print the values using the function defined above for (int i = 0; i < counter; i++) { printNutritionData(nutritions[i]); } return 0;
Can you fix the code to out put the same as the picture.
// #include <iostream>
// #include <string>
#include <bits/stdc++.h>
using namespace std;
class NutritionData {
private:
//to store the food name
string foodName;
//to store the serving size of a food type
int servingSize;
//to store amount of cal coming from carbs in the food type
double calFromCarb;
//to store amount of cal coming from Fat in the food type
double calFromFat;
//to store amount of cal coming from protien in the food type
double calFromProtien;
public:
//default constructor
NutritionData();
//mutator for food name
void setFoodName(string foodName);
//mutator for servingSize
void setServingSize(int servingSize);
//mutator for calFromCarb
void setCalFromCarb(double calFromCarb);
//mutator for calFromFat
void setCalFromFat(double calFromFat);
// mutator for calFromProtein
void setCalFromProtien(double calFromProtien);
//accessor for food name
string getFoodName();
//accessor for foodserving size
int getServingSize();
//accessor for calFromCarb
double getCalFromCarb();
//accessor for calFromFat
double getCalFromFat();
//accessor for calFromProtien
double getCalFromProtien();
//get calorie per serving calculates the calories in one serving of the food
double getCaloriesPerServing();
};
//get calorie per serving
double NutritionData::getCaloriesPerServing() {
return (calFromCarb + calFromFat + calFromProtien);
}
//default constructor
NutritionData::NutritionData() {
foodName = "";
servingSize = 0;
calFromCarb = 0.0;
calFromFat = 0.0;
calFromProtien = 0.0;
}
//mutator for food name
void NutritionData::setFoodName(string foodName) {
this->foodName = foodName;
}
//mutator for servingSize
void NutritionData::setServingSize(int servingSize) {
this->servingSize = servingSize;
}
//mutator for calFromCarb
void NutritionData::setCalFromCarb(double calFromCarb) {
this->calFromCarb = calFromCarb;
}
//mutator for calFromFat
void NutritionData::setCalFromFat(double calFromFat) {
this->calFromFat = calFromFat;
}
//mutator for calFromProtien
void NutritionData::setCalFromProtien(double calFromProtien) {
this->calFromProtien = calFromProtien;
}
//accessor for food name
string NutritionData::getFoodName() {
return foodName;
}
//accessor for servingSize
int NutritionData::getServingSize() {
return servingSize;
}
//accessor for calFromCarb
double NutritionData::getCalFromCarb() {
return calFromCarb;
}
//accessor for calFromFat
double NutritionData::getCalFromFat() {
return calFromFat;
}
//accessor for calFromProtien
double NutritionData::getCalFromProtien() {
return calFromProtien;
}
//prniting the nutrition data of a food type
void printNutritionData( NutritionData &n) {
cout << "\nFood Name: " << n.getFoodName() << endl;
cout << " Serving Size: " << n.getServingSize() << " grams\n";
cout << " Calories Per Serving: " << n.getCaloriesPerServing() << endl;
cout << " Calories From Carb: " << n.getCalFromCarb() << endl;
cout << " Calories From Fat: " << n.getCalFromFat() << endl;
cout << " Calories From Protien: " << n.getCalFromProtien() << endl;
}
int main() {
// read the values from a text file
// save the text file in the same directory as "nutri.txt"
ifstream input("nutri.txt");
// stores the total number of inputs
int N;
// create an array of class type NutritionData objects
NutritionData nutritions[100];
// counter for the array
int counter = 0;
// bool to check for first
bool first = true;
// first line contains the number of inputs
for (string line; getline(input, line);) {
if (first) {
first = false;
continue;
}
// stores the four cal values
// 0: serving size, 1:cal carb, 2: cal fat, 3: protein
double calValues[4] = {0};
int calCounter = 0;
bool foundName = false;
// now process the line
string foodName;
string curr = "";
for (int i = 0; i < line.length(); i++) {
if (line[i] == ',') {
if (foundName) {
calValues[calCounter++] = stod(curr);
} else {
foodName = curr;
foundName = true;
}
curr = "";
} else {
curr += line[i];
}
}
calValues[calCounter++] = stod(curr);
// insert the values in the nutritions array
nutritions[counter].setFoodName(foodName);
nutritions[counter].setServingSize(calValues[0]);
nutritions[counter].setCalFromCarb(calValues[1]);
nutritions[counter].setCalFromFat(calValues[2]);
nutritions[counter].setCalFromProtien(calValues[3]);
counter++;
}
// print the values using the function defined above
for (int i = 0; i < counter; i++) {
printNutritionData(nutritions[i]);
}
return 0;
}

Step by step
Solved in 2 steps

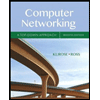
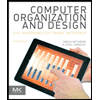
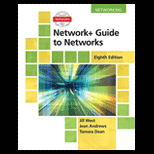
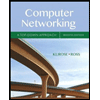
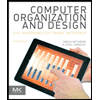
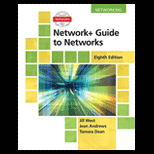
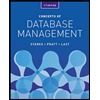
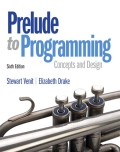
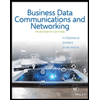