Part B-The Constructor and Method getVowels() (30 – 40 minutes) 1. Driver: Complete the constructor. It should be straightforward. 2. Method getVowels () returns all vowels of the stored text. For example, if the stored text is "monopoly", then the return value should be "o00". It is useful to think about "building blocks:" snippets of code that may help you attack this problem. These are things you already know how to do, and you may be able to put various ideas together to solve the current problem. Here are a few things you know how to do: a. Extracting the ith character of a string char ch str.charAt (i); %3D You could call method substring() to get a string containing the ith character. b. Looping over all characters in a string for (int i = 0; i < str.length (); i++) ... c. Adding a character to a string, which could be empty result = result + ch; d. Checking whether a letter is a vowel
Part B-The Constructor and Method getVowels() (30 – 40 minutes) 1. Driver: Complete the constructor. It should be straightforward. 2. Method getVowels () returns all vowels of the stored text. For example, if the stored text is "monopoly", then the return value should be "o00". It is useful to think about "building blocks:" snippets of code that may help you attack this problem. These are things you already know how to do, and you may be able to put various ideas together to solve the current problem. Here are a few things you know how to do: a. Extracting the ith character of a string char ch str.charAt (i); %3D You could call method substring() to get a string containing the ith character. b. Looping over all characters in a string for (int i = 0; i < str.length (); i++) ... c. Adding a character to a string, which could be empty result = result + ch; d. Checking whether a letter is a vowel
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
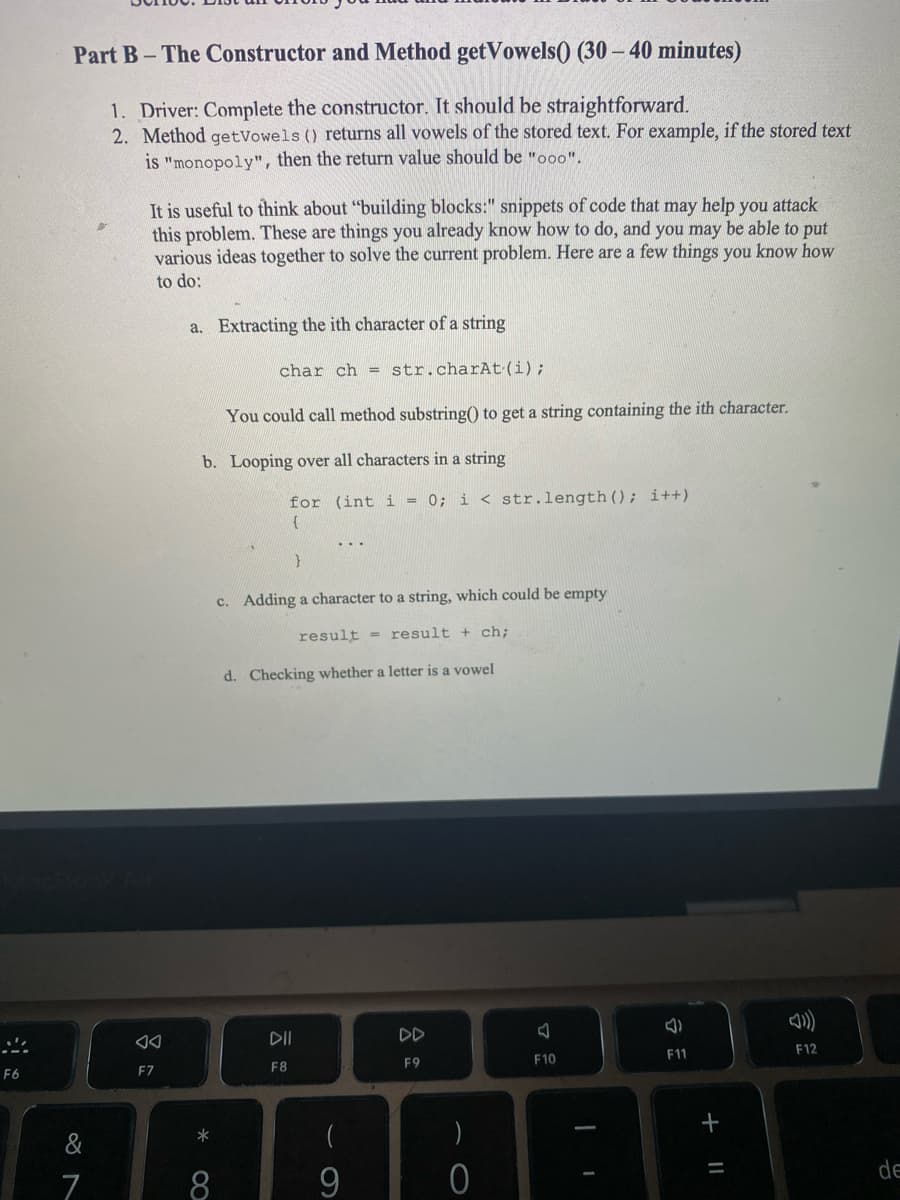
Transcribed Image Text:Part B- The Constructor and Method getVowels() (30 – 40 minutes)
1. Driver: Complete the constructor. It should be straightforward.
2. Method getVowels () returns all vowels of the stored text. For example, if the stored text
is "monopoly", then the return value should be "ooo".
It is useful to think about "building blocks:" snippets of code that may help you attack
this problem. These are things you already know how to do, and you may be able to put
various ideas together to solve the current problem. Here are a few things you know how
to do:
a. Extracting the ith character of a string
char ch = str.charAt (i);
You could call method substring() to get a string containing the ith character.
b. Looping over all characters in a string
for (int i = 0; i < str.length (); i++)
c. Adding a character to a string, which could be empty
result = result + ch;
d. Checking whether a letter is a vowel
DII
DD
F11
F12
F9
F10
F7
F8
F6
de
7
8
9.
令
+
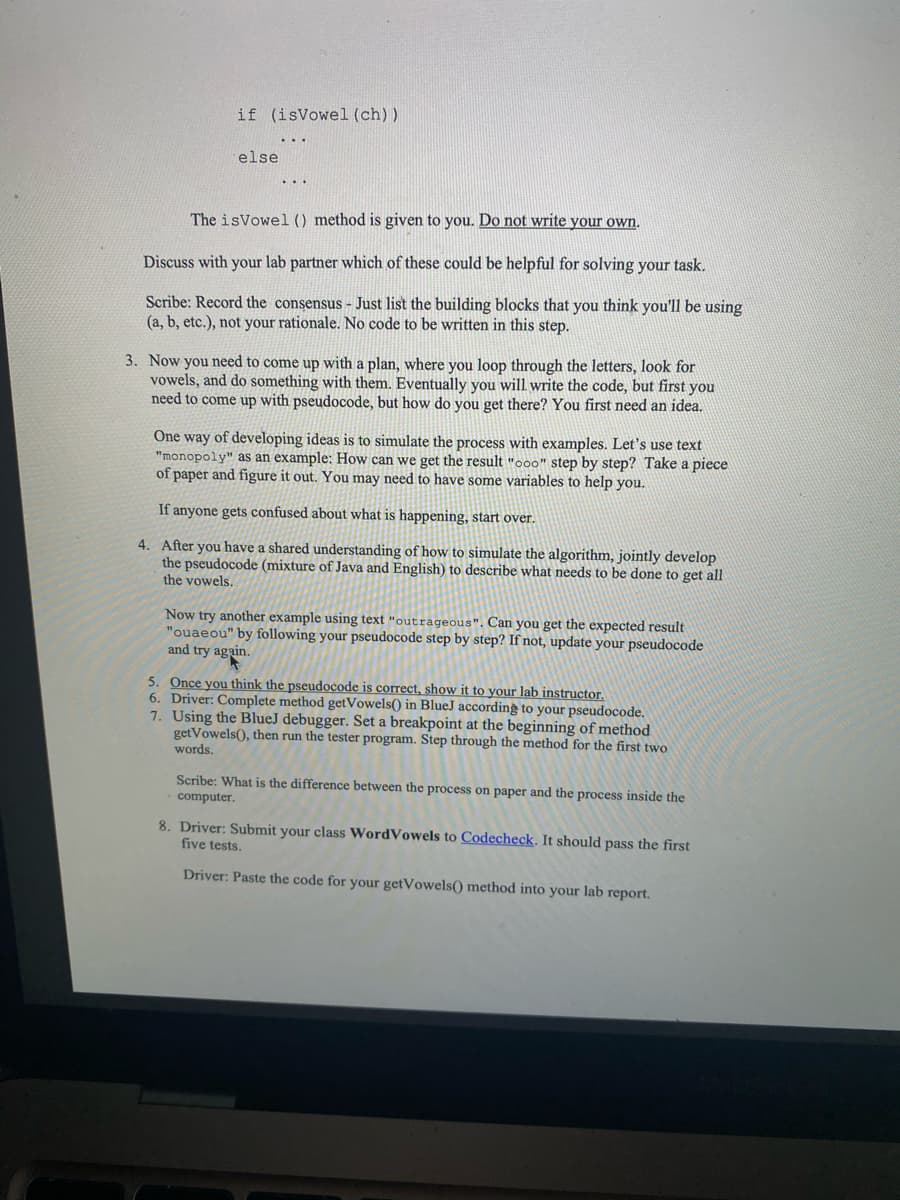
Transcribed Image Text:if (isVowel (ch))
else
The isVowel () method is given to you. Do not write your own.
Discuss with your lab partner which of these could be helpful for solving your task.
Scribe: Record the consensus - Just list the building blocks that you think you'll be using
(a, b, etc.), not your rationale. No code to be written in this step.
3. Now you need to come up with a plan, where you loop through the letters, look for
vowels, and do something with them. Eventually you will write the code, but first you
need to come up with pseudocode, but how do you get there? You first need an idea.
One way of developing ideas is to simulate the process with examples. Let's use text
"monopoly" as an example: How can we get the result "ooo" step by step? Take a piece
of paper and figure it out. You may need to have some variables to help you.
If anyone gets confused about what is happening, start over.
4. After you have a shared understanding of how to simulate the algorithm, jointly develop
the pseudocode (mixture of Java and English) to describe what needs to be done to get all
the vowels.
Now try another example using text "outrageous". Can you get the expected result
"ouaeou" by following your pseudocode step by step? If not, update your pseudocode
and try again.
5. Once you think the pseudocode is correct, show it to your lab instructor,
6. Driver: Complete method getVowels() in BlueJ according to your pseudocode.
7. Using the BlueJ debugger. Set a breakpoint at the beginning of method
getVowels(), then run the tester program. Step through the method for the first two
words.
Scribe: What is the difference between the process on paper and the process inside the
computer.
8. Driver: Submit your class WordVowels to Codecheck. It should pass the first
five tests.
Driver: Paste the code for your getVowels() method into your lab report.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
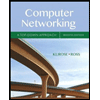
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
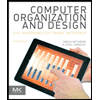
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
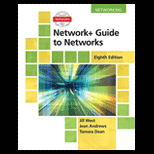
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
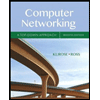
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
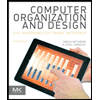
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
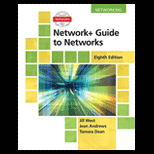
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
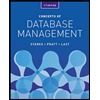
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
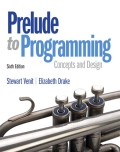
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
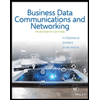
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY