please answer if the question is true or false In the Recursive Binary Search shown below, there are two base cases: Line 10: the search is exhausted without a finding a value that matches the key Line 16: the search finds a value that matches the key 1 public class RecursiveBinarySearch { 2 public static int binarySearch(int[] list, int key) { 3 int low = 0; 4 int high = list.length - 1; 5 return binarySearch(list, key, low, high); 6 } 7 8 private static int binarySearch(int[] list, int key, 9 int low, int high) { 10 if (low > high) // The list has been exhausted without a match 11 return -low - 1; 12 13 int mid = (low + high) / 2; 14 if (key < list[mid]) 15 return binarySearch(list, key, low, mid - 1); 16 else if (key == list[mid]) 17 return mid; 18 else 19 return binarySearch(list, key, mid + 1, high); 20 } 21 22 public static void main(String[] args) { 23 int[] list = {3, 5, 7, 8, 12, 17, 24, 29}; 24 System.out.println(binarySearch(list, 7)); 25 System.out.println(binarySearch(list, 0)); 26 } 27 } True False
please answer if the question is true or false
In the Recursive Binary Search shown below, there are two base cases:
- Line 10: the search is exhausted without a finding a value that matches the key
- Line 16: the search finds a value that matches the key
1 public class RecursiveBinarySearch {
2 public static int binarySearch(int[] list, int key) {
3 int low = 0;
4 int high = list.length - 1;
5 return binarySearch(list, key, low, high);
6 }
7
8 private static int binarySearch(int[] list, int key,
9 int low, int high) {
10 if (low > high) // The list has been exhausted without a match
11 return -low - 1;
12
13 int mid = (low + high) / 2;
14 if (key < list[mid])
15 return binarySearch(list, key, low, mid - 1);
16 else if (key == list[mid])
17 return mid;
18 else
19 return binarySearch(list, key, mid + 1, high);
20 }
21
22 public static void main(String[] args) {
23 int[] list = {3, 5, 7, 8, 12, 17, 24, 29};
24 System.out.println(binarySearch(list, 7));
25 System.out.println(binarySearch(list, 0));
26 }
27 }
True
False

Step by step
Solved in 3 steps

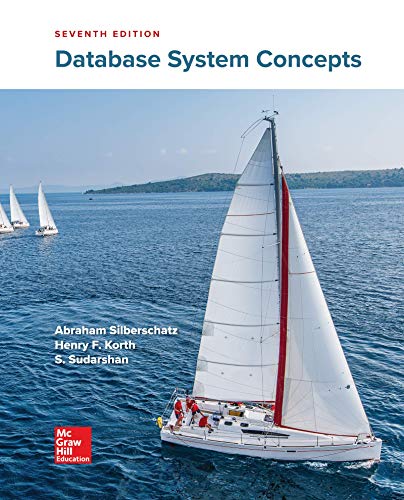
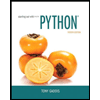
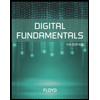
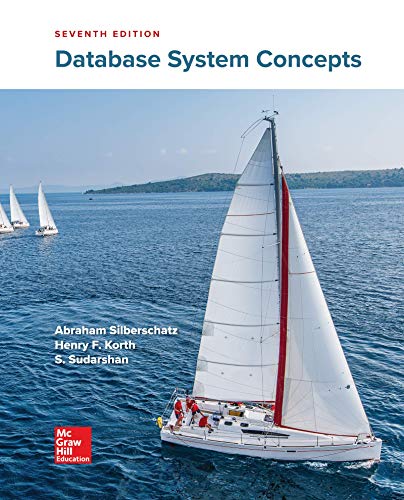
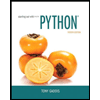
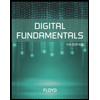
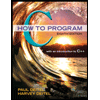
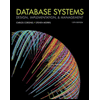
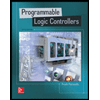