Please in java , I cant get the output for t totalsalesforweek // b. avgsalesforweek // c. totalsalesforallweeks // d. averageweeklysales // e. weekwithhighestsaleamt // f. weekwithlowestsaleamt // analyzeresults //call a through f Write a program that opens the salesdat.txt file and processes it contents. The program should display the following per store: The total sales for each week. (Should print 5 values - one for each week). The average daily sales for each week. (Should print 5 values - one for each week). The total sales for all the weeks. (Should print 1 value) The average weekly sales. (Should print 1 value) The week with the highest amount in sales. (Should print 1 week #) The week with the lowest amount in sales. (Should print 1 week #) All Values (Total Sales, Average Daily Sales for Each Week, Total Sales for all Weeks, Average Weekly Sales, Highest Amount in Sales, Lowest Amount in Sales) The file contains the dollars amount of sales that a retail store made each day for a number of weeks. Each line in the file contains thirty five numbers, which are sales numbers for five weeks. The number are separated by space. Each line in the file represents a separate store. Please make sure that you: a. Add a class diagram with your submission. b. Add comments to your code in FileIO class. c. Make sure you adequately test your code. d. Provide a user-friendly interface (Console based). The following is source code public class Driver { public static void main(String[] args) { // TODO Auto-generated method stub FileIO a1 = new FileIO("Z:\\JavaPrograms2\\assignment336b\\src\\Salesdat.txt"); Franchise f = a1.readData(); } } ------------------------------------------ import java.io.*; import java.util.*; public class FileIO { private String fname = null; private boolean DEBUG = true; public FileIO(String fname) { this.fname = fname; } public Franchise readData() { Franchise a1 = null; int counter = 0; try { FileReader file = new FileReader(fname); BufferedReader buff = new BufferedReader(file); String temp; boolean eof = false; while (!eof) { String line = buff.readLine(); counter++; if (line == null) eof = true; else { if (DEBUG) System.out.println("Reading" + line); if (counter == 1) { temp = line; a1 = new Franchise(Integer.parseInt(temp)); if (DEBUG) System.out.println("d " + a1.numberofstores()); } if (counter == 2) ; if (counter > 2) { int x = buildStore(a1, (counter-3), line); if (DEBUG) System.out.println("Reading Store # "+(counter-2)+" Number of weeks read = " + x); if (DEBUG) { System.out.println("Data read:"); a1.getStores(counter-3).printdata(); } } } } buff.close(); } catch (Exception e) { System.out.println("Error -- " + e.toString()); } return a1; } public int buildStore(Franchise a1, int counter, String temp) { Store tstore = new Store(); String s1 = ""; float sale = 0.0f; int week = 0; int day = 0; StringTokenizer st = new StringTokenizer(temp); while (st.hasMoreTokens()) { for(day=0;day<7;day++) { s1 = st.nextToken(); sale = Float.parseFloat(s1); tstore.setsaleforweekdayintersection(week, day, sale); } week++; } a1.setStores(tstore, counter); return week; } } --------------------- public class Franchise { private Store stores[]; public Franchise(int num) { stores = new Store[num]; } public Store getStores(int i) { return stores[i]; } public void setStores(Store stores, int i) { this.stores[i] = stores; } public int numberofstores() { return stores.length; } } ---------------------- public class Store { private float salesbyweek[][]; Store() { salesbyweek = new float[5][7]; } // getter and setters // setsaleforweekdayintersection(int week, int day, float sale) public void setsaleforweekdayintersection(int week, int day, float sale) { salesbyweek[week][day] = sale; } public void printdata() { for (int i = 0; i < 5; i++) { for (int j = 0; j < 7; j++) { System.out.print(salesbyweek[i][j] + " "); } System.out.println(""); } } // float [] getsalesforentireweek(int week) // float getsaleforweekdayintersection(int week, int day) // businessmethod // a. totalsalesforweek // b. avgsalesforweek // c. totalsalesforallweeks // d. averageweeklysales // e. weekwithhighestsaleamt // f. weekwithlowestsaleamt // analyzeresults //call a through f // print() }
Please in java , I cant get the output for t totalsalesforweek
// b. avgsalesforweek
// c. totalsalesforallweeks
// d. averageweeklysales
// e. weekwithhighestsaleamt
// f. weekwithlowestsaleamt
// analyzeresults
//call a through f
Write a program that opens the salesdat.txt file and processes it contents. The program should display the following per store:
The total sales for each week. (Should print 5 values - one for each week).
The average daily sales for each week. (Should print 5 values - one for each week).
The total sales for all the weeks. (Should print 1 value)
The average weekly sales. (Should print 1 value)
The week with the highest amount in sales. (Should print 1 week #)
The week with the lowest amount in sales. (Should print 1 week #)
All Values (Total Sales, Average Daily Sales for Each Week, Total Sales for all Weeks, Average Weekly Sales, Highest Amount in Sales, Lowest Amount in Sales)
The file contains the dollars amount of sales that a retail store made each day for a number of weeks. Each line in the file contains thirty five numbers, which are sales numbers for five weeks. The number are separated by space. Each line in the file represents a separate store.
Please make sure that you:
a. Add a class diagram with your submission.
b. Add comments to your code in FileIO class.
c. Make sure you adequately test your code.
d. Provide a user-friendly interface (Console based).
The following is source code
public class Driver {
public static void main(String[] args) {
// TODO Auto-generated method stub
FileIO a1 = new FileIO("Z:\\JavaPrograms2\\assignment336b\\src\\Salesdat.txt");
Franchise f = a1.readData();
}
}
------------------------------------------
import java.io.*;
import java.util.*;
public class FileIO {
private String fname = null;
private boolean DEBUG = true;
public FileIO(String fname) {
this.fname = fname;
}
public Franchise readData() {
Franchise a1 = null;
int counter = 0;
try {
FileReader file = new FileReader(fname);
BufferedReader buff = new BufferedReader(file);
String temp;
boolean eof = false;
while (!eof) {
String line = buff.readLine();
counter++;
if (line == null)
eof = true;
else {
if (DEBUG)
System.out.println("Reading" + line);
if (counter == 1) {
temp = line;
a1 = new Franchise(Integer.parseInt(temp));
if (DEBUG)
System.out.println("d " + a1.numberofstores());
}
if (counter == 2)
;
if (counter > 2) {
int x = buildStore(a1, (counter-3), line);
if (DEBUG)
System.out.println("Reading Store # "+(counter-2)+" Number of weeks read = " + x);
if (DEBUG)
{
System.out.println("Data read:");
a1.getStores(counter-3).printdata();
}
}
}
}
buff.close();
} catch (Exception e) {
System.out.println("Error -- " + e.toString());
}
return a1;
}
public int buildStore(Franchise a1, int counter, String temp) {
Store tstore = new Store();
String s1 = "";
float sale = 0.0f;
int week = 0;
int day = 0;
StringTokenizer st = new StringTokenizer(temp);
while (st.hasMoreTokens()) {
for(day=0;day<7;day++)
{
s1 = st.nextToken();
sale = Float.parseFloat(s1);
tstore.setsaleforweekdayintersection(week, day, sale);
}
week++;
}
a1.setStores(tstore, counter);
return week;
}
}
---------------------
public class Franchise {
private Store stores[];
public Franchise(int num) {
stores = new Store[num];
}
public Store getStores(int i) {
return stores[i];
}
public void setStores(Store stores, int i) {
this.stores[i] = stores;
}
public int numberofstores()
{
return stores.length;
}
}
----------------------
public class Store {
private float salesbyweek[][];
Store() {
salesbyweek = new float[5][7];
}
// getter and setters
// setsaleforweekdayintersection(int week, int day, float sale)
public void setsaleforweekdayintersection(int week, int day, float sale) {
salesbyweek[week][day] = sale;
}
public void printdata() {
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 7; j++)
{
System.out.print(salesbyweek[i][j] + " ");
}
System.out.println("");
}
}
// float [] getsalesforentireweek(int week)
// float getsaleforweekdayintersection(int week, int day)
// businessmethod
// a. totalsalesforweek
// b. avgsalesforweek
// c. totalsalesforallweeks
// d. averageweeklysales
// e. weekwithhighestsaleamt
// f. weekwithlowestsaleamt
// analyzeresults //call a through f
// print()
}

Step by step
Solved in 3 steps

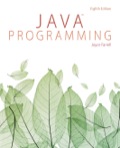
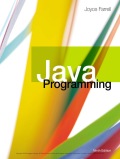
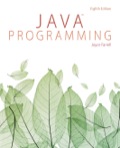
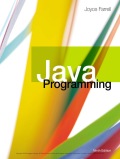