ogram and here are the instructions: In your program define a class called GeoPoint that will have the following: A constructor __init__(self, lat=0, lon=0,description=’TBD’) that will initialize the class variables called: self.lat, self.lon, and self.description. A SetPoint(self, point) method that takes an individual points coordinates as a single sequence and sets them to self.lat, self.long. E.g: self.lat = point[0]
I have to show a program that demonstrates a class using geo points. I have attached a previous program that I think can help with this. I don't know where to begin with inserting functions. I will attach a screenshot of my program and here are the instructions:
- In your program define a class called GeoPoint that will have the following:
- A constructor __init__(self, lat=0, lon=0,description=’TBD’) that will initialize the class variables called: self.lat, self.lon, and self.description.
- A SetPoint(self, point) method that takes an individual points coordinates as a single sequence and sets them to self.lat, self.long. E.g:
self.lat = point[0]
self.lon =point[1]
- A GetPoint(self) method that will return a tuple or list with self.lat, self.lon.
- A CalcDistance(self, lat, lon) method that will figure out the distance between the object’s self.lat, self.lon and lat, lon parameters passed in.
- A CalcDistancePoint(self, point) method that takes in a point (both coordinates at once, a data sequence, or GeoPoint object) that will figure out the distance between the object’s self.lat, self.lon and the lat and lon from the point.
- A SetDescription(self, description) method that will set the objects self.description attribute (variable).
- A GetDescription(self) method that will return the objects self.description attribute.
- Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for points.
- Add another property: Description = property(GetDescription, SetDescription). Make sure GetDescription and SetDescription are the names you used for the get and set methods you already wrote.
- In the main part of your program do the following:
- Include the class.
- Instantiate three points.
- Use the constructor to set point1 coordinates and description. It should look something like the following but with your own coordinates and description:
point1 = GeoPoint(12.3456,-123.4567,'Loc1')
- Use a constructor without any arguments to instantiate the second point and use its properties to set its values. It should look something like the following but with your own coordinates and description:
point2 = GeoPoint()
point2.Point = 23.4567, -213.456
point2.Description = 'Loc2'
- For point3 use the SetPoint and SetDescription methods to set the points location and description. Make sure they have different coordinates and different descriptions. It should look something like the following but with your own coordinates and description:
point3 = GeoPoint()
point3.SetPoint((25.25,52.52))
point3.SetDescription(“Loc3”)
- Inside a “Do another (y/n)?” loop do the following:
- Create a fourth point by asking the user for their location. You can ask for coordinates in three inputs or ask them for their coordinates in one input with each element separated by a coma.
- Use the CalcDistance method to find the distance between point1 and a new latitude and longitude of your choosing. Then tell the user the coordinates for point1, the new latitude and longitude that was entered, and the distance between those coordinates. For example:
distanceToPoint1 = point1.CalcDistance(lat, lon)
- Use point2 and point3’s CalcDistancePoint method to find the distance from each point to the user’s location. Notice we are passing in the users point rather than the separate coordinates:
distanceToTwo = point2.CalcDistancePoint(userPoint)
distanceToThree = point3.CalcDistancePoint(userPoint)
- Tell the user which point they are closest to in this format:
You are closest to <description> which is located at <point’s lat and lon coordinates>
- Ask “Do another (y/n)?” and loop if they respond with ‘y’


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

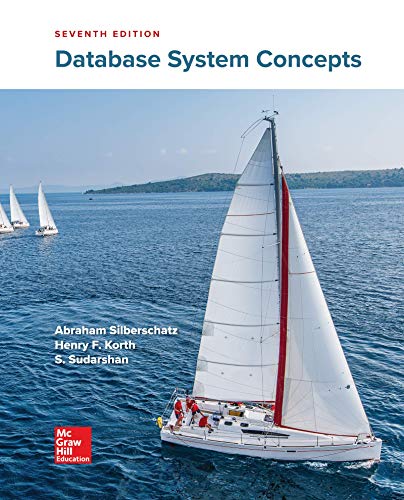
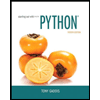
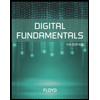
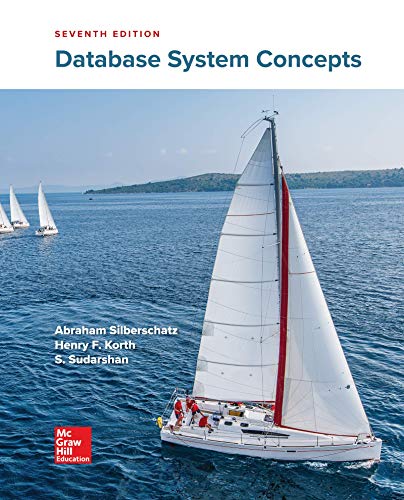
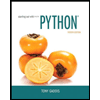
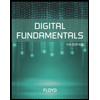
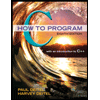
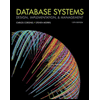
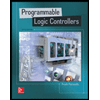