Open the application in the folder under the coursework. Open the HTML file and notice that an Invoice Date field has been added. Then, open the HTML file and notice that it includes a getFormattedDate() function that formats the Date object that’s passed to it in MM/DD/YYYY format and then returns the date string. Start the application, enter a subtotal, and click the Calculate button. Note that nothing is displayed in the Invoice Date field. In the click() event handler for the Calculate button, add code that gets the current date and formats it using the getFormattedDate() function. Then, add code that provides a default value of the formatted current date for the invoice date. Note that if an invoice date is entered, that date isn’t validated. Add code that sets the value of the Invoice Date field. If you’ve done this right, the application should display the current date when you click the Calculate button without entering anything in the Invoice Date field.
Open the application in the folder under the coursework. Open the HTML file and notice that an Invoice Date field has been added. Then, open the HTML file and notice that it includes a getFormattedDate() function that formats the Date object that’s passed to it in MM/DD/YYYY format and then returns the date string. Start the application, enter a subtotal, and click the Calculate button. Note that nothing is displayed in the Invoice Date field. In the click() event handler for the Calculate button, add code that gets the current date and formats it using the getFormattedDate() function. Then, add code that provides a default value of the formatted current date for the invoice date. Note that if an invoice date is entered, that date isn’t validated. Add code that sets the value of the Invoice Date field. If you’ve done this right, the application should display the current date when you click the Calculate button without entering anything in the Invoice Date field.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
- Open the application in the folder under the coursework.
- Open the HTML file and notice that an Invoice Date field has been added. Then, open the HTML file and notice that it includes a getFormattedDate() function that formats the Date object that’s passed to it in MM/DD/YYYY format and then returns the date string.
- Start the application, enter a subtotal, and click the Calculate button. Note that nothing is displayed in the Invoice Date field.
- In the click() event handler for the Calculate button, add code that gets the current date and formats it using the getFormattedDate() function. Then, add code that provides a default value of the formatted current date for the invoice date. Note that if an invoice date is entered, that date isn’t validated.
- Add code that sets the value of the Invoice Date field. If you’ve done this right, the application should display the current date when you click the Calculate button without entering anything in the Invoice Date field.
<!DOCTYPE html>
<html lang="en">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Invoice Total Calculator</title>
<link rel="stylesheet" href="invoice.css">
</head>
<body>
<main>
<h1>Invoice Total Calculator</h1>
<p>Enter the two values that follow and click "Calculate".</p>
<div>
<label for="type">Customer Type:</label>
<select id="type">
<option value="reg">Regular</option>
<option value="loyal">Loyalty Program</option>
<option value="honored">Honored Citizen</option>
</select>
</div>
<div>
<label for="subtotal">Invoice Subtotal:</label>
<input type="text" id="subtotal">
</div>
<div>
<label for="invoice_date">Invoice Date:</label>
<input type="text" id="invoice_date">
</div>
<hr>
<div>
<label for="percent">Discount Percent:</label>
<input type="text" id="percent" disabled>%
</div>
<div>
<label for="discount">Discount Amount:</label>
<input type="text" id="discount" disabled>
</div>
<div>
<label for="total">Invoice Total:</label>
<input type="text" id="total" disabled><br>
</div>
<div>
<label> </label>
<input type="button" id="calculate" value="Calculate">
</div>
</main>
<script src="https://code.jquery.com/jquery-3.4.1.slim.min.js"></script>
<script src="invoice.js"></script>
</body>
</html>
body {
font-family: Arial, Helvetica, sans-serif;
background-color: white;
margin: 0 auto;
width: 500px;
border: 3px solid blue;
padding: 0 2em 1em;
}
h1 {
color: blue;
}
div, hr {
margin-bottom: 1em;
}
label {
display: inline-block;
width: 8em;
}
input, select {
margin-left: 1em;
margin-right: 0.5em;
}
span {
color: red;
}
"use strict";
const calculateDiscount = (customer, subtotal) => {
switch(customer) {
case "reg":
if (subtotal >= 100 && subtotal < 250) {
return .1;
} else if (subtotal >= 250 && subtotal < 500) {
return .25;
} else if (subtotal >= 500) {
return .3;
} else {
return 0;
}
case "loyal":
return .3;
case "honored":
return (subtotal < 500) ? .4 : .5;
}
};
const getFormattedDate = date => {
const month = date.getMonth() + 1;
const day = date.getDate();
const year = date.getFullYear();
const dateText = month + "/" + day + "/" + year;
return dateText;
}
$( document ).ready( () => {
$("#calculate").click( () => {
const customerType = $("#type").val();
let subtotal = $("#subtotal").val() || 0; // default value of zero
subtotal = parseFloat(subtotal);
const discountPercent = calculateDiscount(customerType, subtotal);
const discountAmount = subtotal * discountPercent;
const invoiceTotal = subtotal - discountAmount;
$("#subtotal").val( subtotal.toFixed(2) );
$("#percent").val( (discountPercent * 100).toFixed(2) );
$("#discount").val( discountAmount.toFixed(2) );
$("#total").val( invoiceTotal.toFixed(2) );
// set focus on type drop-down when done
$("#type").focus();
});
// set focus on type drop-down on initial load
$("#type").focus();
});
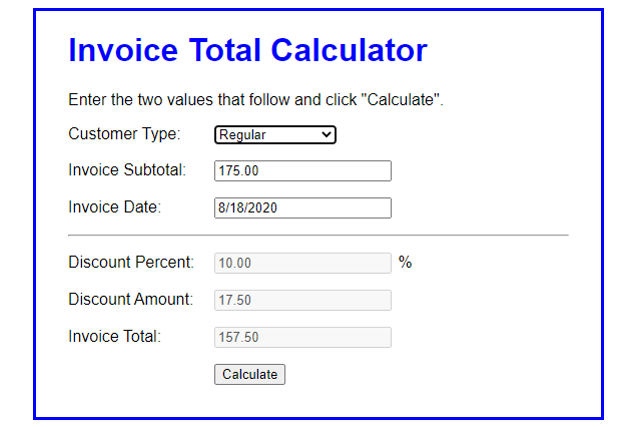
Transcribed Image Text:Invoice Total Calculator
Enter the two values that follow and click "Calculate".
Customer Type: Regular
Invoice Subtotal:
175.00
Invoice Date:
8/18/2020
Discount Percent: 10.00
%
Discount Amount:
17.50
Invoice Total:
157.50
Calculate
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
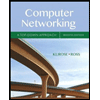
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
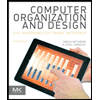
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
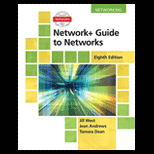
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
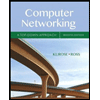
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
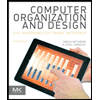
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
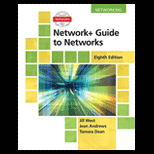
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
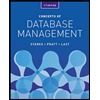
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
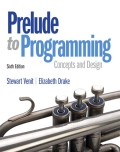
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
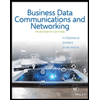
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY