Output is nearly correct, but whitespace differs. See highlights below. Special character legend Your output + $10.95 is $16.40 - $10.95 is null 5 $5.45 is $5.50 3 method result: false reTo method result: -550 ted list $4.90 $17.56 $8.88 $22.14 $14.99 $22.08 $16.80 $17.53 $14.38 9 $16.08 $6.49 $15.70 $9.50 ax is $24.09 list $4.90 $6.49 $8.88 $9.50 $14.38 $14.99 $15.70 $16.08 $16.80 3 $17.56 $22.08 $22.14 $24.09 45 $10.95 is $16.40 45 $10.95 is null .95 $5.45 is $5.50 als method result: false pareTo method result: -550 brted list Expected output 30 $4.90 $17.56 $8.88 $22.14 $14.99 $22.08 $16.80 $17.53 $14.38 09 $16.08 $6.49 $15.70 $9.50 Max is $24.09 ted list 30 $4.90 $6.49 $8.88 $9.50 $14.38 $14.99 $15.70 $16.08 $16.80 .53 $17.56 $22.08 $22.14 $24.09
import java.util.*;
class Money {
private int dollar;
private int cent;
final static int MIN_CENT_VALUE = 0;
final static int MAX_CENT_VALUE = 99;
public Money() {
this.dollar = 0;
this.cent = 0;
}
public Money(int dollar, int cent) {
this.dollar = dollar;
if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) {
this.cent = cent;
} else {
cent = 0;
}
}
public int getDollar() {
return this.dollar;
}
public void setDollar(int dol) {
this.dollar = dol;
}
public int getCent() {
return this.cent;
}
public void setCent(int c) {
if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) {
this.cent = c;
}
}
public Money add(Money otherMoney) {
Money m = new Money();
m.dollar = this.dollar + otherMoney.dollar;
m.cent = this.cent + otherMoney.cent;
if (m.cent >= 100) {
m.dollar++;
m.cent -= 100;
}
return m;
}
public Money subtract(Money otherMoney) {
Money m = new Money();
m.dollar = this.dollar - otherMoney.dollar;
m.cent = this.cent - otherMoney.cent;
if (m.dollar < 0 || m.cent < 0) {
return null;
}
return m;
}
public boolean equals(Money otherMoney) {
return this == otherMoney;
}
public int compareTo(Money otherMoney) {
Money m = new Money();
if (this.dollar < otherMoney.dollar) {
m = otherMoney.subtract(this);
return -(m.dollar * 100 + m.cent);
} else if (this.dollar > otherMoney.dollar) {
m = this.subtract(otherMoney);
return m.dollar * 100 + m.cent;
} else {
return 0;
}
}
public String toString() {
if (this.cent < 10) {
return "$" + this.dollar + ".0" + this.cent;
}
return "$" + this.dollar + "." + this.cent;
}
public static Money max(Money[] m) {
int max = (m[0].dollar*100) + m[0].cent;
int index = 0;
for (int i = 0; i < m.length; i++) {
if (max < (m[i].dollar*100) + m[i].cent) {
max = (m[i].dollar*100) + m[i].cent;
index = i;
}
}
return m[index];
}
public static void selectionSort(Money arr[]) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
int min = i;
for (int j = i + 1; j < n; j++)
if (arr[j].dollar + ((arr[j].cent) / 100.0) < arr[min].dollar + ((arr[min].cent) / 100.0))
min = j;
Money temp = new Money();
temp = arr[min];
arr[min] = arr[i];
arr[i] = temp;
}
}
public static void printList(Money[] m, int i)
{
int count=0;
for (Money mm : m) {
System.out.print(mm +" ");
count++;
if(count%10==0){
System.out.println();
}
}
System.out.println();
}
}
can you change the following code to follow the formatting requirements stated here
I don't know what to do
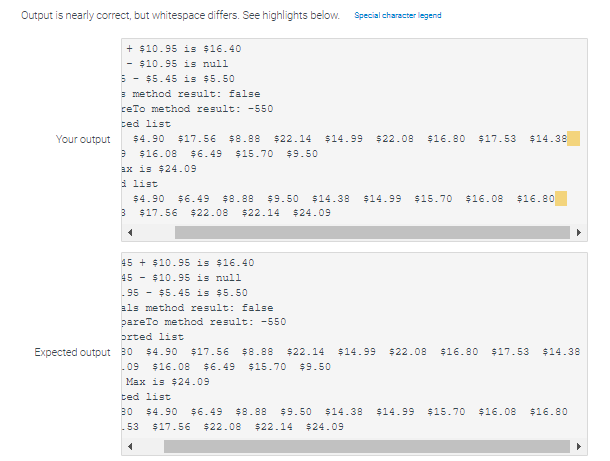

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

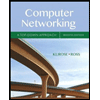
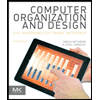
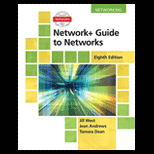
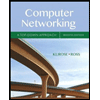
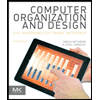
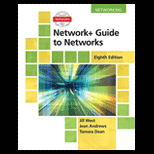
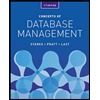
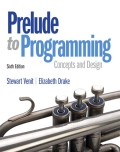
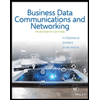