P| Understanding if Statements In this lab, you complete a prewritten C++ program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: • The charge for all signs is a minimum of $35.00. • The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. • If the sign is made of oak, add $20.00. No charge is added for pine. • Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.cpp is open in the code editor. 2. You need to declare variables for the following, and initialize them where specified: o A variable for the cost of the sign initialized to 0.00 ( charge ). o A variable for the number of characters initialized to 8 ( numChars ). o A variable for the color of the characters initialized to "gold" ( color ). o A variable for the wood type initialized to
P| Understanding if Statements In this lab, you complete a prewritten C++ program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: • The charge for all signs is a minimum of $35.00. • The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. • If the sign is made of oak, add $20.00. No charge is added for pine. • Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.cpp is open in the code editor. 2. You need to declare variables for the following, and initialize them where specified: o A variable for the cost of the sign initialized to 0.00 ( charge ). o A variable for the number of characters initialized to 8 ( numChars ). o A variable for the color of the characters initialized to "gold" ( color ). o A variable for the wood type initialized to
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
// HouseSign.cpp - This program calculates prices for custom made signs.
#include <iostream>
#include <string>
using namespace std;
int main()
{
// This is the work done in the housekeeping() function
// Declare and initialize variables here
// Charge for this sign
// Color of characters in sign
// Number of characters in sign
// Type of wood
// This is the work done in the detailLoop() function
// Write assignment and if statements here
// This is the work done in the endOfJob() function
// Output charge for this sign
cout << "The charge for this sign is $" << charge << endl;
return(0);
}
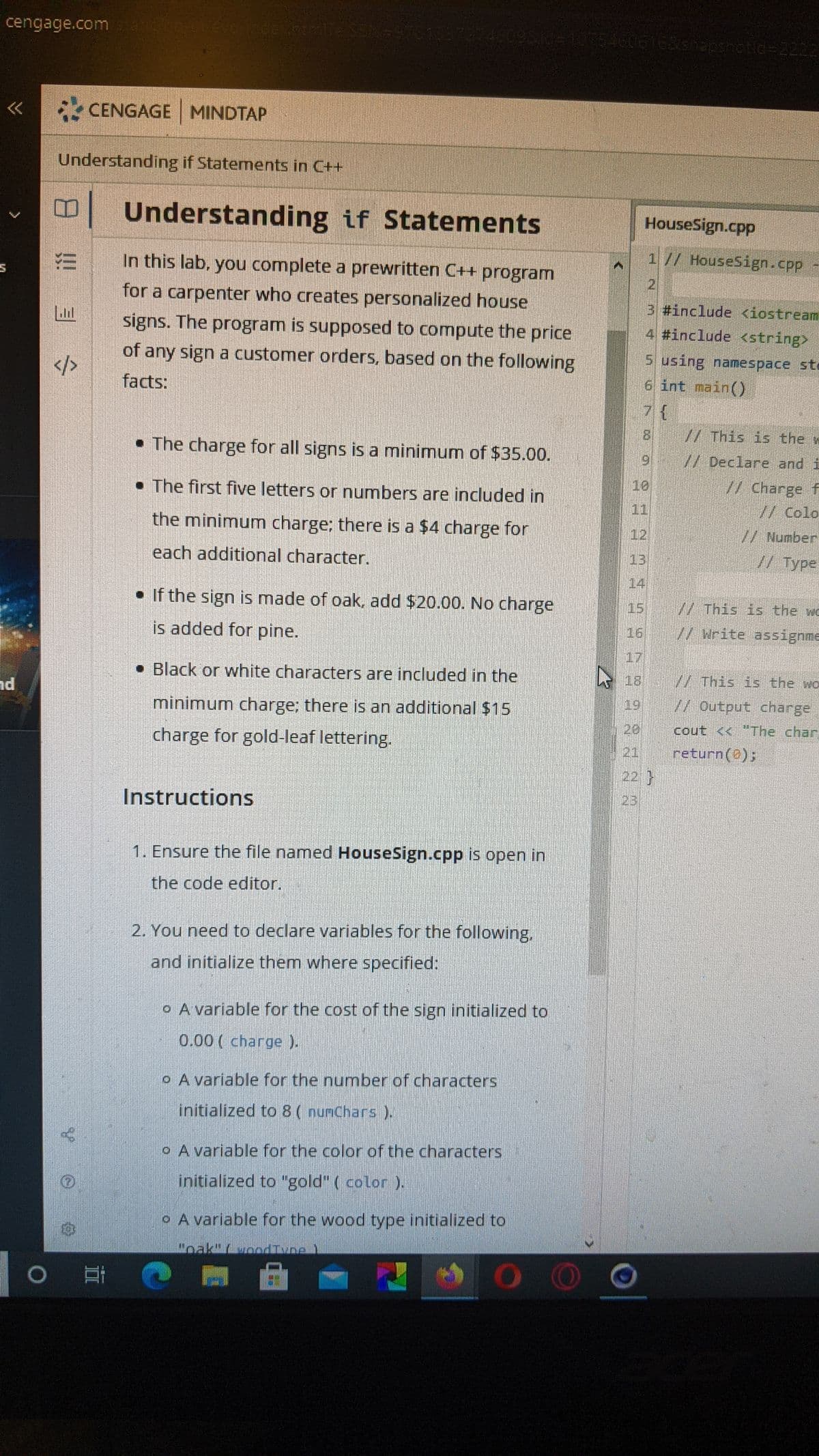
Transcribed Image Text:cengage.com
4009510-103546061638h2pshotid=2222
く
* CENGAGE MINDTAP
Understanding if Statements in C++
Understanding if Statements
HouseSign.cpp
In this lab, you complete a prewritten C++ program
1// HouseSign.cpp -
21
for a carpenter who creates personalized house
3 #include <iostream
signs. The program is supposed to compute the price
4 #include <string>
of any sign a customer orders, based on the following
5 using namespace sto
6 int main()
</>
facts:
7{
8 // This is the w
• The charge for all signs is a minimum of $35.00.
9 // Declare and i
• The first five letters or numbers are included in
10
//Charge f
// Colo
11
the minimum charge; there is a $4 charge for
12
//Number
each additional character.
13
// Type
14
• If the sign is made of oak, add $20.00. No charge
15
//This is the wo
is added for pine.
// Write assignne
16
17
• Black or white characters are included in the
18
V/ This is the wo
minimum charge; there is an additional $15
19
// Output charge
charge for goldHeaf lettering.
20,
cout <<"The char
(21
return(0);
22}
23
Instructions
1. Ensure the file named HouseSign.cpp is open in
the code editor.
2. You need to declare variables for the following.
and initialize them where specified:
o A variable for the cost of the sign initialized to
0.00 ( charge ).
o A variable for the number of characters
initialized to 8 ( numChars).
o A variable for the color of the characters
initialized to "gold" ( color ).
oA variable for the wood type initialized to
Hoak"(vodIvne)
OO
!!!
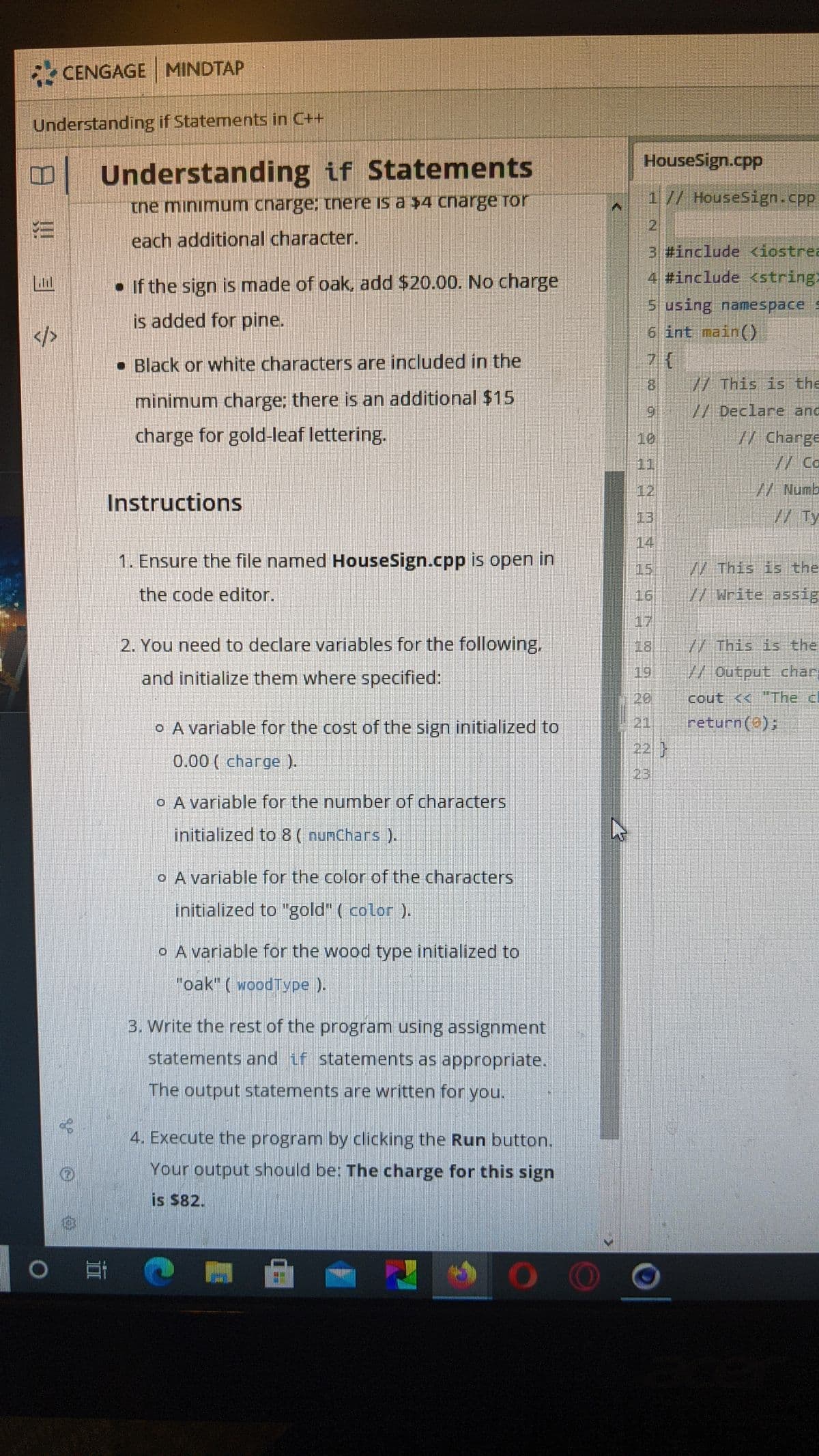
Transcribed Image Text:* CENGAGE MINDTAP
Understanding if Staterments in C++
HouseSign.cpp
Understanding if Statements
1// HouseSign.cpp
tne minimum cnarge; tnere is a $4 charge for
21
each additional character.
3 #include <iostrea
4 #include <string:
• If the sign is made of oak, add $20.00. No charge
5 using namespace s
is added for pine.
</>
6 int main()
7 (
8 // This is the
• Black or white characters are included in the
minimum charge: there is an additional $15
9 // Declare and
charge for gold-leaf lettering.
10
//Charge
11
// Ca
12
//Numb
Instructions
13
//Ty
14
1. Ensure the file named HouseSign.cpp is open in
15 // This is the
the code editor.
16
//Write assig
17
2. You need to declare variables for the following,
18 // This is the
and initialize them where specified:
19
//Output char
20
cout <<The cl
return(0);
22}
O A variable for the cost of the sign initialized to
21
0.00 ( charge ).
23
o A variable for the number of characters
initialized to 8( numChars).
o A variable for the color of the characters
initialized to "gold" ( color ).
o A variable for the wood type initialized to
"oak" ( vood Type ).
3. Write the rest of the program using assignment
statements and if statements as appropriate.
The output statements are written for you.
4. Execute the program by clicking the Run button.
Your output should be: The charge for this sign
is $82.
0耳
!!
目
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
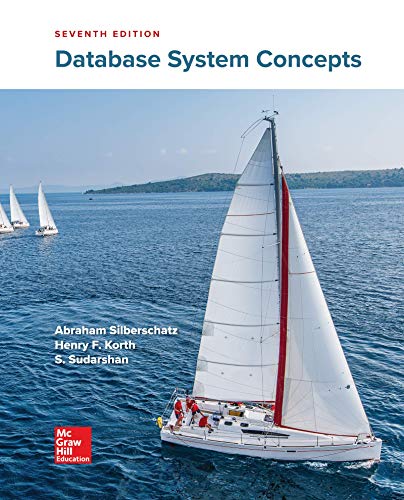
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
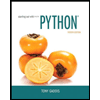
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
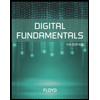
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
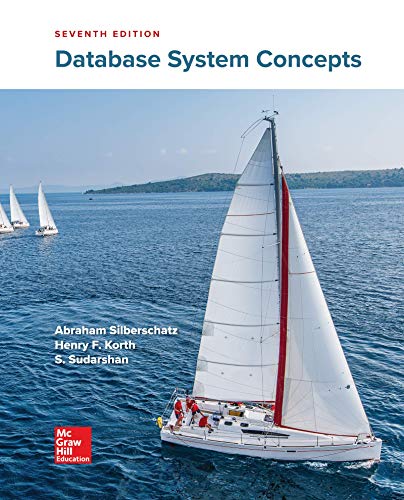
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
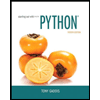
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
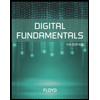
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
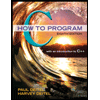
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
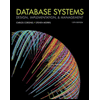
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
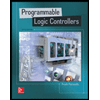
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education