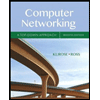
- Practice recursion on lists
- Practice multiple base conditions
- Combine recursion call with and,or not operators
- Search for an element in a list using recursion
Instructions
In this lab, we will write a recursive function for searching a list of integers/strings.
Write a recursive function to determine if a list contains an element.
Name the function recursive_search(aList, value), where aList is a list and value if primitive type is the object we want to search for.
Return a boolean, specifically True if and only if value is an element of aList else return False from the function
Examples:
recursive_search([1,2,3], 2) == True recursive_search([1,2,3], 4) == False recursive_search([ ], 4) == False recursive_search([ [ 1 ], 2 ], 1) == False # -----> (because the list contains [ 1 ] and 2, not 1)
Hint:
Think about what the base case is? When is it obvious that the element is not in the list? If we are not at the base case, how can you use the information about the first element of the list to possibly see if the "value" exists in the list?
Call the function recursively on a list of the last n-1 elements, essentially asking "does the element exist somewhere down the list"? Use that information when returning a value (True/False).
For on a list of size n, make the recursive call on the last n-1 elements, also called the tail of the list.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Why does dynamic programming provide faster solutions that recursive algorithms solving the same problem? 1.avoids resolving overlapping subproblems 2.dynamic programming uses dynamic memory that is more efficient than stack memory 3.loops are always faster than recursion 4.dynamic uses arrays that are faster than function callsarrow_forwardHow would I write double_str and double_list using only recursion functions?arrow_forwardWrite a statement that calls the recursive function backwards_alphabet() with input starting_letter.arrow_forward
- Write a recursive function to sort an array of integers into ascending order using the following idea: the function must place the smallest element in the first position, then sort the rest of the array by a recursive call. This is a recursive version of the selection sort. (Note: You will probably want to call an auxiliary function that finds the index of the smallest item in the array. Make sure that the sorting function itself is recursive. Any auxiliary function that you use may be either recursive or iterative.) Embed your sort function in a driver program to test it. Turn in the entire program and the output.arrow_forwardIndirect recursion is when function A calls function B, which in turn calls function A. is it true or false.arrow_forwardT/F 9. As loop variables and recursive solutions are also not used for iterative solutions, the recursive solution generally makes memorization more effective (use fewer stored) than the corresponding iterative solution.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
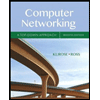
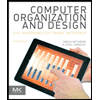
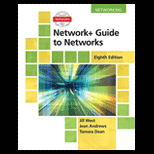
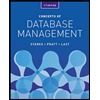
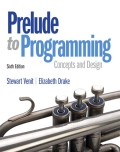
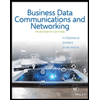