imaginaryParam) Allows a Complex object to be created with the specified real and imaginary parts. copy constructor Signature: Complex(const Complex ©Complex) Copies the data members from copyComplex to this object. destructor Signature: ~Complex() Output a message in the format: Destructor for: (a + bi) followed by a newline. If the imaginary part is negative (less than 0), print in the format Destructor for: (a - bi) followed by a newline. For example, if real is 6 and imaginary is -3: Destructor for: (6 - 3i)
- Objective
- Create a class named Complex to represent complex numbers. Use the main() function and create at least three complex number objects and test all member functions of the Complex class.
- How to use classes.
- Create a program named LastnameFirstname19.cpp, that does the following:
- Note: There is no user input in this assignment.
- Copy and paste the Complex class definition from the previous assignment to this one.
- If you did not complete the previous assignment, you will need to create the class definition.
- Make any corrections to your Complex class based on the feedback provided from Assignment 18.
- Add the following member functions to the Complex class definition:
- Additional constructor with 2 parameters
- Signature: Complex(double realParam, double imaginaryParam)
- Allows a Complex object to be created with the specified real and imaginary parts.
- copy constructor
- Signature: Complex(const Complex ©Complex)
- Copies the data members from copyComplex to this object.
- destructor
- Signature: ~Complex()
- Output a message in the format: Destructor for: (a + bi) followed by a newline.
- If the imaginary part is negative (less than 0), print in the format Destructor for: (a - bi) followed by a newline.
- For example, if real is 6 and imaginary is -3: Destructor for: (6 - 3i)
- See the example program destructor.cpp
- add member function
- Signature: Complex add(const Complex &addComplex) const
- Returns a new Complex object that is this object added by the specified object.
- Formula: (a + bi) + (c + di) = (a + c) + (b + d)i
- New Real: (a + c)
- New Imaginary: (b + d)
- subtract member function
- Signature: Complex subtract(const Complex &subtractComplex) const
- Returns a new Complex object that is this object subtracted by the specified object.
- Formula: (a + bi) - (c + di) = (a - c) + (b - d)i
- New Real: (a - c)
- New Imaginary: (b - d)
- multiply member function
- Signature: Complex multiply(const Complex &multiplyComplex) const
- Returns a new Complex object that is this object multiplied by the specified object.
- Formula: (a + bi) * (c + di) = (a*c - b*d) + (b*c + a*d)i
- New Real: (a*c - b*d)
- New Imaginary: (b*c + a*d)
- divide member function
- Signature: Complex divide(const Complex ÷Complex) const
- Returns a new Complex object that is this object divided by the specified object.
- Formula: (a + bi) / (c + di) = ((a*c + b*d)/(c*c + d*d)) + ((b*c - a*d)/(c*c + d*d))i
- New Real: ((a*c + b*d) / (c*c + d*d))
- New Imaginary: ((b*c - a*d) / (c*c + d*d))
- Be sure to have a program description at the top and in-line comments.
- Be clear with your comments and output to the user, so I can understand what the program is doing.
- Additional constructor with 2 parameters
Complex Class that should be adjusted
class Complex
{
private:
double real;
double imaginary;
public:
//structure of non parameterized constructor
Complex()
{
real = imaginary = 0.0;
}
void set(double r, double i)
{
real = r; //r initialized during object creation
imaginary = i; //i initialized during object creation
}
void print() const
{
//statement that adds real part of complex and adds imaginary parts of complex numbers.
if(imaginary < 0)
if(imaginary == -1)
cout << "(" << real << " - i" << ")" << endl;
else
cout << "("<< real << " - " << imaginary << "i" << ")" << endl;
else
if(imaginary == 1)
cout << "("<< real << " + i" << ")"<< endl;
else
cout << "("<< real << " + " << imaginary << "i" << ")" <<endl;
}
//return real
double getReal()
{
return real;
}
//return imaginary
double getImaginary()
{
return imaginary;
}
};
// main class
int main()
{
//printing of first complex statement
cout<<"Test the constructor"<<endl;
Complex c1;
cout<<"Complex number complex1 is: ";
c1.print();
//printing of second complex statement
cout<<"\nTest the one set() function."<<endl;
Complex c2;
c2.set(3.3, -4.4);
cout<<"Complex number complex2 is: ";
c2.print();
//printing of third complex statement
cout<<"\nTest the two get() function."<<endl;
Complex c3;
c3.set(5.5, 6.6);
cout<<"Complex number complex3's real part is: " << c3.getReal() << endl;
cout<<"Complex number complex3's imaginary part is: " << c3.getImaginary() << endl;
return 0;
}
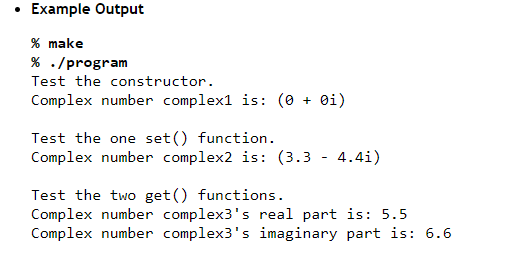

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

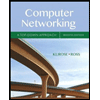
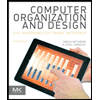
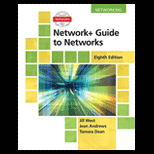
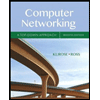
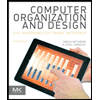
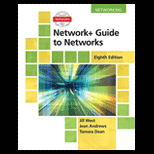
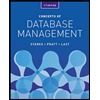
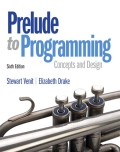
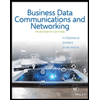