Please write the script and introduction for the program so that the number of words is between 300-400 //importing scanner import java.util.Scanner; //our main class public class Main { //our main method public static void main(String args[]) { //declaring pegs int pegs; //declaring source,destination,middleone strings String source,destination,middleone; //creating scanner class Scanner mine = new Scanner(System.in); //asking number of pegs System.out.print("Please enter the number of pegs: "); //user input pegs=mine.nextInt(); //to the nextline mine.nextLine(); //printing System.out.print("Please enter the source name: "); //user input source=mine.nextLine(); //printing System.out.print("Please enter the destination name: "); //user input destination=mine.nextLine(); //printing System.out.print("Please enter the middle_one name: "); //user input middleone=mine.nextLine(); //printing new line System.out.println("\n\n"); //calling our function Towers_of_Hanoi Towers_of_Hanoi(pegs,source,destination,middleone); //closing scanner class mine.close(); } //our recursive function Towers of Hanoi public static void Towers_of_Hanoi(int pegs, String source, String destination, String middle_one) { //if condition if(pegs==1){ //printing System.out.println("MOVE Disc 1 from bar " + source + " to bar " + destination); //return statement return; } //calling Towers_of_Hanoi function Towers_of_Hanoi(pegs-1, source, middle_one, destination); //printing System.out.println("MOVE Disc " + pegs + " from bar " + source + " to bar " + destination); //calling Towers_of_Hanoi function Towers_of_Hanoi(pegs-1, middle_one, destination, source); } }
Please write the script and introduction for the program so that the number of words is between 300-400
//importing scanner
import java.util.Scanner;
//our main class
public class Main {
//our main method
public static void main(String args[])
{
//declaring pegs
int pegs;
//declaring source,destination,middleone strings
String source,destination,middleone;
//creating scanner class
Scanner mine = new Scanner(System.in);
//asking number of pegs
System.out.print("Please enter the number of pegs: ");
//user input
pegs=mine.nextInt();
//to the nextline
mine.nextLine();
//printing
System.out.print("Please enter the source name: ");
//user input
source=mine.nextLine();
//printing
System.out.print("Please enter the destination name: ");
//user input
destination=mine.nextLine();
//printing
System.out.print("Please enter the middle_one name: ");
//user input
middleone=mine.nextLine();
//printing new line
System.out.println("\n\n");
//calling our function Towers_of_Hanoi
Towers_of_Hanoi(pegs,source,destination,middleone);
//closing scanner class
mine.close();
}
//our recursive function Towers of Hanoi
public static void Towers_of_Hanoi(int pegs, String source, String destination, String middle_one)
{
//if condition
if(pegs==1){
//printing
System.out.println("MOVE Disc 1 from bar " + source + " to bar " + destination);
//return statement
return;
}
//calling Towers_of_Hanoi function
Towers_of_Hanoi(pegs-1, source, middle_one, destination);
//printing
System.out.println("MOVE Disc " + pegs + " from bar " + source + " to bar " + destination);
//calling Towers_of_Hanoi function
Towers_of_Hanoi(pegs-1, middle_one, destination, source);
}
}
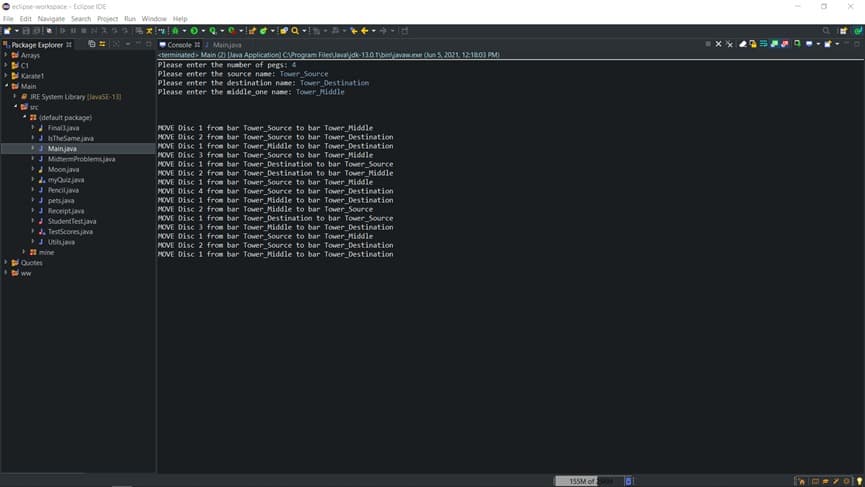

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

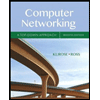
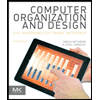
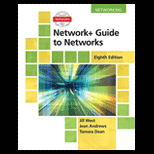
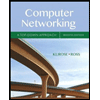
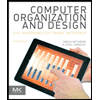
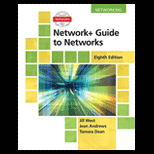
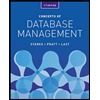
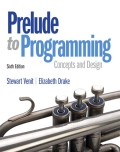
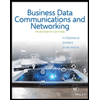