Please help me fix my python program: (see image attached for error message) import random def nucleotide(str1, X): #1. Take two parameters, a nucleotide sequence as a string, and an integer. #2. Check for invalid characters (not ATCG). for i in str1: if i != 'A' or i != 'T' or i != 'G' or i != 'C': sys.exit("Invalid nucleotide") #2. If invalid character is found, exit function #3. Make the entire string lower case str1 = str1.lower() lst = list(str1) #Convert string to list #4. for i in range(0,X): num = len(str1) charPosition = random.randint(0,num-1) #Choose a character position in the string at random charNucleotide = lst[charPosition] #Determine which nucleotide is at that position. randomNucleotide = lst[random.randint(0,3)] #At random, select a different nucleotide and replace the previous nucleotide with the new one. lst[charPosition] = randomNucleotide.upper() listToStr = ''.join(map(str, lst)) #Convert list to string. return listToStr #6. Return the new string #5. Test your code str1 = nucleotide("ATGCGTACTG", 4) print("Orignal nucleotide: ATGCGTACTG") print ("New nucleotide:",str1) This is the guideline in making the code above: I need help to write a function that: 1. Take two parameters, a nucleotide sequence as a string, and an integer. 2. Check for invalid characters (not ATCG). If invalid character is found, exit function 3. Make the entire string lower case 4. For X iterations, where x is the integer parameter - Choose a character position in the string at random - Determine which nucleotide is at that position. At random, select a different nucleotide and replace the previous nucleotide with the new one. Use String splicing, or list() and "".join() -The new nucleotide should be uppercase so that the substitution is clear and obvious. 5. Test your code to make sure this behavior works as intended. Keep the number of iterations low, and add in a reporting mechanism that makes it clear what is happening (what position was chosen, what new nucleotide was chosen, etc). Comment out this out once you're sure the function works as it should. 6. Return the new string
Please help me fix my python program: (see image attached for error message)
import random
def nucleotide(str1, X): #1. Take two parameters, a nucleotide sequence as a string, and an integer.
#2. Check for invalid characters (not ATCG).
for i in str1:
if i != 'A' or i != 'T' or i != 'G' or i != 'C':
sys.exit("Invalid nucleotide") #2. If invalid character is found, exit function
#3. Make the entire string lower case
str1 = str1.lower()
lst = list(str1) #Convert string to list
#4.
for i in range(0,X):
num = len(str1)
charPosition = random.randint(0,num-1) #Choose a character position in the string at random
charNucleotide = lst[charPosition] #Determine which nucleotide is at that position.
randomNucleotide = lst[random.randint(0,3)] #At random, select a different nucleotide and replace the previous nucleotide with the new one.
lst[charPosition] = randomNucleotide.upper()
listToStr = ''.join(map(str, lst)) #Convert list to string.
return listToStr #6. Return the new string
#5. Test your code
str1 = nucleotide("ATGCGTACTG", 4)
print("Orignal nucleotide: ATGCGTACTG")
print ("New nucleotide:",str1)
This is the guideline in making the code above:
I need help to write a function that:
1. Take two parameters, a nucleotide sequence as a string, and an integer.
2. Check for invalid characters (not ATCG). If invalid character is found, exit function
3. Make the entire string lower case
4. For X iterations, where x is the integer parameter
- Choose a character position in the string at random
- Determine which nucleotide is at that position. At random, select a different nucleotide and replace the previous nucleotide with the new one. Use String splicing, or list() and "".join()
-The new nucleotide should be uppercase so that the substitution is clear and obvious.
5. Test your code to make sure this behavior works as intended. Keep the number of iterations low, and add in a reporting
6. Return the new string
![main.py
1 import random
2
3- def nucleotide(str1, X): #1. Take two parameters, a nucleotide sequence as a stri
#2. Check for invalid characters (not ATCG).
for i in str1:
if i != 'A' or i != 'T' or i != 'G' or i != 'C':
sys.exit("Invalid nucleotide") #2. If invalid character is found, exi
#3. Make the entire string Lower case
str1 = str1.lower()
1st =
10
11
12
list(str1) #Convert string to list
13
14
#4.
for i in range(0,X):
num = len(str1)
charPosition = random.randint(0,num-1) #Choose a character position in th
charNucleotide = 1st[charPosition] #Determine which nucleotide is at tho
15
16
17
18
19
20
randomNucleotide = 1st[random.randint(0,3)] #At random, select a differe
21
22
input
File "main.py", line 8, in nucleotide
sys.exit("Invalid nucleotide") #2. If invalid character is found, exit function
NameError: name 'sys' is not defined
... Program finished with exit code 0
Press ENTER to exit console.I
LO N](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9b1b483a-fbb9-4e01-adc3-918d57c456ae%2F1089ca0e-387c-4ab6-b010-ce362d4c0de9%2Fktn4ymq_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

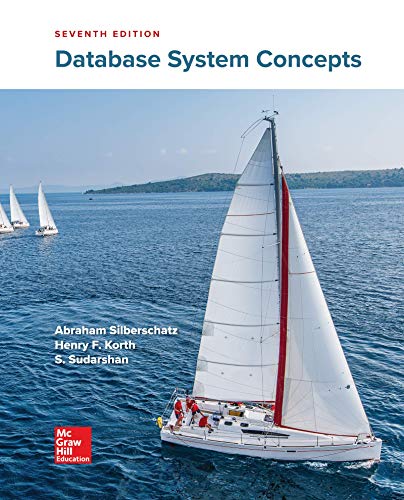
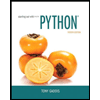
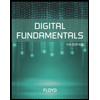
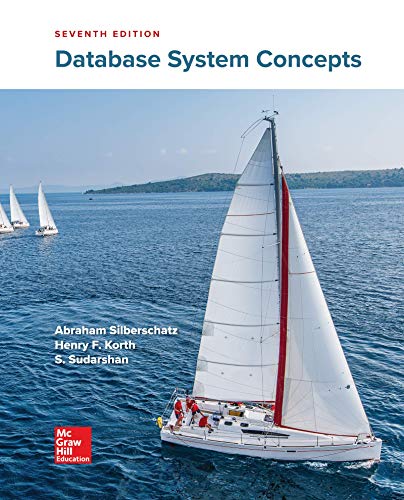
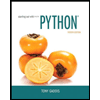
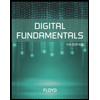
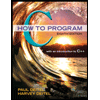
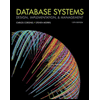
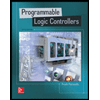