ow to do all of this in java following the diagram in image 1 using these rules
ow to do all of this in java following the diagram in image 1 using these rules
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 19RQ
Related questions
Question
how to do all of this in java following the diagram in image 1 using these rules
![For this task you will create a series of classes/interfaces that will implement 1-D and
2- automata (check out this link for information on 1-D automata as well as this one for 2-
D). The classes/interfaces that we will create are shown in UML diagram below:
Cell
Life
}
Board
automata
<<interface>>
drawable
We have the abstract class Board which is made up of Cells, with both Cells and Board
implementing the drawable interface. The concrete classes, Life and automata, implement 2D
and 1D automata, respectively.
<<enumeration>>
state
The Drawable interface is shown below. Put it in its own class file called Drawable.java
public interface Drawable {
void Draw();
public enum state {DEAD, ALIVE};
The enumeration class state is a special Java class that lets you create a new type, state, with
very specific values. In our case, these values are DEAD and ALIVE. Here is the code for this
class.
This line must be inserted in a file called state.java. You will use this type when keeping track of
the the state of a cell. Here is a bit a code showing how you might use it.
states;
int x = 0;
if(0 == x)
else s
Cell
-s: state
-alive :char
-dead : char
Lets start with the Cell class. Here is the the UML class diagram for it:
s = state. DEAD; //access values via type name
state.ALIVE;
+Cell(s:state)
+Cell(s:state, alive :char, dead :char)
+getState(): state
+setState(s:state) : void
+Draw(): void
Next we have the abstract class Board
Board
#curr : Cell[][]
#alive :char
#dead : char
// you can create a variable
// of the enumurated type
+numLiveCell((i :int, j : int): int
+nextGen():void
The state of the cell
Character to draw on screen if cell is alive (default='#')
Character to draw on screen if cell is dead (default='')
Constructs a cell with the given state and default chars
Constructs a cell with the given state and characters
Returns state of cell
Sets state of cell.
Draw method implementation of Drawable interface
A 2-D array used to represent the board (or row)
Character to draw on screen if cell is alive (default='#')
Character to draw on screen if cell is dead (default='')
+Board(r:int; c:int)
Creates array curr with r rows and c columns
+Board(r:int, c:int, alive :char, dead :char) Like above but also changes printing characters
+setBoard (s:state) : void
Creates and sets the state of ALL cells in curr to s.
+setCell(i :int, j : int, c : Cell): void
+getCell(i :int, j : int) :Cell
+Draw(): void
Changes reference at curr[i][j] to c
Returns reference to cell found at curr[i][j]
Draw method implementation of Drawable interface
How many live cells exist around cell at curr[i][j]
Computes new generation based on old](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F66301e59-a86d-47c0-9dd8-21e24614422a%2F95fb4598-1745-4b83-84f1-bd40d48d2d1f%2F9l8959x_processed.png&w=3840&q=75)
Transcribed Image Text:For this task you will create a series of classes/interfaces that will implement 1-D and
2- automata (check out this link for information on 1-D automata as well as this one for 2-
D). The classes/interfaces that we will create are shown in UML diagram below:
Cell
Life
}
Board
automata
<<interface>>
drawable
We have the abstract class Board which is made up of Cells, with both Cells and Board
implementing the drawable interface. The concrete classes, Life and automata, implement 2D
and 1D automata, respectively.
<<enumeration>>
state
The Drawable interface is shown below. Put it in its own class file called Drawable.java
public interface Drawable {
void Draw();
public enum state {DEAD, ALIVE};
The enumeration class state is a special Java class that lets you create a new type, state, with
very specific values. In our case, these values are DEAD and ALIVE. Here is the code for this
class.
This line must be inserted in a file called state.java. You will use this type when keeping track of
the the state of a cell. Here is a bit a code showing how you might use it.
states;
int x = 0;
if(0 == x)
else s
Cell
-s: state
-alive :char
-dead : char
Lets start with the Cell class. Here is the the UML class diagram for it:
s = state. DEAD; //access values via type name
state.ALIVE;
+Cell(s:state)
+Cell(s:state, alive :char, dead :char)
+getState(): state
+setState(s:state) : void
+Draw(): void
Next we have the abstract class Board
Board
#curr : Cell[][]
#alive :char
#dead : char
// you can create a variable
// of the enumurated type
+numLiveCell((i :int, j : int): int
+nextGen():void
The state of the cell
Character to draw on screen if cell is alive (default='#')
Character to draw on screen if cell is dead (default='')
Constructs a cell with the given state and default chars
Constructs a cell with the given state and characters
Returns state of cell
Sets state of cell.
Draw method implementation of Drawable interface
A 2-D array used to represent the board (or row)
Character to draw on screen if cell is alive (default='#')
Character to draw on screen if cell is dead (default='')
+Board(r:int; c:int)
Creates array curr with r rows and c columns
+Board(r:int, c:int, alive :char, dead :char) Like above but also changes printing characters
+setBoard (s:state) : void
Creates and sets the state of ALL cells in curr to s.
+setCell(i :int, j : int, c : Cell): void
+getCell(i :int, j : int) :Cell
+Draw(): void
Changes reference at curr[i][j] to c
Returns reference to cell found at curr[i][j]
Draw method implementation of Drawable interface
How many live cells exist around cell at curr[i][j]
Computes new generation based on old
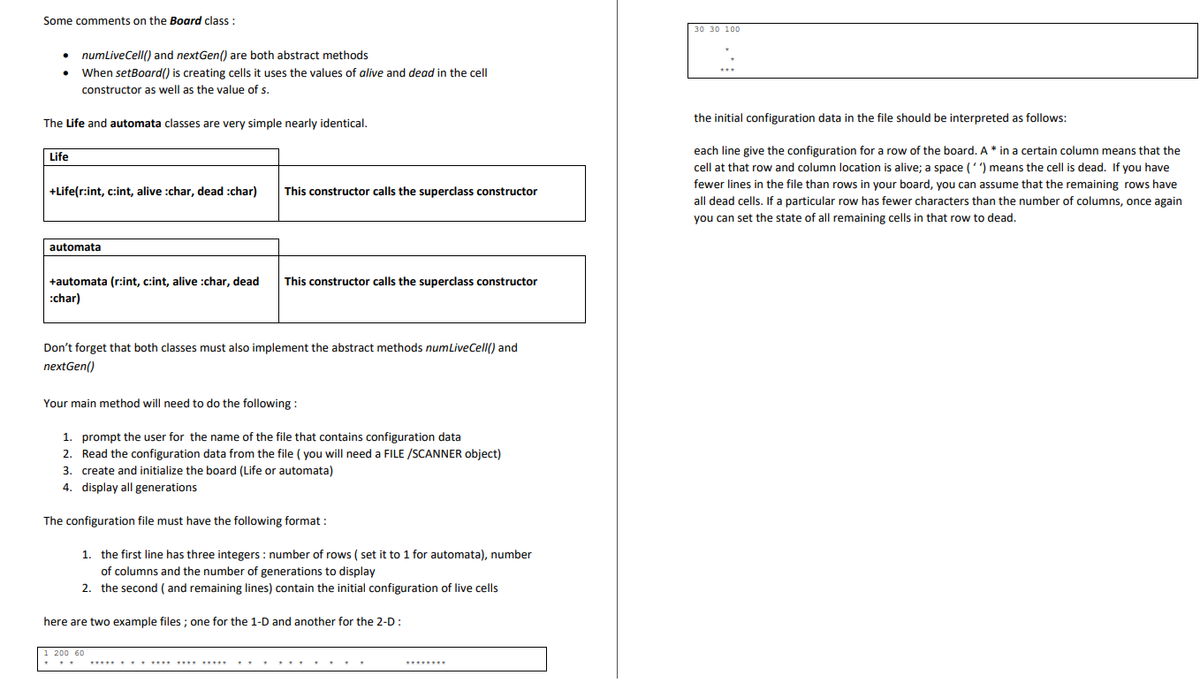
Transcribed Image Text:Some comments on the Board class :
●
numLiveCell() and nextGen() are both abstract methods
When setBoard() is creating cells it uses the values of alive and dead in the cell
constructor as well as the value of s.
The Life and automata classes are very simple nearly identical.
Life
+Life(r:int, c:int, alive :char, dead :char)
automata
+automata (r:int, c:int, alive :char, dead This constructor calls the superclass constructor
:char)
This constructor calls the superclass constructor
Don't forget that both classes must also implement the abstract methods numLiveCell() and
nextGen()
Your main method will need to do the following:
1. prompt the user for the name of the file that contains configuration data
2. Read the configuration data from the file (you will need a FILE /SCANNER object)
3. create and initialize the board (Life or automata)
4. display all generations
The configuration file must have the following format:
1. the first line has three integers: number of rows (set it to 1 for automata), number
of columns and the number of generations to display
2. the second (and remaining lines) contain the initial configuration of live cells
here are two example files; one for the 1-D and another for the 2-D :
1 200 60
***** ******* **** *****
********
30 30 100
the initial configuration data in the file should be interpreted as follows:
each line give the configuration for a row of the board. A * in a certain column means that the
cell at that row and column location is alive; a space ('') means the cell is dead. If you have
fewer lines in the file than rows in your board, you can assume that the remaining rows have
all dead cells. If a particular row has fewer characters than the number of columns, once again
you can set the state of all remaining cells in that row to dead.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
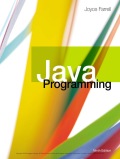
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
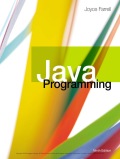
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT