Part II While HeapSort (as we saw in Studio 6) is more amenable than MergeSort to an in-place implementation, MergeSort has its own advantages. For this problem, suppose you want to perform MergeSort on a really huge array A. The array is so big that it doesn't fit in your computer's memory and has to be stored in the cloud. More specifically, assume that our computer has enough memory to hold 36 elements, for some constant b, but A has size n much greater than b. We can call read (X, i, B) to read a chunk of b elements from an array X (in the cloud) starting at index i into a local array B. Similarly, we can call write (C, X, i) to write a chunk of b elements stored in local array C to a cloud array X starting at index i. Here's a proposed (incomplete!) implementation of the merge operation that merges cloud arrays X and Y into cloud array Z. The code uses local arrays A, B, and C, each of size b, to cache X, Y, and Z. For simplicity, we assume that the input arrays X and Y have sizes a multiple of b, and that reading past the end of either X or Y returns values ∞ as in the studio. "mod" is the integer modulo operator (% in Java). MERGE(X, Y, Z) i +0 j+0 k +0 READ(X, 0, A) READ(Y, 0, B) while A[i mod b] <∞ or B[j mod b] < ∞ do u ← A[i mod b] v← B[j mod b] C[k mod b] = min(u, v) if u
Part II While HeapSort (as we saw in Studio 6) is more amenable than MergeSort to an in-place implementation, MergeSort has its own advantages. For this problem, suppose you want to perform MergeSort on a really huge array A. The array is so big that it doesn't fit in your computer's memory and has to be stored in the cloud. More specifically, assume that our computer has enough memory to hold 36 elements, for some constant b, but A has size n much greater than b. We can call read (X, i, B) to read a chunk of b elements from an array X (in the cloud) starting at index i into a local array B. Similarly, we can call write (C, X, i) to write a chunk of b elements stored in local array C to a cloud array X starting at index i. Here's a proposed (incomplete!) implementation of the merge operation that merges cloud arrays X and Y into cloud array Z. The code uses local arrays A, B, and C, each of size b, to cache X, Y, and Z. For simplicity, we assume that the input arrays X and Y have sizes a multiple of b, and that reading past the end of either X or Y returns values ∞ as in the studio. "mod" is the integer modulo operator (% in Java). MERGE(X, Y, Z) i +0 j+0 k +0 READ(X, 0, A) READ(Y, 0, B) while A[i mod b] <∞ or B[j mod b] < ∞ do u ← A[i mod b] v← B[j mod b] C[k mod b] = min(u, v) if u
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
10, 11, 12
![Part II
While HeapSort (as we saw in Studio 6) is more amenable than MergeSort to an in-place implementation,
MergeSort has its own advantages. For this problem, suppose you want to perform MergeSort on a really
huge array A. The array is so big that it doesn't fit in your computer's memory and has to be stored in the
cloud.
More specifically, assume that our computer has enough memory to hold 36 elements, for some constant
b, but A has size n much greater than b. We can call read (X, i, B) to read a chunk of b elements from an
array X (in the cloud) starting at index i into a local array B. Similarly, we can call write (C, X, i) to
write a chunk of 6 elements stored in local array C to a cloud array X starting at index i.
Here's a proposed (incomplete!) implementation of the merge operation that merges cloud arrays X and
Y into cloud array Z. The code uses local arrays A, B, and C, each of size b, to cache X, Y, and Z. For
simplicity, we assume that the input arrays X and Y have sizes a multiple of b, and that reading past the
end of either X or Y returns values ∞o as in the studio. "mod" is the integer modulo operator (% in Java).
MERGE(X, Y, Z)
i +0
j40
k 0
READ(X, 0, A)
READ(Y, 0, B)
while A[i mod b] <∞ or B[j mod b] <∞ do
u ← A[i mod b]
v← Bj mod b]
C[k mod b] = min(u, v)
if u < v
BLOCK1
else
BLOCK2
k++
if k mod b = 0
WRITE(C, Z, k-b)
10. Supply blocks of pseudocode to replace the lines "BLOCK1" and "BLOCK2" to complete the imple-
mentation of the merge operation. Consider which parts of the regular merge algorithm are missing,
and when/how to fetch more data from the cloud.
11. Exactly how many times must the merge function call each of read and write to merge two arrays
of size n/2 into an array of size n, assuming that n/2 is divisible by b? (Hint: Beware off-by-1 errors
when thinking about how many elements must be examined!)
12. What is the total number of cloud read and write operations performed by MergeSort to sort an array
A of size n stored in the cloud? Give an asymptotic answer in terms of n, the number of elements in
A, and b, the chunk size. How does this cost compare to the asymptotic cost of merge-sorting an array
of size n held entirely in your computer's memory?
13. Why might MergeSort be preferable to HeapSort in the cloud sorting model, where the cost is based
on the number of chunk reads and writes? If there is an asymptotic difference between MergeSort and
HeapSort in the cloud, include it in your argument.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F92856043-199e-49b6-95ce-27336f8d0cbd%2Fa215fe1b-c551-4faa-a8ae-6cff58f1cfb1%2Fzs3fjt_processed.png&w=3840&q=75)
Transcribed Image Text:Part II
While HeapSort (as we saw in Studio 6) is more amenable than MergeSort to an in-place implementation,
MergeSort has its own advantages. For this problem, suppose you want to perform MergeSort on a really
huge array A. The array is so big that it doesn't fit in your computer's memory and has to be stored in the
cloud.
More specifically, assume that our computer has enough memory to hold 36 elements, for some constant
b, but A has size n much greater than b. We can call read (X, i, B) to read a chunk of b elements from an
array X (in the cloud) starting at index i into a local array B. Similarly, we can call write (C, X, i) to
write a chunk of 6 elements stored in local array C to a cloud array X starting at index i.
Here's a proposed (incomplete!) implementation of the merge operation that merges cloud arrays X and
Y into cloud array Z. The code uses local arrays A, B, and C, each of size b, to cache X, Y, and Z. For
simplicity, we assume that the input arrays X and Y have sizes a multiple of b, and that reading past the
end of either X or Y returns values ∞o as in the studio. "mod" is the integer modulo operator (% in Java).
MERGE(X, Y, Z)
i +0
j40
k 0
READ(X, 0, A)
READ(Y, 0, B)
while A[i mod b] <∞ or B[j mod b] <∞ do
u ← A[i mod b]
v← Bj mod b]
C[k mod b] = min(u, v)
if u < v
BLOCK1
else
BLOCK2
k++
if k mod b = 0
WRITE(C, Z, k-b)
10. Supply blocks of pseudocode to replace the lines "BLOCK1" and "BLOCK2" to complete the imple-
mentation of the merge operation. Consider which parts of the regular merge algorithm are missing,
and when/how to fetch more data from the cloud.
11. Exactly how many times must the merge function call each of read and write to merge two arrays
of size n/2 into an array of size n, assuming that n/2 is divisible by b? (Hint: Beware off-by-1 errors
when thinking about how many elements must be examined!)
12. What is the total number of cloud read and write operations performed by MergeSort to sort an array
A of size n stored in the cloud? Give an asymptotic answer in terms of n, the number of elements in
A, and b, the chunk size. How does this cost compare to the asymptotic cost of merge-sorting an array
of size n held entirely in your computer's memory?
13. Why might MergeSort be preferable to HeapSort in the cloud sorting model, where the cost is based
on the number of chunk reads and writes? If there is an asymptotic difference between MergeSort and
HeapSort in the cloud, include it in your argument.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
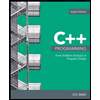
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
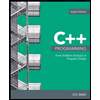
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning