Please design a "Poly" class to simulate a polynomial with real coefficients and follow the requirements. Implement Poly.h & Poly.cpp, and write down output of demo.cpp private data: 1. int power; // store the maximal power of the polynomial 2. double * coef; // store all the coefficients of the polynomial • public static data: 1. int count; // store the number of polynomial objects 2. string comment; // please input your comments of IC-II (at least 50 words) public member functions: 1. Poly(); // default constructor, build a polynomial of power 2 and all coefficients = 1 2. Poly(int); // set up the maximal power of the polynomial with all coefficients = 1 3. Poly(int, double []); // convert the array into a polynomial. The array stores the coefficients of power 0, 1, 2, .. in order. int is "size of array" // copy a polynomial // destructor 4. copy constructor 5. destructor > 6. void setPower(int); // set up the maximal power of the polynomial I/ get the maximal power of the polynomial // set up the coefficient of the power (power, coef). Coefficient of maximal power can't be 0. // get the coefficient of the power (power) // get the number of objects now 7. int getPower() const; 8. void setCoef(int, double); 9.double getCoef(int) const; 10.static int getCount(); 11.void display() { // display the polynomial cout << coef[power]; for (int i = power;i > 0; ) { cout << "xA" << i; do { --i; } while (i>-1 && coef[i]==0); if(i<0) break; cout <<" "; if (coef[i) > 0 ) cout << "+" cout << coef(i)]; } cout << end%;
demo.cpp
#include "Poly.h"
#include<iostream>
using namespace std;
int main() {
Poly p1;
couble d[3]={1,2,3}:
Poly p2(3, d);
Poly p3(p2);
cout << "Polynomial p1 is: ";
p1.display();
cout << "Polynomial p2 is: " << p2 << endl;
cout << "Polynomial p3 is: " << p3 << endl;
if (p2 == p3) {
cout << "p2 and p3 are the same.";
}
else {
cout << "p2 and p3 are different.";
}
cout << endl;
cout << "The coefficient of power 1 of p3 is: " << p2.getCoef(1) << endl;
p3.setCoef(1, 99);
cout << "p3 after setup is: " << p3 << endl;
if (p2 == p3) {
cout << "p2 and p3 are the same.";
}
else {
cout << "p2 and p3 are different.";
}
cout << endl;
Poly p4;
cout << "Please enter the power and coefficients of Polynomial p4: " << endl;
cin >> p4;
cout << "Polynomial p4 is: " << p4 << endl;
Poly p5 = p2 + p3;
cout << "p2+p3 is: " << p5 << endl;
p5 = p2 + 3.1;
cout << "p2+3.1 is: " << p5 << endl;
p5 += p2;
cout << "p2+p2+3.1 is: " << p5 << endl;
p5++;
cout << "p2+p2+3.1+1 is: " << p5 << endl;
p5 = p2 * p3;
cout << "p2*p3 is: " << p5 << endl;
p5 = !p4;
cout << "p4 after reversing the sign is: " << p5 << endl;
cout << "The number of polynomials now is: " << Poly::getCount() << endl;
cout << "The comment for this class is: " << Poly::comment << endl;
return 0;
}

![Please design a "Poly" class to simulate a polynomial with real coefficients and follow
the requirements. Implement Poly.h & Poly.cpp, and write down output of demo.cpp
-===
private data:
// store the maximal power of the polynomial
// store all the coefficients of the polynomial
1. int power;
2. double * coef;
public static data:
1. int count;
// store the number of polynomial objects
2. string comment;
// please input your comments of IC-II (at least 50 words)
public member functions:
1. Poly();
// default constructor, build a polynomial of power 2 and all
coefficients = 1
2. Poly(int);
// set up the maximal power of the polynomial with all
coefficients = 1
3. Poly(int, double [1); // convert the array into a polynomial. The array stores the
coefficients of power 0, 1, 2, . in order. int is "size of array"
4. copy constructor
5. destructor
// copy a polynomial
// destructor
→ 6. void setPower(int);
7. int getPower() const;
ef
// get the maximal power of the polynomial
// set up the coefficient of the power (power,
coef). Coefficient of maximal power can't be 0.
// set up the maximal power of the polynomial ponr Eu
8. void setCoef(int, double);
9.double getCoef(int) const;
// get the coefficient of the power (power)
10.static int getCount();
// get the number of objects now
11.void display() {
// display the polynomial
cout << coef[power];
for (int i = power ; i> 0; ) {
cout << "xA" << i;
do {--i; } while (i>-1 && coef[i)==0);
if(i<0) break;
cout <<" ";
if (coef[i) > 0) cout << "+";
cout << coef(i];
} cout << endl;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff619ad36-1d6e-4fad-b0bd-b681a9cba0db%2Fe2593cbc-8a28-428e-939b-1e7e3bccb9db%2Fz1cfhcc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

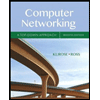
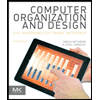
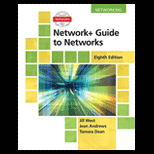
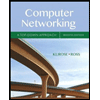
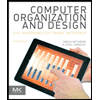
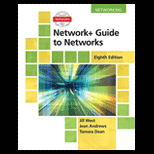
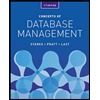
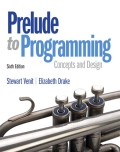
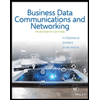