Code in C : Data Structures , Linked List Objective: Implement programmer defined-data types with linked lists. Project name: SetList Make sure that the functions are tested in your main function. In case you can not implement all functions, ensure that there are no compile-time errors and that there are no run-time errors for the completed functions. A set of integers may be implemented using a linked list. Implement the following functions given the definition: typedef struct node* nodeptr; typedef struct node{ int data; nodeptr next; }Node; typedef Node* Set; Set initialize(); - simply initialize to NULL void display(Set s); - display on the screen all valid elements of the list Set add(Set s, elem); - simply store elem in the list int contains(Set s, int elem); - search the array elements for the value elem Set getUnion(Set s1, Set s2); - get the union of s1 and s2 and return - x is an element of s1 union s2 if x is an element of s1 or x is an element of s2 Set intersection(Set s1, Set s2); - get the intersection of s1 and s2 and return - x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2 Set difference(Set s1, Set s2); - get the difference of s1 and s2 and return - x is an element of s1 - s2 if x is an element of s1 and x is not an element of s2 Set symmetricdifference(Set s1, Set s2); - get the symmetric difference of s1 and s2 and return - x is an element of s1 - s2 if x is an element of s1 and x is not an element of s2 and vice versa int subset(Set s1, Set s2); - s1 is a subset of s2 if all elements of s1 are in s2 int disjoint(Set s1, Set s2); - two sets are disjoint if the intersection is empty int equal(Set s1, Set s2); - two sets are equal if they have exactly the same elements
Code in C : Data Structures , Linked List
Objective: Implement programmer defined-data types with linked lists.
Project name: SetList
Make sure that the functions are tested in your main function.
In case you can not implement all functions, ensure that there are no compile-time errors and
that there are no run-time errors for the completed functions.
A set of integers may be implemented using a linked list.
Implement the following functions given the definition:
typedef struct node* nodeptr;
typedef struct node{
int data;
nodeptr next;
}Node;
typedef Node* Set;
Set initialize();
- simply initialize to NULL
void display(Set s);
- display on the screen all valid elements of the list
Set add(Set s, elem);
- simply store elem in the list
int contains(Set s, int elem);
- search the array elements for the value elem
Set getUnion(Set s1, Set s2);
- get the union of s1 and s2 and return
- x is an element of s1 union s2 if x is an element of s1 or x is an element of s2
Set intersection(Set s1, Set s2);
- get the intersection of s1 and s2 and return
- x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2
Set difference(Set s1, Set s2);
- get the difference of s1 and s2 and return
- x is an element of s1 - s2 if x is an element of s1 and x is not an element of s2
Set symmetricdifference(Set s1, Set s2);
- get the symmetric difference of s1 and s2 and return
- x is an element of s1 - s2 if x is an element of s1 and x is not an element of s2 and vice
versa
int subset(Set s1, Set s2);
- s1 is a subset of s2 if all elements of s1 are in s2
int disjoint(Set s1, Set s2);
- two sets are disjoint if the intersection is empty
int equal(Set s1, Set s2);
- two sets are equal if they have exactly the same elements

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Please write a main method in C that calls and implements all of the functions above.
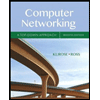
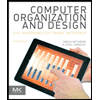
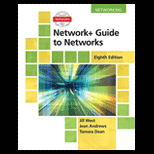
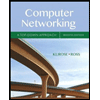
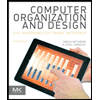
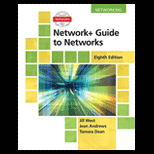
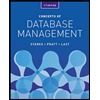
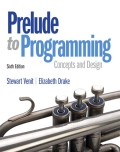
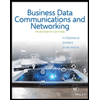