calFromCarb (double) calFromFat (double) calFromProtein (double) Use the data types in parentheses for the fields. Note that you need to use the proper C++ syntax for the fields. Each field should have a co
PLease! IN C++
Write A Class
Declare a class/struct named NutritionData that contains these private fields
foodName (string)
servingSize (int)
calFromCarb (double)
calFromFat (double)
calFromProtein (double)
Use the data types in parentheses for the fields. Note that you need to use the proper C++ syntax for the fields.
Each field should have a comment documenting what it is for. Place one comment above each field.
Add the public default constructor. Read the textbook for the syntax of the default constructor. Write the body of the default constructor inline. The default constructor initializes the fields so that the food name is an empty string and all other fields are 0 for int and 0.0 for double.
Add public mutator member functions to set the fields. One mutator member function for each field. Each mutator member function's name should begin with the word 'set' followed by the field name with the first letter changed to uppercase. Each member function should have a comment above the declaration describing its purpose. Write the bodies of the mutator functions inline.
Add public accessor member functions to return the fields. One accessor member function for each field. Each member function should begin with the word 'get' followed by the field name with the first letter changed to uppercase. You must place the keyword const to indicate accessor function (look up the textbook or some other resource for details). Each member function should have a comment above the declaration describing its purpose. Write the bodies of these accessor functions inline.
Add a special public accessor member function named getCaloriesPerServing that returns the total calories from carb, protein and fat. Again add the const keyword and place the comment above the declaration of this member function. Write the body outside of the class.
Write the definition of getCaloriesPerServing outside of the class declaration.
Regarding the block comment for the function, place it inside the class above the member function declaration.
Write the declaration of the function named printNutritionData. The function receives two parameters, a pointer to const NutritionData and an int for the number of NutritionData, and returns void. The parameters represent an array of NutritionData objects.
Write the main function as follows:
The main function gets nutrition data for a list of foods from a file named 'nutri.txt' and store them in an array of NutritionData objects. It then calls printNutritionData to print the nutrition data of the foods. You can download the file from nutri.txt download and place it in the same folder/directory as your executable program of this lab.
The first line of the file contains the number of nutrition data stored in the file. Get that number and use that number to dynamically create an array of NutritionData object. Save the address in a pointer variable. Do not change this pointer until the memory is recycled through the delete operation.
For each object in the array, populate the object with data from the input file. The data for each food are stored in the file as a comma delimited string in the file. They are food name, serving size (an integer), calories from carb, calories from fat, and calories from protein. Here is an example for one food.
Bread pita whole wheat, 64, 134, 14, 22.6
Use the mutator functions to set the nutrition data for each object in the array.
Print the nutrition data of foods on the screen by calling printNutritionData.
Recycle the allocated array using the delete operator.
Reset the pointer to nullptr. (Don't use NULL, we have a C++ keyword named nullptr.)
Write the block comment and the definition of the function printNutritionData.
Use a loop, cout, and the accessor functions to print the nutrition data.
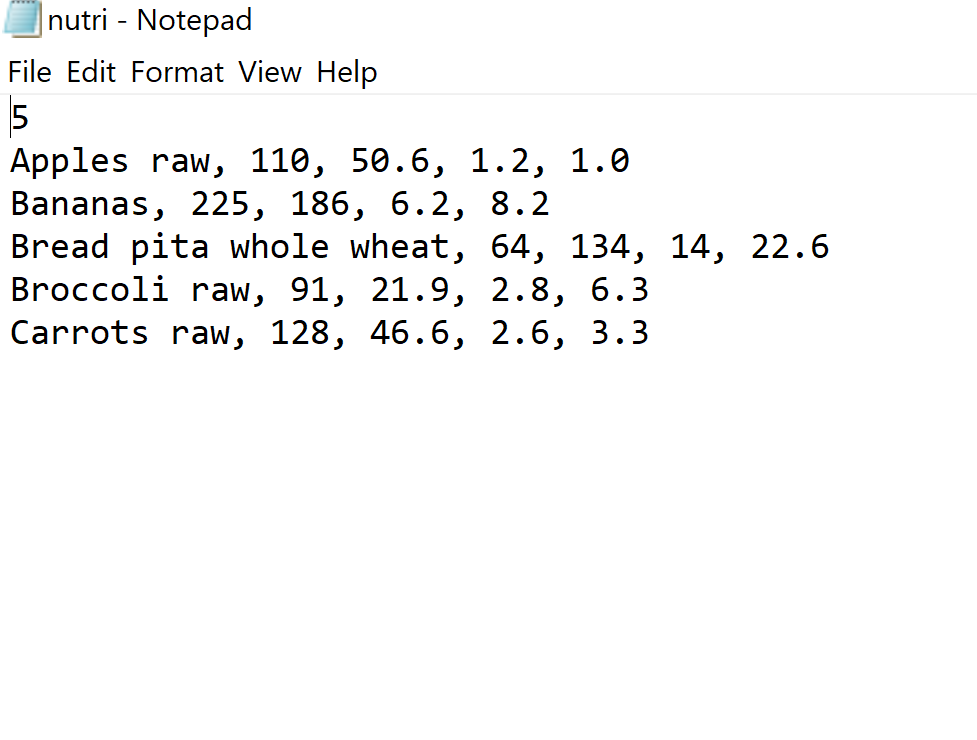
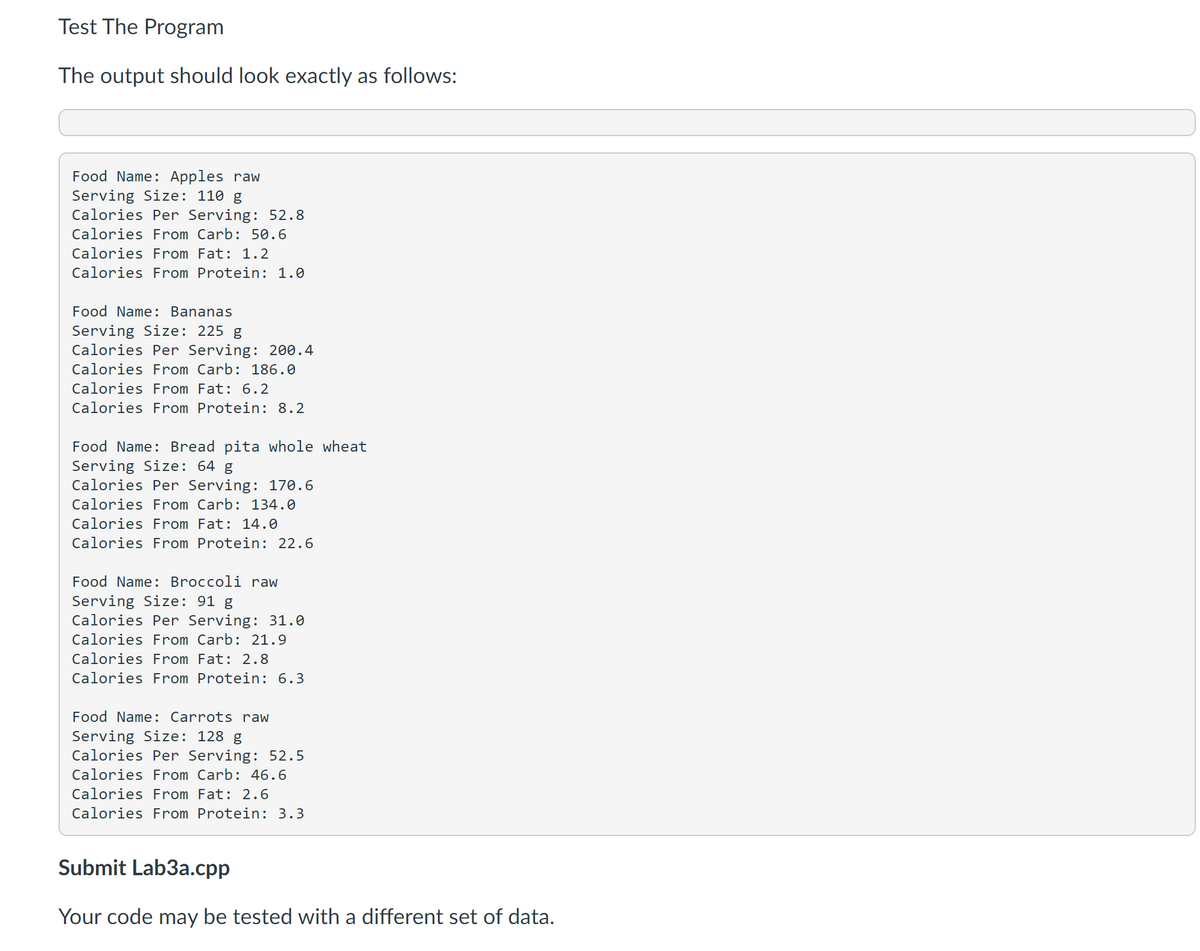

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

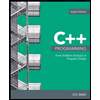
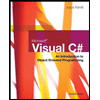
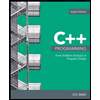
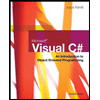