Please help me with my code in python. Can you change the start_tag and end_tag to strip. Since we have not discussed anything about tag yet. Thank you def read_data(): with open("simple.xml", "r") as file: content = file.read() return content def extract_data(tag, string): data = [] start_tag = f"<{tag}>" end_tag = f"" while start_tag in string: start_index = string.find(start_tag) + len(start_tag) end_index = string.find(end_tag) value = string[start_index:end_index] data.append(value) string = string[end_index + len(end_tag):] return data def get_names(string): names = extract_data("name", string) return names def get_calories(string): calories = extract_data("calories", string) return calories def get_descriptions(string): descriptions = extract_data("description", string) return descriptions def get_prices(string): prices = extract_data("price", string) return prices def display_menu(names, descriptions, calories, prices): for i in range(len(names)): print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}") def display_stats(names, calories, prices): total_items = len(names) average_calories = sum(map(int, calories)) / total_items average_price = sum([float(price[1:]) for price in prices]) / total_items print(f"\n{total_items} items - {average_calories:.1f} average calories - ${average_price:.2f} average price") def main(): content = read_data() names = get_names(content) descriptions = get_descriptions(content) calories = get_calories(content) prices = get_prices(content) display_menu(names, descriptions, calories, prices) display_stats(names, calories, prices) main()
Please help me with my code in python. Can you change the start_tag and end_tag to strip. Since we have not discussed anything about tag yet. Thank you
def read_data():
with open("simple.xml", "r") as file:
content = file.read()
return content
def extract_data(tag, string):
data = []
start_tag = f"<{tag}>"
end_tag = f"</{tag}>"
while start_tag in string:
start_index = string.find(start_tag) + len(start_tag)
end_index = string.find(end_tag)
value = string[start_index:end_index]
data.append(value)
string = string[end_index + len(end_tag):]
return data
def get_names(string):
names = extract_data("name", string)
return names
def get_calories(string):
calories = extract_data("calories", string)
return calories
def get_descriptions(string):
descriptions = extract_data("description", string)
return descriptions
def get_prices(string):
prices = extract_data("price", string)
return prices
def display_menu(names, descriptions, calories, prices):
for i in range(len(names)):
print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}")
def display_stats(names, calories, prices):
total_items = len(names)
average_calories = sum(map(int, calories)) / total_items
average_price = sum([float(price[1:]) for price in prices]) / total_items
print(f"\n{total_items} items - {average_calories:.1f} average calories - ${average_price:.2f} average price")
def main():
content = read_data()
names = get_names(content)
descriptions = get_descriptions(content)
calories = get_calories(content)
prices = get_prices(content)
display_menu(names, descriptions, calories, prices)
display_stats(names, calories, prices)
main()

Step by step
Solved in 3 steps

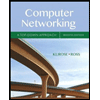
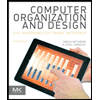
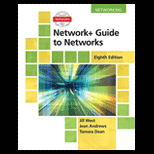
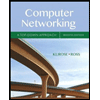
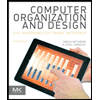
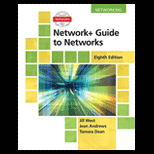
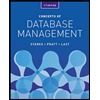
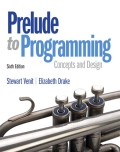
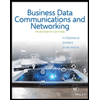