Please help me fix the errors in this java program. I can’t get the program to work. There are two classes AnimatedBall and BouncingBall Class: AnimatedBall Import java.swing.*; import java.awt.*; import java.awt.event.*; public class AnimatedBall extends JFrame implements ActionListener { private Button btnBounce; // declare a button to play private TextField txtSpeed; // declare a text field to enter number private Label lblDelay; private Panel controls; // generic panel for controls private BouncingBall display; // drawing panel for ball Container frame; public AnimatedBall () { // Set up the controls on the applet frame = getContentPane(); btnBounce = new Button ("Bounce Ball"); //create the objects txtSpeed = new TextField("10000", 10); lblDelay = new Label("Enter Delay"); display = new BouncingBall();//create the panel objects controls = new Panel(); setLayout(new BorderLayout()); // set the frame layout controls.add (btnBounce); // add controls to panel controls.add (lblDelay); controls.add (txtSpeed); frame.add(controls, BorderLayout.NORTH); // add panel to top frame.add(display, BorderLayout.CENTER); //add panel to center btnBounce.addActionListener(this); //add action listener setSize(450,500); setVisible(true); } // init method public void paint (Graphics g) { // paint no longer used // we are not painting on the JFrame - remove } // paint method public void actionPerformed (ActionEvent e) { long speed; speed = Long.parseLong(txtSpeed.getText()); //read text field if (e.getSource() == btnBounce) { display.draw(speed); } } // action method } // AnimatedBall class The BouncingBall class below is used by the AnimatedBall class to draw the graphics panel. Class: BouncingBall import java.awt.*; public class BouncingBall extends Panel { private long delay; // Overridden paint method for painting the BouncingBall panel public void paint( Graphics g ) { super.paint( g ); // calls paint method from Panel. int radius = (getSize().width/10)/2; int Z = getSize().height/2 - radius; x = (int)(Math.random()*(getSize().width)) + radius; int dy = 1; for (int count = 0; count < 1000 ;count++ ) { g.setColor(getBackground()); //get the panel background color to erase g.drawOval(X, Y, 2*radius, 2*radius); if (Y<=0 || Y>=(getSize().height)- 2*radius) { dy = -dy; } Y += dy; g.setColor( Color.BLUE ); g.fillOval(X, Y, 2*radius, 2*radius); try { Thread.sleep(delay); } catch (InterruptedException ex) { } } } // method to set delay value and draw the BouncingBall Panel public void draw(long d) { this.delay = d; repaint(); } } // end class BouncingBall
Please help me fix the errors in this java program. I can’t get the program to work. There are two classes AnimatedBall and BouncingBall
Class: AnimatedBall
Import java.swing.*;
import java.awt.*;
import java.awt.event.*;
public class AnimatedBall extends JFrame implements ActionListener
{
private Button btnBounce; // declare a button to play
private TextField txtSpeed; // declare a text field to enter
number
private Label lblDelay;
private Panel controls; // generic panel for controls
private BouncingBall display; // drawing panel for ball
Container frame;
public AnimatedBall ()
{
// Set up the controls on the applet
frame = getContentPane();
btnBounce = new Button ("Bounce Ball");
//create the objects
txtSpeed = new TextField("10000", 10);
lblDelay = new Label("Enter Delay");
display = new BouncingBall();//create the panel objects
controls = new Panel();
setLayout(new BorderLayout()); // set the frame layout
controls.add (btnBounce); // add controls to panel
controls.add (lblDelay);
controls.add (txtSpeed);
frame.add(controls, BorderLayout.NORTH);
// add panel to top
frame.add(display, BorderLayout.CENTER);
//add panel to center
btnBounce.addActionListener(this);
//add action listener
setSize(450,500);
setVisible(true);
} // init method
public void paint (Graphics g)
{
// paint no longer used
// we are not painting on the JFrame - remove
} // paint method
public void actionPerformed (ActionEvent e)
{
long speed;
speed = Long.parseLong(txtSpeed.getText());
//read text field
if (e.getSource() == btnBounce)
{
display.draw(speed);
}
} // action method
} // AnimatedBall class
The BouncingBall class below is used by the AnimatedBall class to draw the graphics
panel.
Class: BouncingBall
import java.awt.*;
public class BouncingBall extends Panel
{
private long delay;
// Overridden paint method for painting the BouncingBall panel
public void paint( Graphics g )
{
super.paint( g ); // calls paint method from Panel.
int radius = (getSize().width/10)/2;
int Z = getSize().height/2 - radius;
x = (int)(Math.random()*(getSize().width)) + radius;
int dy = 1;
for (int count = 0; count < 1000 ;count++ )
{
g.setColor(getBackground());
//get the panel background color to erase
g.drawOval(X, Y, 2*radius, 2*radius);
if (Y<=0 || Y>=(getSize().height)- 2*radius)
{
dy = -dy;
}
Y += dy;
g.setColor( Color.BLUE );
g.fillOval(X, Y, 2*radius, 2*radius);
try
{
Thread.sleep(delay);
}
catch (InterruptedException ex)
{
}
}
}
// method to set delay value and draw the BouncingBall Panel
public void draw(long d)
{
this.delay = d;
repaint();
}
} // end class BouncingBall

Step by step
Solved in 2 steps

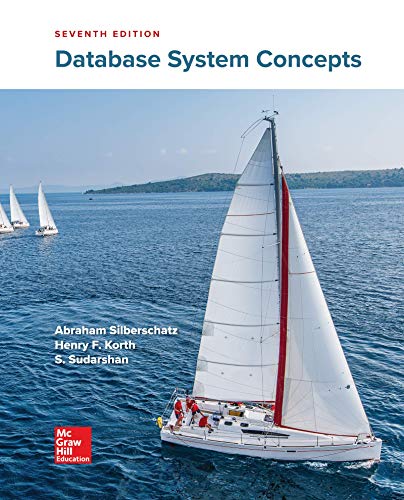
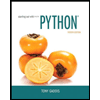
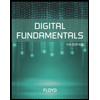
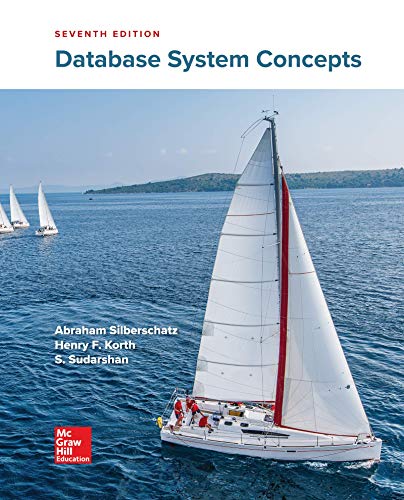
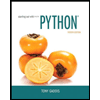
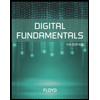
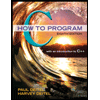
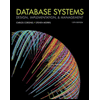
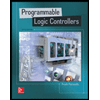