please go in to the detail and explain the purpose and function to each line & function of code listed below. Dsecribe each line.
please go in to the detail and explain the purpose and function to each line & function of code listed below. Dsecribe each line.
#include <stdio.h>//including headers
#include <string.h>
#include <stdlib.h>
struct node{//structure intialization
int data;
struct node *next;
};
#include <assert.h>
typedef struct Info_ {
char name[100];
} Info;
typedef struct Compar_ {
Info info;
struct Compar_* next;
} Compar;
void print_comparisons(Compar* CP)//comparison method
{
assert(CP);
Compar* cur = CP;
Compar* next = cur->next;
for (; next; cur = next, next = next->next) {
if (strcmp(cur->info.name, next->info.name) == 0)
printf("Same name\n");
else
printf("Diff name\n");
}
}
struct node *head, *tail = NULL;
void addNode(int data) {//adding node method
struct node *newNode = (struct node*)malloc(sizeof(struct node));
newNode->data = data;
newNode->next = NULL;
if(head == NULL) {
head = newNode;
tail = newNode;
}
else {
tail->next = newNode;
tail = newNode;
}
}
void removeDuplicate() {//duplicate method definition
struct node *current = head, *index = NULL, *temp = NULL;
if(head == NULL) {
return;
}
else {
while(current != NULL){
temp = current;
index = current->next;
while(index != NULL) {
if(current->data == index->data) {
temp->next = index->next;
}
else {
temp = index;
}
index = index->next;
}
current = current->next;
}
}
}
void display() {
struct node *current = head;
if(head == NULL) {
printf("List is empty \n");
return;
}
while(current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
struct node* findMiddle(struct node* b, struct node* e)
{
if(b == NULL)
return NULL;
struct node* slow = b;
struct node* fast = b->next;
while(fast != e)
{
fast=fast->next;
if(fast != e)
{
slow = slow->next;
fast = fast->next;
}
}
return slow;
};
struct node* binarysearch(int searchItem)
{
struct node* beg = head;
struct node* end = NULL;
do
{
struct node* middle = findMiddle(beg, end);
if(middle == NULL)
return middle;
else if(middle->data == searchItem)
return middle;
else if(middle->data < searchItem)
beg = middle->next;
else
end = middle;
}while(end==NULL || end != beg);
return NULL;
}
int main()//main method
{
addNode(1);
addNode(2);
addNode(3);
addNode(2);
addNode(2);
addNode(4);
addNode(1);
printf("Originals list: \n");
display();
printf("================");
removeDuplicate();
printf("List after removing duplicates: \n");
display();
printf("Search for 3 in the linked list:\n");
struct node* n = binarysearch(3);
if(n==NULL)
{
printf("Item not Found\n");
}
else
{
printf("Item Found\n");
printf("%d\n",n->data);
}
printf("Search for 7 in the linked list:\n");
n = binarysearch(7);
if(n==NULL)
{
printf("Item not Found\n");
}
else
{
printf("Item Found\n");
printf("%d",n->data);
}
printf("================");
Info a; a.name[0] = 'A'; a.name[1] = '\0';
Info b; b.name[0] = 'B'; b.name[1] = '\0';
Info c; c.name[0] = 'B'; c.name[1] = '\0';
Info d; d.name[0] = 'D'; d.name[1] = '\0';
Compar na; na.info = a;
Compar nb; nb.info = b;
Compar nc; nc.info = c;
Compar nd; nd.info = d;
na.next = &nb;
nb.next = &nc;
nc.next = &nd;
nd.next = NULL;
print_comparisons(&na);
return 0;
}

Step by step
Solved in 2 steps with 1 images

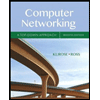
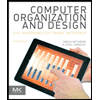
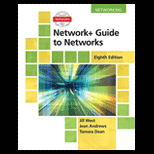
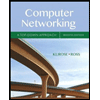
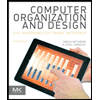
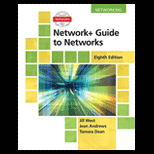
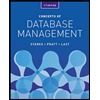
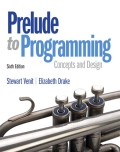
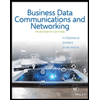