Please do not give solution in image format thanku I have the following subclass that extends from a main class. Can you give me a brief explanation talking about all of the attributes, methods and etc. I want it in a very professional way please. here's the code package Banking_Management_Sys; public class MortgageAccount extends CustomersAccounts { private double loanAmount; private double interestRate; private int loanTerm; private int paymentFrequency; private String lender; public MortgageAccount() { } public MortgageAccount(int accountnum, String type, double balance, String dateopened, String dateclosed, String owner, double loanAmount, double interestRate, int loanTerm, int paymentFrequency, String lender) { super(accountnum, type, balance, dateopened, dateclosed, owner); this.loanAmount = loanAmount; this.interestRate = interestRate; this.loanTerm = loanTerm; this.paymentFrequency = paymentFrequency; this.lender = lender; } public double getLoanAmount() { return loanAmount; } public void setLoanAmount(double loanAmount) { this.loanAmount = loanAmount; } public double getInterestRate() { return interestRate; } public void setInterestRate(double interestRate) { this.interestRate = interestRate; } public int getLoanTerm() { return loanTerm; } public void setLoanTerm(int loanTerm) { this.loanTerm = loanTerm; } public int getPaymentFrequency() { return paymentFrequency; } public void setPaymentFrequency(int paymentFrequency) { this.paymentFrequency = paymentFrequency; } public String getLender() { return lender; } public void setLender(String lender) { this.lender = lender; } public double calculateMonthlyPayment() { try { return loanAmount * (interestRate / 12) / (1 - Math.pow(1 + interestRate / 12, -loanTerm)); } catch (ArithmeticException e) { System.err.println("Error calculating monthly payment: " + e.getMessage()); return 0.0; } } public double calculateInterest() { return loanAmount * interestRate * (loanTerm / 12); } public double checkBalance() { try { return super.checkBalance() - calculateMonthlyPayment(); } catch (Exception e) { System.err.println("Error checking balance: " + e.getMessage()); return 0.0; } } public void makePayment(double amount) { try { setAccountBalance(checkBalance() - amount); } catch (Exception e) { System.err.println("Error making payment: " + e.getMessage()); } } @Override public String toString() { return String.format("Mortgage Account:\n" + "Account Number: \n" + "Account Type: \n" + "Date Opened: \n" + "Account Balance: \n" + "Date Closed: \n" + "Account Owner: \n" + "Loan Amount: \n" + "Interest Rate: \n" + "Loan Term: \n" + "Payment Frequency: \n" + "Lender: \n" + "Monthly Payment: \n" + "Interest: \n" + "Remaining Balance: ", getAccountNumber(), getAccountType(), getDateOpened(), checkBalance(), getDateClosed(), getAcoountOwner(), loanAmount, interestRate, loanTerm, paymentFrequency, lender, calculateMonthlyPayment(), calculateInterest(), checkBalance()); } }
Please do not give solution in image format thanku
I have the following subclass that extends from a main class. Can you give me a brief explanation talking about all of the attributes, methods and etc.
I want it in a very professional way please.
here's the code
package Banking_Management_Sys;
public class MortgageAccount extends CustomersAccounts {
private double loanAmount;
private double interestRate;
private int loanTerm;
private int paymentFrequency;
private String lender;
public MortgageAccount() {
}
public MortgageAccount(int accountnum, String type, double balance, String dateopened, String dateclosed, String owner,
double loanAmount, double interestRate, int loanTerm, int paymentFrequency, String lender) {
super(accountnum, type, balance, dateopened, dateclosed, owner);
this.loanAmount = loanAmount;
this.interestRate = interestRate;
this.loanTerm = loanTerm;
this.paymentFrequency = paymentFrequency;
this.lender = lender;
}
public double getLoanAmount() {
return loanAmount;
}
public void setLoanAmount(double loanAmount) {
this.loanAmount = loanAmount;
}
public double getInterestRate() {
return interestRate;
}
public void setInterestRate(double interestRate) {
this.interestRate = interestRate;
}
public int getLoanTerm() {
return loanTerm;
}
public void setLoanTerm(int loanTerm) {
this.loanTerm = loanTerm;
}
public int getPaymentFrequency() {
return paymentFrequency;
}
public void setPaymentFrequency(int paymentFrequency) {
this.paymentFrequency = paymentFrequency;
}
public String getLender() {
return lender;
}
public void setLender(String lender) {
this.lender = lender;
}
public double calculateMonthlyPayment() {
try {
return loanAmount * (interestRate / 12) / (1 - Math.pow(1 + interestRate / 12, -loanTerm));
} catch (ArithmeticException e) {
System.err.println("Error calculating monthly payment: " + e.getMessage());
return 0.0;
}
}
public double calculateInterest() {
return loanAmount * interestRate * (loanTerm / 12);
}
public double checkBalance() {
try {
return super.checkBalance() - calculateMonthlyPayment();
} catch (Exception e) {
System.err.println("Error checking balance: " + e.getMessage());
return 0.0;
}
}
public void makePayment(double amount) {
try {
setAccountBalance(checkBalance() - amount);
} catch (Exception e) {
System.err.println("Error making payment: " + e.getMessage());
}
}
@Override
public String toString() {
return String.format("Mortgage Account:\n" + "Account Number: \n" + "Account Type: \n" +
"Date Opened: \n" + "Account Balance: \n" + "Date Closed: \n" + "Account Owner: \n" +
"Loan Amount: \n" + "Interest Rate: \n" + "Loan Term: \n" + "Payment Frequency: \n" + "Lender: \n" +
"Monthly Payment: \n" + "Interest: \n" + "Remaining Balance: ",
getAccountNumber(), getAccountType(), getDateOpened(), checkBalance(), getDateClosed(), getAcoountOwner(),
loanAmount, interestRate, loanTerm, paymentFrequency, lender, calculateMonthlyPayment(),
calculateInterest(), checkBalance());
}
}

Step by step
Solved in 3 steps

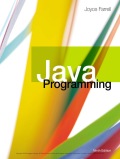
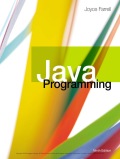