please fix the following , when user log in with debit# and pin and choose 1 for deposit and you choose negative amount , it accept it, i need it to only accpet positive amount import tkinter as tk from datetime import datetime, timedelta class ATM: def __init__(self): self.accounts = {} def create_account(self): # Get user inputs first_name = first_name_entry.get() last_name = last_name_entry.get() dob = dob_entry.get() address = address_entry.get() debit_card = debit_card_entry.get() while len(debit_card) != 16 or not debit_card.isdigit(): debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ") pin = pin_entry.get() while len(pin) != 4 or not pin.isdigit(): pin = input("Invalid pin. Enter 4-digit pin: ") # Create account and update GUI self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address, "pin": pin, "balance": 0} status_label.config(text="Account created successfully!") def login(self): debit_card = login_debit_card_entry.get() pin = login_pin_entry.get() if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin: while True: choice = login_choice_entry.get() if choice == "1": amount = float(deposit_entry.get()) self.accounts[debit_card]["balance"] += amount status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "2": amount = float(withdraw_entry.get()) if amount <= self.accounts[debit_card]["balance"]: self.accounts[debit_card]["balance"] -= amount status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break else: status_label.config(text="Insufficient balance.") break elif choice == "3": status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "4": status_label.config(text="Logged out.") break else: status_label.config(text="Invalid choice.") break else: status_label.config(text="Invalid debit card or pin.") atm = ATM() # create GUI root = tk.Tk() root.title("ATM software") create_account_frame = tk.Frame(root) create_account_frame.pack(padx=10, pady=10) tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0) first_name_entry = tk.Entry(create_account_frame) first_name_entry.grid(row=0, column=1) tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0) last_name_entry = tk.Entry(create_account_frame) last_name_entry.grid(row=1, column=1) tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0) dob_entry = tk.Entry(create_account_frame) dob_entry.grid(row=2, column=1) tk.Label(create_account_frame, text="Enter address:").grid(row=3, column=0) address_entry = tk.Entry(create_account_frame) address_entry.grid(row=3, column=1) tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0) debit_card_entry = tk.Entry(create_account_frame) debit_card_entry.grid(row=4, column=1) tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0) pin_entry = tk.Entry(create_account_frame, show="*") pin_entry.grid(row=5, column=1) create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account) create_account_button.grid(row=6, column=0, columnspan=2) login_frame = tk.Frame(root) login_frame.pack(padx=10, pady=10) tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0) login_debit_card_entry = tk.Entry(login_frame) login_debit_card_entry.grid(row=0, column=1) tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0) login_pin_entry = tk.Entry(login_frame, show="*") login_pin_entry.grid(row=1, column=1) tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0) login_choice_entry = tk.Entry(login_frame) login_choice_entry.grid(row=2, column=1) deposit_entry = tk.Entry(login_frame) deposit_entry.grid(row=3, column=0) withdraw_entry = tk.Entry(login_frame) withdraw_entry.grid(row=3, column=1) login_button = tk.Button(login_frame, text="Login", command=atm.login) login_button.grid(row=4, column=0, columnspan=2) status_label = tk.Label(root, text="") status_label.pack() root.mainloop()
please fix the following , when user log in with debit# and pin and choose 1 for deposit and you choose negative amount , it accept it, i need it to only accpet positive amount
import tkinter as tk
from datetime import datetime, timedelta
class ATM:
def __init__(self):
self.accounts = {}
def create_account(self):
# Get user inputs
first_name = first_name_entry.get()
last_name = last_name_entry.get()
dob = dob_entry.get()
address = address_entry.get()
debit_card = debit_card_entry.get()
while len(debit_card) != 16 or not debit_card.isdigit():
debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ")
pin = pin_entry.get()
while len(pin) != 4 or not pin.isdigit():
pin = input("Invalid pin. Enter 4-digit pin: ")
# Create account and update GUI
self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address,
"pin": pin, "balance": 0}
status_label.config(text="Account created successfully!") def login(self):
debit_card = login_debit_card_entry.get()
pin = login_pin_entry.get()
if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin:
while True:
choice = login_choice_entry.get()
if choice == "1":
amount = float(deposit_entry.get())
self.accounts[debit_card]["balance"] += amount
status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
elif choice == "2":
amount = float(withdraw_entry.get())
if amount <= self.accounts[debit_card]["balance"]:
self.accounts[debit_card]["balance"] -= amount
status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
else:
status_label.config(text="Insufficient balance.")
break
elif choice == "3":
status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"])
break
elif choice == "4":
status_label.config(text="Logged out.")
break
else:
status_label.config(text="Invalid choice.")
break
else:
status_label.config(text="Invalid debit card or pin.")
atm = ATM()
# create GUI
root = tk.Tk()
root.title("ATM software")
create_account_frame = tk.Frame(root)
create_account_frame.pack(padx=10, pady=10)
tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0)
first_name_entry = tk.Entry(create_account_frame)
first_name_entry.grid(row=0, column=1)
tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0)
last_name_entry = tk.Entry(create_account_frame)
last_name_entry.grid(row=1, column=1)
tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0)
dob_entry = tk.Entry(create_account_frame)
dob_entry.grid(row=2, column=1)
tk.Label(create_account_frame, text="Enter address:").grid(row=3, column=0)
address_entry = tk.Entry(create_account_frame)
address_entry.grid(row=3, column=1)
tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0)
debit_card_entry = tk.Entry(create_account_frame)
debit_card_entry.grid(row=4, column=1)
tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0)
pin_entry = tk.Entry(create_account_frame, show="*")
pin_entry.grid(row=5, column=1)
create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account)
create_account_button.grid(row=6, column=0, columnspan=2)
login_frame = tk.Frame(root)
login_frame.pack(padx=10, pady=10)
tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0)
login_debit_card_entry = tk.Entry(login_frame)
login_debit_card_entry.grid(row=0, column=1)
tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0)
login_pin_entry = tk.Entry(login_frame, show="*")
login_pin_entry.grid(row=1, column=1)
tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0)
login_choice_entry = tk.Entry(login_frame)
login_choice_entry.grid(row=2, column=1)
deposit_entry = tk.Entry(login_frame)
deposit_entry.grid(row=3, column=0)
withdraw_entry = tk.Entry(login_frame)
withdraw_entry.grid(row=3, column=1)
login_button = tk.Button(login_frame, text="Login", command=atm.login)
login_button.grid(row=4, column=0, columnspan=2)
status_label = tk.Label(root, text="")
status_label.pack()
root.mainloop()

Let's understand your requirement and let's see how we can achieve it step by step .
We want to accept only the positive values on the screen but currently it is also accepting the negative values . Hence we will fix this to accept values greater than 0 because even the deposit of the $0 does not make any sense .
Hence when the deposit will be less than the 0 then we will give the message to the user on the window saying .
"Deposit Failed (Please enter value greater than 0 ) . Current balance : (Account balance) $"
We should not update the balance in case when the deposit value is less than 0 .
Let's check the code now . In the code the value input for the deposit is taken here :
if choice == "1":
amount = float(deposit_entry.get())
self.accounts[debit_card]["balance"] += amount
status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
Now we want to check the amount
amount = float(deposit_entry.get()) <--- Code to take the value input for the user deposit amount
We want to check the amount now if greater than 0 . Hence what we can do is :
choice = login_choice_entry.get()
if choice == "1":
amount = float(deposit_entry.get())
#check if the amount is greater than 0
if(amount <= 0):
#If less than 0 then give the message and do not update the balance
status_label.config(
text="Deposit Failed (Please enter value greater than 0 only). Current balance : $%.2f" % self.accounts[debit_card]["balance"])
else:
self.accounts[debit_card]["balance"] += amount
status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
Hence from the above code we can see that we have added the if block to check the amount if greater than 0 or not . If yes then update the balance and print the balance on the screen else do not update the balance and show the deposit failed message on the screen .
Step by step
Solved in 2 steps with 8 images

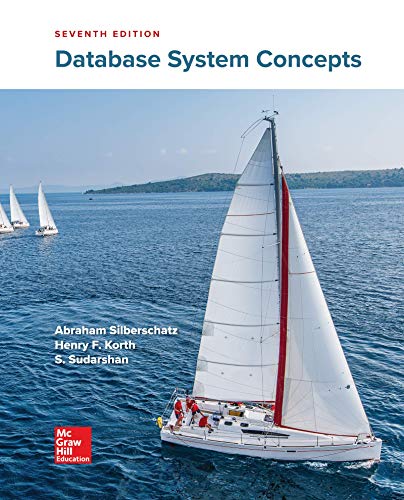
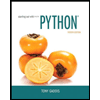
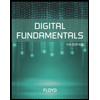
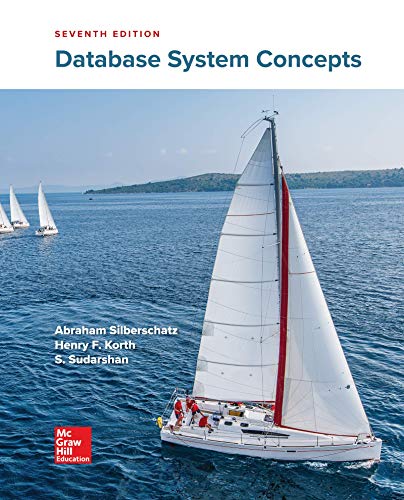
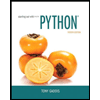
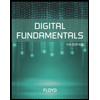
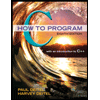
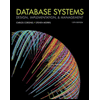
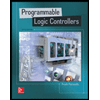