Part 1: 1. Copy the source file Square class you crated in Lab 03 and paste the source file in the default package (you may need to fix any problems related to package declaration). 2. Open Square class using NetBeans editor. 3. Modify the class implementation to throw IllegalArgumentException if the side’s length is negative. You should modify the implementation of the arguments constructor and setSide method to throw the IllegalArgumentException. Use the following constructor header as a start: /** * Construct a Square object with the specified side’s length * @param side the side’s length of a square * @throws IllegalArgumentException if the side’s length is negative */ public Square (double side) throws IllegalArgumentException { // implement it } Part 2: 1. Write a program that prompts the user to read two integers and displays their sum.
Create a new Java Application project called lab_07
Part 1:
1. Copy the source file Square class you crated in Lab 03 and paste the source file in the default package (you may need to fix any problems related to package declaration).
2. Open Square class using NetBeans editor.
3. Modify the class implementation to throw IllegalArgumentException if the side’s length is negative.
You should modify the implementation of the arguments constructor and setSide method to throw the IllegalArgumentException.
Use the following constructor header as a start:
/**
* Construct a Square object with the specified side’s length
* @param side the side’s length of a square
* @throws IllegalArgumentException if the side’s length is negative
*/ public Square (double side) throws IllegalArgumentException {
// implement it
}
Part 2:
1. Write a program that prompts the user to read two integers and displays their sum.
Your program should prompt the user to enter the number again if the input is incorrect (if the input is not an integer number)
and display the message “Incorrect input”.
You must use Exceptions to handle incorrect input error.
Hint: Use InputMismatchException.
2. Modify the previous program, so it also displays the result of dividing first number by second number.
It should prompt the user to enter the number again if the divisor is zero and display the message “The divisor cannot be zero”.
You must use Exceptions to handle “divisor is zero” error.
Hint: Use ArithmeticException.
Part 3:
Write a program that creates an array with 100 randomly chosen integers.
Your program should prompt the user to enter an index of the array, then displays the corresponding element value.
It should prompt he user to enter the index again if the specified index is out of bounds and display the message “Index is out of bounds”.
You must use Exceptions to handle “index is out of bounds” error.
Hint: Use ArrayIndexOutOfBoundsException.
Here is Lab 03 program.
public class Square {
// default value for the variable side = 1
private double side = 1;
// create no-arg constructor
public Square(){
}
public Square( double side){
this.side = side;
}
//A method named getArea() that returns the area of this square.
public double getArea(){
double area = side * side / 2;
return area;
}
//A method named getPerimeter() that returns the perimeter of this square.
public double getParameter(){
double parameter = 4 * side;
return parameter;
}
//the getter to get the side length
public double getSide(){
return side;
}
//the setter to update the side length
public void setSide(double side){
this.side = side;
}
public static void main(String[] args) {
}
}
public class TestSquare {
// the main method
public static void main(String[] args) {
//the first object
Square s1 = new Square(5);
//the second object
Square s2 = new Square((int) 5.75);
System.out.println("OutPut for the the first object :");
System.out.println("The side length is :"+ s1.getSide());
System.out.println("The areaof square is : "+s1.getArea());
System.out.println("The Perimeter is "+ s1.getParameter());
System.out.println("------------------------------------------------");
System.out.println("OutPut for the second object :");
System.out.println("The side length is :"+ s2.getSide());
System.out.println("The areaof square is : "+s2.getArea());
System.out.println("The Perimeter is "+ s2.getParameter());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

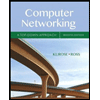
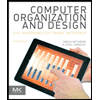
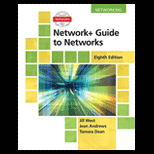
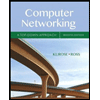
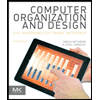
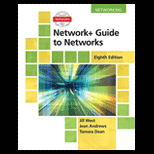
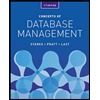
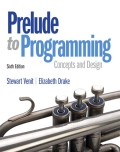
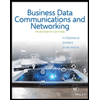