osts are Store onstant) lists: ITEMS = ["Beans", "Rice", "Banana", "Ice", "Tea", "Bread", "Orange", "Sugar"] PRICES = [3.25, 4.31, 6.88, 3.3, 5.25, 4.89, 6.32, 2.25] should implement the following functions to print a cost table. make_cost_table). Called by the main() function, this function will prompt the user for the maximum quantity (between 1 an orientation of the table (item\qty as horizontal). It will handle the user input error. Once valid values are obtained from the us next function to build the string that contains the formatted cost table, and return it. build_cost_table(items, prices, max_qty, horizontal). This function will build and return a string that represents the cost table cost of buying that item for a quantity from 1 to the max_qty. If horizontal is True, each row is for one item and each column otherwise, each row will be for each quantity and each column is for each item. You must use nested for loops to build the c
osts are Store onstant) lists: ITEMS = ["Beans", "Rice", "Banana", "Ice", "Tea", "Bread", "Orange", "Sugar"] PRICES = [3.25, 4.31, 6.88, 3.3, 5.25, 4.89, 6.32, 2.25] should implement the following functions to print a cost table. make_cost_table). Called by the main() function, this function will prompt the user for the maximum quantity (between 1 an orientation of the table (item\qty as horizontal). It will handle the user input error. Once valid values are obtained from the us next function to build the string that contains the formatted cost table, and return it. build_cost_table(items, prices, max_qty, horizontal). This function will build and return a string that represents the cost table cost of buying that item for a quantity from 1 to the max_qty. If horizontal is True, each row is for one item and each column otherwise, each row will be for each quantity and each column is for each item. You must use nested for loops to build the c
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
![Cost Table
The items and costs are stored in two (constant) lists: ITEMS and PRICES.
ITEMS = ["Beans", "Rice", "Banana", "Ice", "Tea", "Bread", "Orange", "Sugar"]
PRICES = [3.25, 4.31, 6.88, 3.3, 5.25, 4.89, 6.32, 2.25]
You should implement the following functions to print a cost table.
• make_cost_table(). Called by the main() function, this function will prompt the user for the maximum quantity (between 1 and 9 inclusive), and the
orientation of the table (item\gty as horizontal). It will handle the user input error. Once valid values are obtained from the user, this function will call the
next function to build the string that contains the formatted cost table, and return it.
• build_cost_table(items, prices, max_gty, horizontal). This function will build and return a string that represents the cost table by listing for each item the
cost of buying that item for a quantity from 1 to the max_gty. If horizontal is True, each row is for one item and each column is for each quantity,
otherwise, each row will be for each quantity and each column is for each item. You must use nested for loops to build the cost table. For each row
and for each column in the row, use string format (use'..'%(...) method) to concatenate the cost to the tabler. For each entity (string, int, or float) set
the field to 8 (therefore, %8s, %8d, %8.2f). For example,
build_cost_table(ITEMS, PRICES, 5, True)
should return the following string.
Cost Table
Item\Qty
1
2
3
4
Вeans
3.25
6.50
9.75
13.00
16.25
Rice
4.31
8.62
12.93
17.24
21.55
Banana
6.88
13.76
20.64
27.52
34.40
Ice
3.30
6.60
9.90
13.20
16.50
Тea
5.25
10.50
15.75
21.00
26.25
Bread
4.89
9.78
14.67
19.56
24.45
Orange
6.32
12.64
18.96
25.28
31.60
Sugar
2.25
4.50
6.75
9.00
11.25
and
build_cost_table(ITEMS, PRICES, 5, False)
should return the following string.
Cost Tahle](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1bc6a0e5-2ee6-4288-975e-2cc10c81a0cb%2F9b8002f5-268e-4a30-8a08-6ea6bb8765da%2Ft7jjgfk_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Cost Table
The items and costs are stored in two (constant) lists: ITEMS and PRICES.
ITEMS = ["Beans", "Rice", "Banana", "Ice", "Tea", "Bread", "Orange", "Sugar"]
PRICES = [3.25, 4.31, 6.88, 3.3, 5.25, 4.89, 6.32, 2.25]
You should implement the following functions to print a cost table.
• make_cost_table(). Called by the main() function, this function will prompt the user for the maximum quantity (between 1 and 9 inclusive), and the
orientation of the table (item\gty as horizontal). It will handle the user input error. Once valid values are obtained from the user, this function will call the
next function to build the string that contains the formatted cost table, and return it.
• build_cost_table(items, prices, max_gty, horizontal). This function will build and return a string that represents the cost table by listing for each item the
cost of buying that item for a quantity from 1 to the max_gty. If horizontal is True, each row is for one item and each column is for each quantity,
otherwise, each row will be for each quantity and each column is for each item. You must use nested for loops to build the cost table. For each row
and for each column in the row, use string format (use'..'%(...) method) to concatenate the cost to the tabler. For each entity (string, int, or float) set
the field to 8 (therefore, %8s, %8d, %8.2f). For example,
build_cost_table(ITEMS, PRICES, 5, True)
should return the following string.
Cost Table
Item\Qty
1
2
3
4
Вeans
3.25
6.50
9.75
13.00
16.25
Rice
4.31
8.62
12.93
17.24
21.55
Banana
6.88
13.76
20.64
27.52
34.40
Ice
3.30
6.60
9.90
13.20
16.50
Тea
5.25
10.50
15.75
21.00
26.25
Bread
4.89
9.78
14.67
19.56
24.45
Orange
6.32
12.64
18.96
25.28
31.60
Sugar
2.25
4.50
6.75
9.00
11.25
and
build_cost_table(ITEMS, PRICES, 5, False)
should return the following string.
Cost Tahle
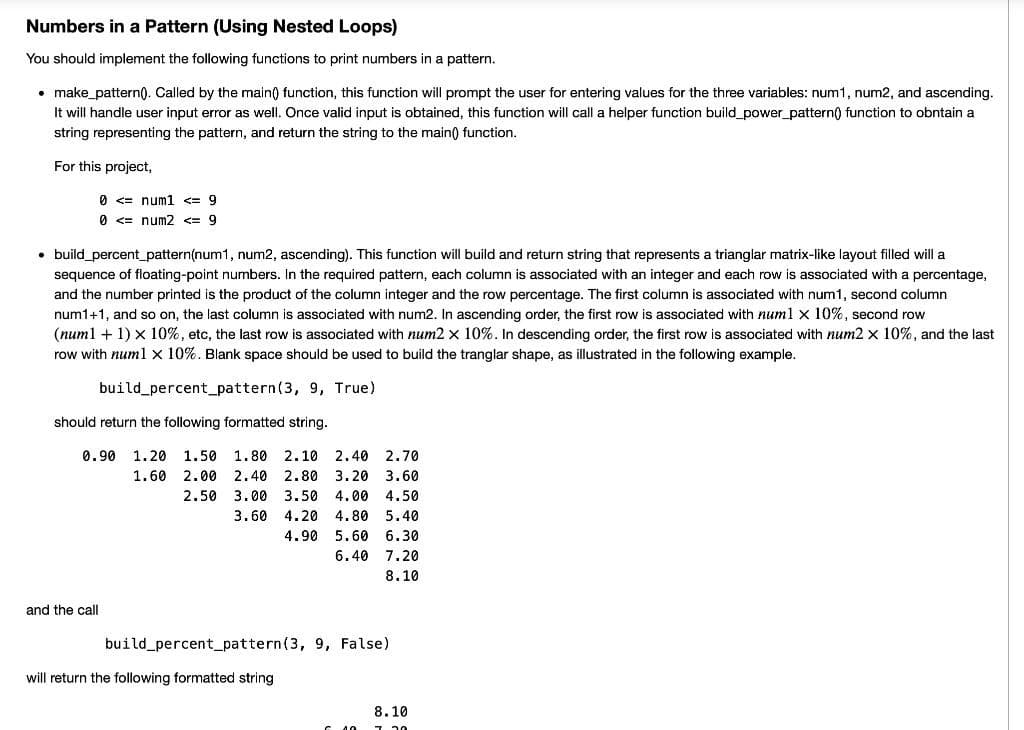
Transcribed Image Text:Numbers in a Pattern (Using Nested Loops)
You should implement the following functions to print numbers in a pattern.
• make_pattern). Called by the main) function, this function will prompt the user for entering values for the three variables: num1, num2, and ascending.
It will handle user input error as well. Once valid input is obtained, this function will call a helper function build_power_pattern() function to obntain a
string representing the pattern, and return the string to the main) function.
For this project,
0 <= num1 <= 9
0 <= num2 <= 9
• build_percent_pattern(num1, num2, ascending). This function will build and return string that represents a trianglar matrix-like layout filled will a
sequence of floating-point numbers. In the required pattern, each column is associated with an integer and each row is associated with a percentage,
and the number printed is the product of the column integer and the row percentage. The first column is associated with num1, second column
num1+1, and so on, the last column is associated with num2. In ascending order, the first row is associated with numl x 10%, second row
(num1 + 1) x 10%, etc, the last row is associated with num2 x 10%. In descending order, the first row is associated with num2 x 10%, and the last
row with num1 x 10%. Blank space should be used to build the tranglar shape, as illustrated in the following example.
build percent_pattern(3, 9, True)
should return the following formatted string.
0.90
1.20
1.50 1.80 2.10 2.40 2.70
1.60
2.00 2,40
2.80
3.20
3.60
2.50 3.00
3.50
4.00
4.50
3.60
4.20
4.80
5.40
4.90
5.60 6.30
6.40
7.20
8.10
and the call
build percent_pattern(3, 9, False)
will return the following formatted string
8.10
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
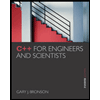
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
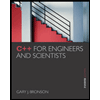
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr