Part-2 (Encapsulation, Inheritance, Polymorphism) ____________________________________________________________________________ Create a Java project that demonstrates various object oriented concepts you have learned so far in this course (encapsulation, inheritance and polymorphism). To do this, create a super class and at least 2 subclasses. You can either do multilevel inheritance or hierarchical inheritance in this to complete this part-2 task. The super class will have following properties/functionalities: a) Create a total of 3 member variables for the class, selecting the appropriate data types for each field. b) Include an argument constructor in this Class, that will initialize all data fields. c) Encapsulate this Class. The two subclasses will have below properties/functionalities: a) One of the subclasses will have at least one member variable and other subclass with at least two member variables. b) Have two constructors in each subclass: • One constructor for initializing only super class data • Another constructor will initialize both super and subclass data. c) Encapsulate the subclasses. d) You should include overloaded methods in any of the classes you have created (superclass or subclass). e) Override the toString( ) method in each of your Classes. f) Override the equals( ) method in each of your Classes. The test class [with the main ( ) method] will perform below tasks: a) Create objects of superclass and each subclass types invoking all constructors and Draw the object diagram for each of the object created. b) Use setter methods to fill missing data in all objects. c) Generate outputs demonstrating overloading, overriding outputs. d) Create object of subclass [actual type] and store it in superclass object [declared type]. e) Generate output for instanceof operator. f) Do object casting (show both implicit and explicit) g) Generate output for constructor chaining [using this and super keywords]
Part-2 (Encapsulation, Inheritance, Polymorphism)
____________________________________________________________________________
Create a Java project that demonstrates various object oriented concepts you have learned so far
in this course (encapsulation, inheritance and polymorphism). To do this, create a super class and
at least 2 subclasses. You can either do multilevel inheritance or hierarchical inheritance in this
to complete this part-2 task.
The super class will have following properties/functionalities:
a) Create a total of 3 member variables for the class, selecting the appropriate data types
for each field.
b) Include an argument constructor in this Class, that will initialize all data fields.
c) Encapsulate this Class.
The two subclasses will have below properties/functionalities:
a) One of the subclasses will have at least one member variable and other subclass with at
least two member variables.
b) Have two constructors in each subclass:
• One constructor for initializing only super class data
• Another constructor will initialize both super and subclass data.
c) Encapsulate the subclasses.
d) You should include overloaded methods in any of the classes you have created
(superclass or subclass).
e) Override the toString( ) method in each of your Classes.
f) Override the equals( ) method in each of your Classes.
The test class [with the main ( ) method] will perform below tasks:
a) Create objects of superclass and each subclass types invoking all constructors and Draw
the object diagram for each of the object created.
b) Use setter methods to fill missing data in all objects.
c) Generate outputs demonstrating overloading, overriding outputs.
d) Create object of subclass [actual type] and store it in superclass object [declared type].
e) Generate output for instanceof operator.
f) Do object casting (show both implicit and explicit)
g) Generate output for constructor chaining [using this and super keywords]
Note:1) Draw object diagrams wherever possible and necessary to show the state of the objects
created
Include the below in your project report:
• The UML diagram of all Classes.
• Screenshots of all output generated from the applicatio
______________________________________________________________
Part-4: Java FX
_____________________________________________________________________________
Create a JavaFx project that demonstrates various topics you have learnt like, creating structure
of JavaFx, Panes, UI Controls, Shapes and events-driven
To do this, use the classes from Part-2. Create a window that consists of the following:
• Window title: “Title of your project”.
• Design your window by using different User Interface Control like Text Field, Labels,
Checkbox / Radio button, Combo box, List box, etc.... Use the data fields from the part-2,
to design the nodes of the window. (Minimum five different user interface controls
should be used.
• Use the concept of the Events Driven Programming (EDP) in order to activate the
following GUI components (Buttons, radio buttons, check box, etc..) to activate the
events to some nodes in your window to implement actions. (Minimum three different
events should be created)
Include the below in your project report:
• Screenshots of all output generated from the application.
• Code for all classes.
ITDR2104 - Programming F
![Part-2 (Encapsulation, Inheritance, Polymorphism)
Create a Java project that demonstrates various object oriented concepts you ha ve learned so far
in this course (encapsulation, inheritance and polymorphism). To do this, create a super class and
at least 2 subclasses. You can either do multilevel inheritance or hierarchical inheritance in this
to complete this part-2 task.
The super class will have following properties/functionalities:
a) Create a total of 3 member variables for the class, selecting the appropriate data types
for each field.
b) Include an argument constructor in this Class, that will initialize all data fields.
c) Encapsulate this Class.
The two subclasses will have below properties/functionalities:
a) One of the subclasses will have at least one member variable and other subclass with at
least two member variables.
b) Have two constructors in each subclass:
One constructor for initializing only super class data
Another constructor will initialize both super and subclass data.
c) Encapsulate the subclasses.
d) You should include overloaded methods in any of the classes you have created
(superclass or subclass).
e) Override the toString() method in each of your Classes.
f) Override the equals( ) method in each of your Classes.
The test class [with the main () method] will perform below tasks:
a) Create objects of superclass and each subclass types invoking all constructors and Draw
the object diagram for each of the object created.
b) Use setter methods to fill missing data in all objects.
c) Generate outputs demonstrating overloading, overriding outputs.
d) Create object of subclass [actual type] and store it in superclass object [declared type].
e) Generate output for instanceof operator.
f) Do object casting (show both implicit and explicit)
g) Generate output for constructor chaining [using this and super keywords]
Note:
1) Draw object diagrams wherever possible and necessary to show the state of the objects
created
Include the below in your project report:
The UML diagram of all Classes.
Screenshots of all output generated from the application.
ITDR2104 - Programming
Fall 2020](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd30e915f-ece1-4eb6-aed7-63c57e2ac611%2F06e0afca-cbb6-4327-8e6c-ea24702f4632%2Fpkj5vfa_processed.jpeg&w=3840&q=75)
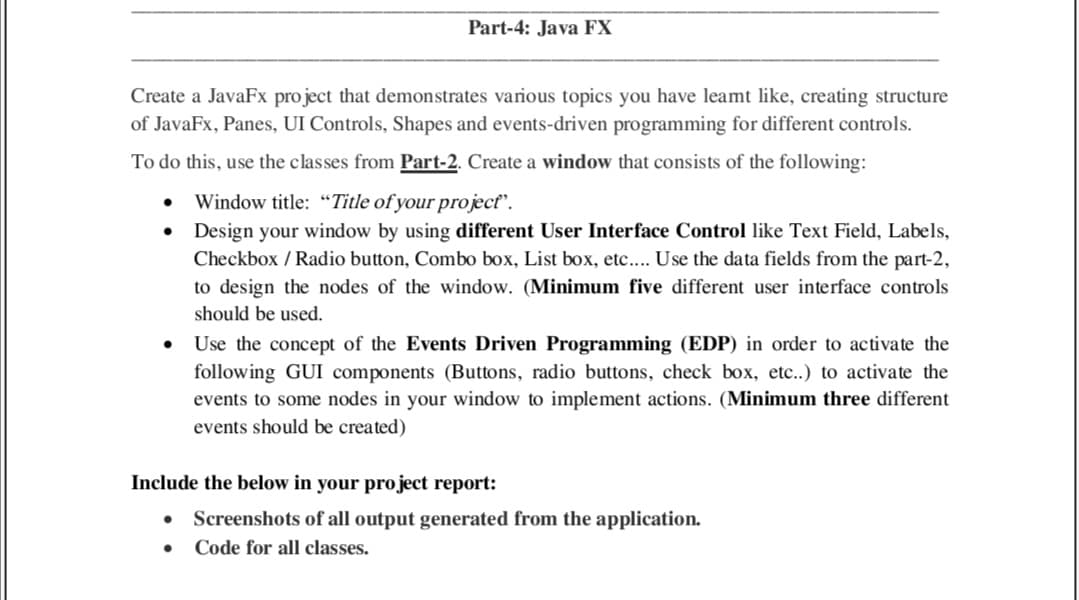

Step by step
Solved in 2 steps

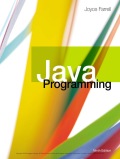
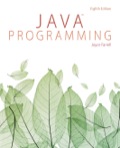
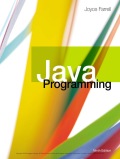
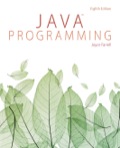