or faster sorting of letters, the United States Postal Service encourages companies that send large volumes of mail to use a bar code denoting the zip code: . The encoding scheme for a five-digit zip code is shown below. • There are full-height frame bars on each side. • The five encoded digits are followed by a check digit, which is computed by add- ing up all digits, and choose a check digit to make the sum a multiple of 10. Digit 1 2 . • For example, the number 95014 has a sum of 19, so the check digit is 1 to make the sum equal to 20. 3 4 5 6 *************** ECRLOT** CO57 Each digit of the zip code, and the check digit, is encoded according to the following table where O denotes a half bar and 1 a full bar. 7 8 9 0 CODE C671RTS2 JOHN DOE 1009 FRANKLIN BLVD SUNNYVALE Hleblebell.llekuleleballadkakalkdald 1 I ll ...lll Digit 1 Digit 2 Digit 3 Digit 4 Digit 5 Check Digit Encoding for Five-Digit Bar Codes 0 0 0 0 1 1 CA 95014-5143 1 Frame bars Bar 2 Bar 3 Bar 4 Bar 5 Bar 1 (weight 7) (weight 4) (weight 2) (weight 1) (weight 0) 0 0 0 1 1 0 0 1 0 0 1 1 1 1 0 0 0 1 CO57 1 0 0 1 0 0 1 0 0 1 0 0 1 0 A Few Hints • codeForDigit() works great with just a switch. 0 1 0 1 0 To solve this problem, write three functions (pay attention to the return types): string codeForDigit(int digit) which takes one digit and returns the ap- propriate code for that digit, using the table above. Use: for the half-bars and 'I' for the full bars. 0 1 0 0 0 int checkDigit(int zip) which calculates the check digit according to the rules shown above. • string barCode(int zip) which returns an entire bar code by breaking the number into individual digits, encoding that digit, and adding it to the string re- turn value. Finally, calculate and encode the check digit, surround the entire code with the frame bars, and return it. Here are the steps to solve this problem: 1. Write and document the prototypes the header file. Add header guards. 2. Stub out your functions in the implementation file. Run make test to make sure you have the mechanics correct before going on. 3. Implement the codeForDigit() function first. Run the tests for that. 4. Calculate the checkDigit() function and run the tests for that. 5. Finally, write and test the barCode() function (which will be fairly short). • For checkDigit(), use a limit loop as well as the remainder operator to find the digit that gets the sum to the next multiple of 10. • For barCode(), first calculate the check digit. Extract each digit using a limit loop and get its code; use the building-a-string pattern to add the code to the result. Finally, add the code for the check digit and the two frame bars. Use make test to test your code or make run to run any student tests. Once your score is OK, use make submit to turn it in.
or faster sorting of letters, the United States Postal Service encourages companies that send large volumes of mail to use a bar code denoting the zip code: . The encoding scheme for a five-digit zip code is shown below. • There are full-height frame bars on each side. • The five encoded digits are followed by a check digit, which is computed by add- ing up all digits, and choose a check digit to make the sum a multiple of 10. Digit 1 2 . • For example, the number 95014 has a sum of 19, so the check digit is 1 to make the sum equal to 20. 3 4 5 6 *************** ECRLOT** CO57 Each digit of the zip code, and the check digit, is encoded according to the following table where O denotes a half bar and 1 a full bar. 7 8 9 0 CODE C671RTS2 JOHN DOE 1009 FRANKLIN BLVD SUNNYVALE Hleblebell.llekuleleballadkakalkdald 1 I ll ...lll Digit 1 Digit 2 Digit 3 Digit 4 Digit 5 Check Digit Encoding for Five-Digit Bar Codes 0 0 0 0 1 1 CA 95014-5143 1 Frame bars Bar 2 Bar 3 Bar 4 Bar 5 Bar 1 (weight 7) (weight 4) (weight 2) (weight 1) (weight 0) 0 0 0 1 1 0 0 1 0 0 1 1 1 1 0 0 0 1 CO57 1 0 0 1 0 0 1 0 0 1 0 0 1 0 A Few Hints • codeForDigit() works great with just a switch. 0 1 0 1 0 To solve this problem, write three functions (pay attention to the return types): string codeForDigit(int digit) which takes one digit and returns the ap- propriate code for that digit, using the table above. Use: for the half-bars and 'I' for the full bars. 0 1 0 0 0 int checkDigit(int zip) which calculates the check digit according to the rules shown above. • string barCode(int zip) which returns an entire bar code by breaking the number into individual digits, encoding that digit, and adding it to the string re- turn value. Finally, calculate and encode the check digit, surround the entire code with the frame bars, and return it. Here are the steps to solve this problem: 1. Write and document the prototypes the header file. Add header guards. 2. Stub out your functions in the implementation file. Run make test to make sure you have the mechanics correct before going on. 3. Implement the codeForDigit() function first. Run the tests for that. 4. Calculate the checkDigit() function and run the tests for that. 5. Finally, write and test the barCode() function (which will be fairly short). • For checkDigit(), use a limit loop as well as the remainder operator to find the digit that gets the sum to the next multiple of 10. • For barCode(), first calculate the check digit. Extract each digit using a limit loop and get its code; use the building-a-string pattern to add the code to the result. Finally, add the code for the check digit and the two frame bars. Use make test to test your code or make run to run any student tests. Once your score is OK, use make submit to turn it in.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In c++ please! I've included the header and makefile.
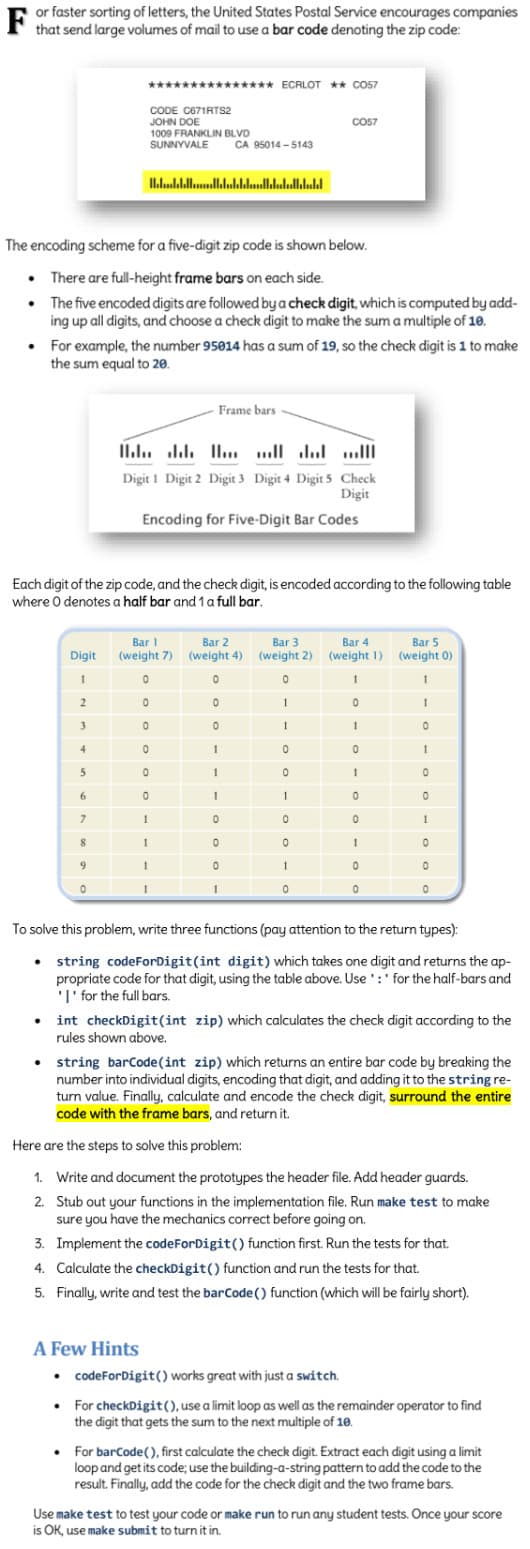
Transcribed Image Text:F
or faster sorting of letters, the United States Postal Service encourages companies
that send large volumes of mail to use a bar code denoting the zip code:
●
The encoding scheme for a five-digit zip code is shown below.
•
There are full-height frame bars on each side.
The five encoded digits are followed by a check digit, which is computed by add-
ing up all digits, and choose a check digit to make the sum a multiple of 10.
Digit
1
2
3
.
4
5
*************** ECRLOT ** CO57
CODE C671RTS2
JOHN DOE
1009 FRANKLIN BLVD
SUNNYVALE
For example, the number 95014 has a sum of 19, so the check digit is 1 to make
the sum equal to 20.
6
Each digit of the zip code, and the check digit, is encoded according to the following table
where 0 denotes a half bar and 1 a full bar.
7
8
9
0
Hllddell...lldalled.ll.lalalklald
CA 95014-5143
1
Frame bars.
Hlllllll
Digit 1 Digit 2 Digit 3 Digit 4 Digit 5 Check
Digit
Encoding for Five-Digit Bar Codes
1
Bar 1
Bar 3
Bar 4
Bar 5
Bar 2
(weight 7) (weight 4) (weight 2) (weight 1) (weight 0)
0
0
0
1
1
0
1
0
0
0
0
0
1
1
CO57
0
0
1
1
1
0
0
0
1
1
0
0
1
0
0
1
0
1
0
1
0
0
1
0
0
1
0
1
0
0
1
To solve this problem, write three functions (pay attention to the return types):
• string codeForDigit(int digit) which takes one digit and returns the ap-
propriate code for that digit, using the table above. Use: for the half-bars and
'I' for the full bars.
0
0
0
int checkDigit(int zip) which calculates the check digit according to the
rules shown above.
string barCode(int zip) which returns an entire bar code by breaking the
number into individual digits, encoding that digit, and adding it to the string re-
turn value. Finally, calculate and encode the check digit, surround the entire
code with the frame bars, and return it.
Here are the steps to solve this problem:
1. Write and document the prototypes the header file. Add header guards.
2. Stub out your functions in the implementation file. Run make test to make
sure you have the mechanics correct before going on.
3. Implement the codeForDigit() function first. Run the tests for that.
4. Calculate the checkDigit() function and run the tests for that.
5. Finally, write and test the barCode() function (which will be fairly short).
A Few Hints
• codeForDigit() works great with just a switch.
• For checkDigit(), use a limit loop as well as the remainder operator to find
the digit that gets the sum to the next multiple of 10.
• For barCode(), first calculate the check digit. Extract each digit using a limit
loop and get its code; use the building-a-string pattern to add the code to the
result. Finally, add the code for the check digit and the two frame bars.
Use make test to test your code or make run to run any student tests. Once your score
is OK, use make submit to turn it in.
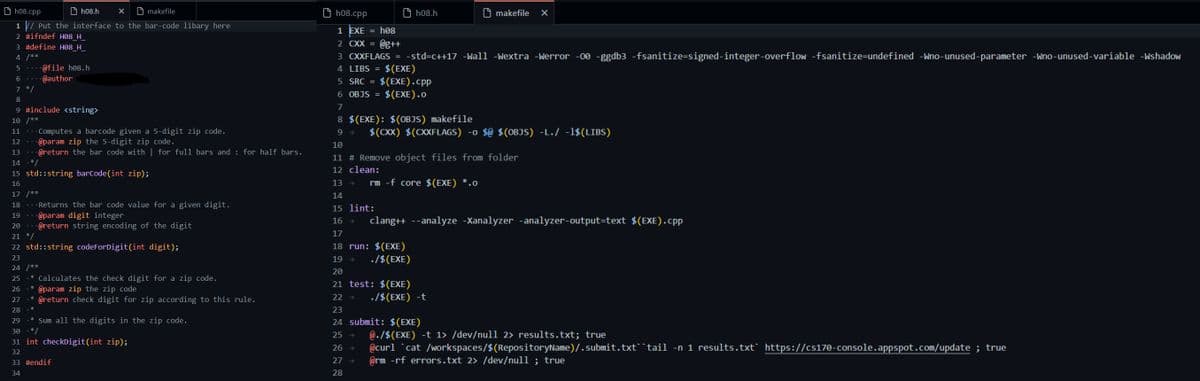
Transcribed Image Text:h08.cpp
h08.h x
makefile
1 /// Put the interface to the bar-code libary here
2 #ifndef H08_H_
3 #define H08_H_
4 /**
5 ... @file h08.h
6 ....@author
7 */
8
9 #include <string>
10 /**
11 ...Computes a barcode given a 5-digit zip code.
12
@param zip the 5-digit zip code.
13 ...@return the bar code with | for full bars and for half bars.
14 .*/
15 std::string barCode(int zip);
16
17 /**
18
19 @param digit integer
20 @return string encoding of the digit
21 */
22 std::string codeForDigit(int digit);
23
24 /**
25 .* Calculates the check digit for a zip code.
26 .* @param zip the zip code
27 .* @return check digit for zip according to this rule.
28
29 .* Sum all the digits in the zip code.
30
31 int checkDigit(int zip);
32
33 #endif
34
eturr the bar code value for a given digit.
h08.cpp
1 EXE h08
2 CXX =
@g++
3 CXXFLAGS = -std=c++17 -Wall -Wextra -Werror -00 -ggdb3 -fsanitize=signed-integer-overflow -fsanitize=undefined -Wno-unused-parameter -Wno-unused-variable -Wshadow
4 LIBS = $(EXE)
h08.h
5 SRC = $(EXE).cpp
6 OBJS = $(EXE).O
7
8 $(EXE): $(OBJS) makefile
9 → $(CXX) $(CXXFLAGS) -o $@ $(OBJS) -L./ -1$(LIBS)
10
11 # Remove object files from folder
12 clean:
13 → rm -f core $(EXE) *.0
20
21 test: $(EXE)
22 →
makefile X
14
15 lint:
16 → clang++ --analyze -Xanalyzer -analyzer-output=text $(EXE).cpp
17
18 run: $(EXE)
19 → ./$(EXE)
./$(EXE) -t
27
28
23
24 submit: $(EXE)
25 @./$(EXE) -t 1> /dev/null 2› results.txt; true
26 → @curl cat /workspaces/$(RepositoryName)/.submit.txt``tail -n 1 results.txt` https://cs170-console.appspot.com/update ; true
@rm -rf errors.txt 2> /dev/null ; true
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
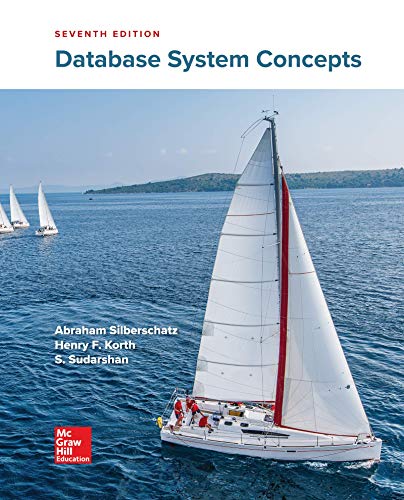
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
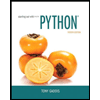
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
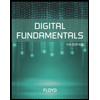
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
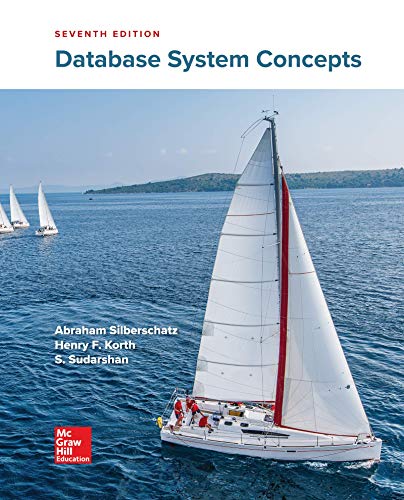
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
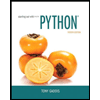
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
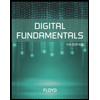
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
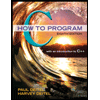
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
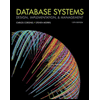
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
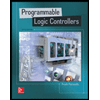
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education