Please modify the code below for "Current Date" ********************************************************************** from pandas_datareader import data as pdr import yfinance as yfin # Work around until # pandas_datareader is fixed. import datetime import pandas as pd import matplotlib.pyplot as plt import numpy as np # Show all columns. pd.set_option('display.max_columns', None) pd.set_option('display.width', 1000) def getStock(stk, numDays): print(stk) # Only gets up until day before during # trading hours dt = datetime.date.today() # For some reason, must add 1 day to get current stock prices # during trade hours. (Prices are about 15 min behind actual prices.) dtNow = dt + datetime.timedelta(days=1) dtNowStr = dtNow.strftime("%Y-%m-%d") dtPast = dt + datetime.timedelta(days=-numDays) dtPastStr = dtPast.strftime("%Y-%m-%d") yfin.pdr_override() df = pdr.get_data_yahoo(stk, start=dtPastStr, end=dtNowStr) return df ################################################################## # CONFIGURATION SECTION NUM_DAYS = 1200 STOCK_SYMBOL = 'AAPL' NUM_TIME_STEPS = 2 TEST_DAYS = 7 ################################################################## df = getStock(STOCK_SYMBOL, NUM_DAYS) def backShiftColumns(df, colName): # Create time step columns for 'Open' price. for i in range(1, NUM_TIME_STEPS + 1): newColumnName = colName + 't-' + str(i) df[newColumnName] = df[colName].shift(periods=i) return df dfBack = backShiftColumns(df, 'Open') dfBack = backShiftColumns(dfBack, 'Close') dfBack = dfBack.dropna() # Remove nulls after back-shifting. y = dfBack[['Open']] X = dfBack[['Opent-1', 'Closet-1', 'Closet-2']] # Add intercept for OLS regression. import statsmodels.api as sm X = sm.add_constant(X) # Split into test and train sets. The test data must be # the latest data range. lenData = len(X) X_train = X[0:lenData-TEST_DAYS] y_train = y[0:lenData-TEST_DAYS] X_test = X[lenData-TEST_DAYS:] y_test = y[lenData-TEST_DAYS:] print(X_test) ################ SECTION B # Model and make predictions. model = sm.OLS(y_train, X_train).fit() print(model.summary()) predictions = model.predict(X_test) ################ SECTION C # Show RMSE and plot the data. from sklearn import metrics print('Root Mean Squared Error:', np.sqrt(metrics.mean_squared_error(y_test, predictions))) plt.plot(y_test.index, predictions, label='Predicted', marker='o', color='orange') plt.plot(y_test.index, y_test, label='Actual', marker='o', color='blue') plt.xticks(rotation=45) plt.legend(loc='best') plt.show()
Please modify the code below for "Current Date" ********************************************************************** from pandas_datareader import data as pdr import yfinance as yfin # Work around until # pandas_datareader is fixed. import datetime import pandas as pd import matplotlib.pyplot as plt import numpy as np # Show all columns. pd.set_option('display.max_columns', None) pd.set_option('display.width', 1000) def getStock(stk, numDays): print(stk) # Only gets up until day before during # trading hours dt = datetime.date.today() # For some reason, must add 1 day to get current stock prices # during trade hours. (Prices are about 15 min behind actual prices.) dtNow = dt + datetime.timedelta(days=1) dtNowStr = dtNow.strftime("%Y-%m-%d") dtPast = dt + datetime.timedelta(days=-numDays) dtPastStr = dtPast.strftime("%Y-%m-%d") yfin.pdr_override() df = pdr.get_data_yahoo(stk, start=dtPastStr, end=dtNowStr) return df ################################################################## # CONFIGURATION SECTION NUM_DAYS = 1200 STOCK_SYMBOL = 'AAPL' NUM_TIME_STEPS = 2 TEST_DAYS = 7 ################################################################## df = getStock(STOCK_SYMBOL, NUM_DAYS) def backShiftColumns(df, colName): # Create time step columns for 'Open' price. for i in range(1, NUM_TIME_STEPS + 1): newColumnName = colName + 't-' + str(i) df[newColumnName] = df[colName].shift(periods=i) return df dfBack = backShiftColumns(df, 'Open') dfBack = backShiftColumns(dfBack, 'Close') dfBack = dfBack.dropna() # Remove nulls after back-shifting. y = dfBack[['Open']] X = dfBack[['Opent-1', 'Closet-1', 'Closet-2']] # Add intercept for OLS regression. import statsmodels.api as sm X = sm.add_constant(X) # Split into test and train sets. The test data must be # the latest data range. lenData = len(X) X_train = X[0:lenData-TEST_DAYS] y_train = y[0:lenData-TEST_DAYS] X_test = X[lenData-TEST_DAYS:] y_test = y[lenData-TEST_DAYS:] print(X_test) ################ SECTION B # Model and make predictions. model = sm.OLS(y_train, X_train).fit() print(model.summary()) predictions = model.predict(X_test) ################ SECTION C # Show RMSE and plot the data. from sklearn import metrics print('Root Mean Squared Error:', np.sqrt(metrics.mean_squared_error(y_test, predictions))) plt.plot(y_test.index, predictions, label='Predicted', marker='o', color='orange') plt.plot(y_test.index, y_test, label='Actual', marker='o', color='blue') plt.xticks(rotation=45) plt.legend(loc='best') plt.show()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please modify the code below for "Current Date"
**********************************************************************
from pandas_datareader import data as pdr
import yfinance as yfin # Work around until
# pandas_datareader is fixed.
import datetime
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
# Show all columns.
pd.set_option('display.max_columns', None)
pd.set_option('display.width', 1000)
def getStock(stk, numDays):
print(stk)
# Only gets up until day before during
# trading hours
dt = datetime.date.today()
# For some reason, must add 1 day to get current stock prices
# during trade hours. (Prices are about 15 min behind actual prices.)
dtNow = dt + datetime.timedelta(days=1)
dtNowStr = dtNow.strftime("%Y-%m-%d")
dtPast = dt + datetime.timedelta(days=-numDays)
dtPastStr = dtPast.strftime("%Y-%m-%d")
yfin.pdr_override()
df = pdr.get_data_yahoo(stk, start=dtPastStr, end=dtNowStr)
return df
##################################################################
# CONFIGURATION SECTION
NUM_DAYS = 1200
STOCK_SYMBOL = 'AAPL'
NUM_TIME_STEPS = 2
TEST_DAYS = 7
##################################################################
df = getStock(STOCK_SYMBOL, NUM_DAYS)
def backShiftColumns(df, colName):
# Create time step columns for 'Open' price.
for i in range(1, NUM_TIME_STEPS + 1):
newColumnName = colName + 't-' + str(i)
df[newColumnName] = df[colName].shift(periods=i)
return df
dfBack = backShiftColumns(df, 'Open')
dfBack = backShiftColumns(dfBack, 'Close')
dfBack = dfBack.dropna() # Remove nulls after back-shifting.
y = dfBack[['Open']]
X = dfBack[['Opent-1', 'Closet-1', 'Closet-2']]
# Add intercept for OLS regression.
import statsmodels.api as sm
X = sm.add_constant(X)
# Split into test and train sets. The test data must be
# the latest data range.
lenData = len(X)
X_train = X[0:lenData-TEST_DAYS]
y_train = y[0:lenData-TEST_DAYS]
X_test = X[lenData-TEST_DAYS:]
y_test = y[lenData-TEST_DAYS:]
print(X_test)
################ SECTION B
# Model and make predictions.
model = sm.OLS(y_train, X_train).fit()
print(model.summary())
predictions = model.predict(X_test)
################ SECTION C
# Show RMSE and plot the data.
from sklearn import metrics
print('Root Mean Squared Error:',
np.sqrt(metrics.mean_squared_error(y_test, predictions)))
plt.plot(y_test.index, predictions, label='Predicted', marker='o', color='orange')
plt.plot(y_test.index, y_test, label='Actual', marker='o', color='blue')
plt.xticks(rotation=45)
plt.legend(loc='best')
plt.show()
import yfinance as yfin # Work around until
# pandas_datareader is fixed.
import datetime
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
# Show all columns.
pd.set_option('display.max_columns', None)
pd.set_option('display.width', 1000)
def getStock(stk, numDays):
print(stk)
# Only gets up until day before during
# trading hours
dt = datetime.date.today()
# For some reason, must add 1 day to get current stock prices
# during trade hours. (Prices are about 15 min behind actual prices.)
dtNow = dt + datetime.timedelta(days=1)
dtNowStr = dtNow.strftime("%Y-%m-%d")
dtPast = dt + datetime.timedelta(days=-numDays)
dtPastStr = dtPast.strftime("%Y-%m-%d")
yfin.pdr_override()
df = pdr.get_data_yahoo(stk, start=dtPastStr, end=dtNowStr)
return df
##################################################################
# CONFIGURATION SECTION
NUM_DAYS = 1200
STOCK_SYMBOL = 'AAPL'
NUM_TIME_STEPS = 2
TEST_DAYS = 7
##################################################################
df = getStock(STOCK_SYMBOL, NUM_DAYS)
def backShiftColumns(df, colName):
# Create time step columns for 'Open' price.
for i in range(1, NUM_TIME_STEPS + 1):
newColumnName = colName + 't-' + str(i)
df[newColumnName] = df[colName].shift(periods=i)
return df
dfBack = backShiftColumns(df, 'Open')
dfBack = backShiftColumns(dfBack, 'Close')
dfBack = dfBack.dropna() # Remove nulls after back-shifting.
y = dfBack[['Open']]
X = dfBack[['Opent-1', 'Closet-1', 'Closet-2']]
# Add intercept for OLS regression.
import statsmodels.api as sm
X = sm.add_constant(X)
# Split into test and train sets. The test data must be
# the latest data range.
lenData = len(X)
X_train = X[0:lenData-TEST_DAYS]
y_train = y[0:lenData-TEST_DAYS]
X_test = X[lenData-TEST_DAYS:]
y_test = y[lenData-TEST_DAYS:]
print(X_test)
################ SECTION B
# Model and make predictions.
model = sm.OLS(y_train, X_train).fit()
print(model.summary())
predictions = model.predict(X_test)
################ SECTION C
# Show RMSE and plot the data.
from sklearn import metrics
print('Root Mean Squared Error:',
np.sqrt(metrics.mean_squared_error(y_test, predictions)))
plt.plot(y_test.index, predictions, label='Predicted', marker='o', color='orange')
plt.plot(y_test.index, y_test, label='Actual', marker='o', color='blue')
plt.xticks(rotation=45)
plt.legend(loc='best')
plt.show()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
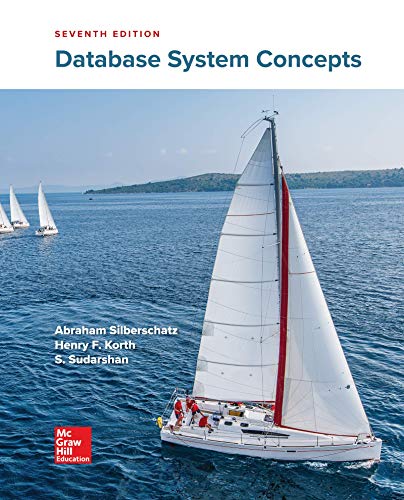
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
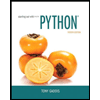
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
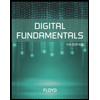
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
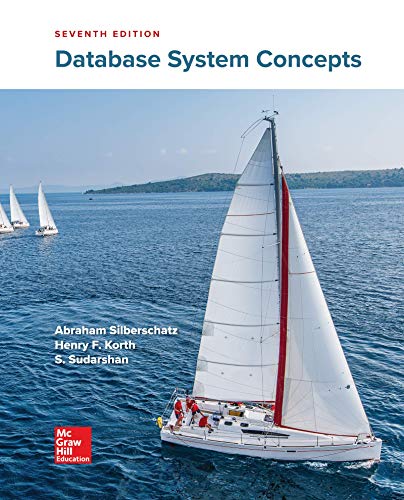
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
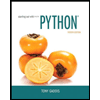
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
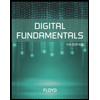
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
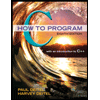
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
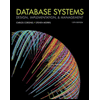
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
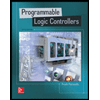
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education