olocks of the Python programming language, that you have leamt so far can be put together to solve a given problem. The Superheroes Federation is an organization that brings together all kinds of superheroes to protect the Earth and Its Inhabitants, help fight dally crimes, as well as combat threats agalnst humanity from supervillains (their criminal counterparts) and make the Earth a better place to live. Some common tralts/characteristics of superheroes are: herolD is the unique ID number for the superhero (default value "N/A") alterEgo is the secondary self or secret identity of the superhero (eg. Batman) (default value "N/A") • knownFor is the special characteristic for which the hero is known (eg. can lift unusually heavy objects) (default value "N/A") numofchallengesMet is a number that indicates how many challenges have been faced and overcome by the superhero (default value o) Given the file, heroes.txt, where each line has the ID, alter ego, special characteristic and number of challenges met by the hero, write a Python program, In a file called supezhero_team.py. to do the following (you should Include the Superhero cass, given below, In the same file): Lin Read the Information from the file heroes.txt; Include necessary exception handling in case the file is not found.
olocks of the Python programming language, that you have leamt so far can be put together to solve a given problem. The Superheroes Federation is an organization that brings together all kinds of superheroes to protect the Earth and Its Inhabitants, help fight dally crimes, as well as combat threats agalnst humanity from supervillains (their criminal counterparts) and make the Earth a better place to live. Some common tralts/characteristics of superheroes are: herolD is the unique ID number for the superhero (default value "N/A") alterEgo is the secondary self or secret identity of the superhero (eg. Batman) (default value "N/A") • knownFor is the special characteristic for which the hero is known (eg. can lift unusually heavy objects) (default value "N/A") numofchallengesMet is a number that indicates how many challenges have been faced and overcome by the superhero (default value o) Given the file, heroes.txt, where each line has the ID, alter ego, special characteristic and number of challenges met by the hero, write a Python program, In a file called supezhero_team.py. to do the following (you should Include the Superhero cass, given below, In the same file): Lin Read the Information from the file heroes.txt; Include necessary exception handling in case the file is not found.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
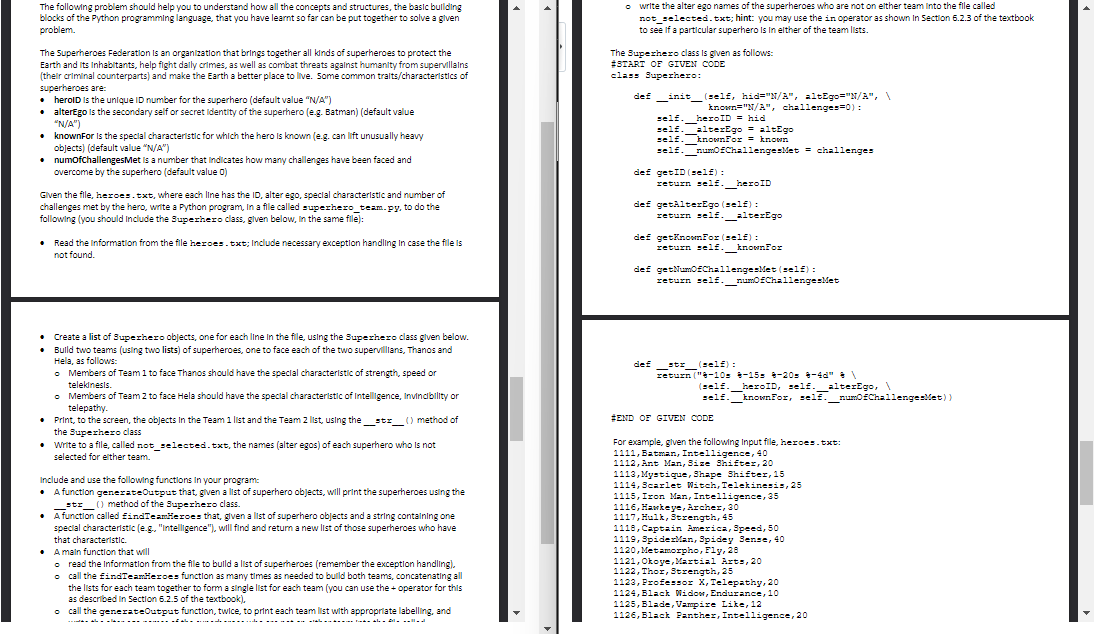
Transcribed Image Text:The following problem should help you to understand how all the concepts and structures, the basic bullding
blocks of the Python programming language, that you have learnt so far can be put together to solve a glven
problem.
• write the alter ego names of the superheroes who are not on elther team Into the file called
not_selected.txt; hint: you may use the in operator as shown In Section 6.2.3 of the textbook
to see if a particular superhero is in elther of the team lists.
The Superheroes Federation is an organization that brings together all kinds of superheroes to protect the
Earth and its Inhabitants, help fight dally crimes, as well as combat threats agalnst humanity from supervillains
(their criminal counterparts) and make the Earth a better place to live. Some common tralts/characteristics of
The Superhero class is given as follows:
#START OF GIVEN CODE
class Superhero:
superheroes are:
def _init_(self, hid="N/A", altEgo="N/A", \
known"N/A", challenges=0):
• herolD Is the unique ID number for the superhero (default value "N/A")
• alterēgo is the secondary self or secret identity of the superhero (e.g. Batman) (default value
"N/A")
• knownFor is the special characteristic for which the hero is known (e.g. can lift unusually heavy
objects) (default value "N/A")
• numofchallengesMet is a number that Indicates how many challenges have been faced and
overcome by the superhero (default value 0)
self._heroID - hid
self. alterEgo - altEgo
self. knownFor = known
self. numoEChallengesMet = challenges
def getID (self) :
return sel£._heroID
Given the file, heroes.txt, where each line has the ID, alter ego, special characteristic and number of
challenges met by the hero, write a Python program, In a file called superhero_team.py. to do the
following (you should Include the Superhero class, glven below, In the same file):
def getAlterEgo (self):
return self._alterEgo
• Read the Information from the file heroes.txt; Include necessary exception handling In case the file is
def getKnowmFor (self) :
return self. knownFor
not found.
def getNumOfChallengesMet (self) :
return self._numOEChallengesMet
• Create a list of Superhero objects, one for each line In the file, using the Superhero class glven below.
Bulld two teams (using two lists) of superheroes, one to face each of the two supervillians, Thanos and
Hela, as follows:
• Members of Team 1 to face Thanos should have the special characteristic of strength, speed or
def _str_(self):
return ("-10: t-15. +-20s -4d" :
telekinesis.
(self._heroID, self.
self. knownFor, self._numOEChallengesMet))
._alterEgo, \
• Members of Team 2 to face Hela should have the special characteristic of Intelligence, Invindbility on
telepathy.
• Print, to the screen, the objects In the Team 1 list and the Team 2 list, using thestr_() method of
the Superhero class
• Write to a file, called not_selected.txt, the names (alter egos) of each superhero who Is not
selected for either team.
#END OF GIVEN CODE
For example, glven the following Input file, heroes.txt:
1111, Batman, Intelligence, 40
1112, Ant Man, Size Shifter, 20
Include and use the following functions In your program:
• A function generateOutput that, given a list of superhero objects, will print the superheroes using the
_str_() method of the Superhero class.
• Afunction called findTeamieroes that, glven a list of superhero objects and a string contalning one
special characteristic (e.g. "Intelligence"), will find and return a new list of those superheroes who have
that characteristic.
• A maln functlon that will
• read the Information from the file to bulld a list of superheroes (remember the exception handling).
• call the findTeamHeroes function as many times as needed to bulld both teams, concatenating all
the lists for each team together to form a single list for each team (you can use the + operator for this
as described in Section 6.2.5 of the textbook).
• call the generateOutput function, twice, to print each team list with appropriate labelling, and
1113, Mystique, Shape Shifter, 15
1114, Scarlet Witch, Telekinesis, 25
1115, Iron Man, Intelligence, 35
1116, Hawkeye, Archer, 30
1117, Hulk, Serength, 45
1118, Captain America, Speed, 50
1119, SpidezMan, Spidey Sense, 40
1120, Metamorpho, Fly, 20
1121,Okoye, Martial Arts, 20
1122, Thor, Serength, 25
1123, Professor X, Telepathy, 20
1124, Black Widow, Endurance, 10
1125, Blade, Vampire Like, 12
1126, Black Panther, Intelligence, 20
- a- -l.-- .. a. d......d..... ...- .a...... a e. ...d
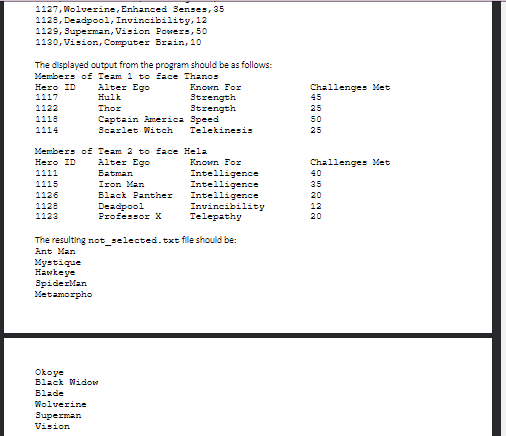
Transcribed Image Text:1127, Wolverine, Enhanced Senses, 35
1128, Deadpool, Invincibility, 12
1129, Superman, Vision Poorers, 50
1130, Vision, Computer Brain, 10
The displayed output from the program should be as follows:
Members of Team 1 to face Thanos
Hero ID
Alter Ego
Known For
Challenges Met
1117
Hulk
Strength
Strength
Captain America Speed
45
1122
1118
Thor
25
50
1114
Scarlet Witch
Telekinesis
25
Members of Team 2 to face Hela
Alter Ego
Hero ID
Enown For
Challenges Met
Intelligence
Intelligence
Intelligence
Invincibility
Telepathy
1111
Batman
40
1115
Iron Man
35
1126
Elack Panther
20
Deadpool
Professor X
1128
12
20
The resulting not_selected.txt file should be:
Ant Man
Mystique
Hawkeye
SpidezMan
Metamorpho
Okoye
Black Midow
Elade
Wolverine
Superman
Vision
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

Recommended textbooks for you
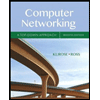
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
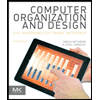
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
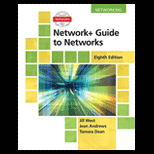
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
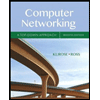
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
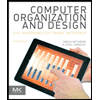
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
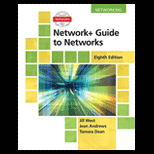
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
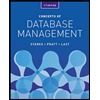
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
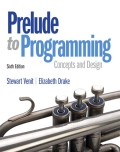
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
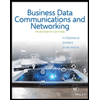
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY