Problem 5: Implement Queue class Queue is data structure which maintains FIFO (First IN First OUT) policy. Create a class named Queue with following methods and attributes: Class Queue: double[] queueElements (public), int queueCapacity (private), int lastIndex (private) public Queue(int capacity) { //assign the member variables } void enqueue(double n) { //add this n to end of the queueElements array, show error if no capacity to hold this value } void dequeue() { //remove the first element of the queueElements array. Update the array accordingly. } void printElements() { //show the elements of queueElements array. } See the following main method and observe the output. Complete the Queue class to get the output exactly shown in the table below: QueueTest.java Ouput public class QueueTest { public static void main(String[] args) { Queue q=new Queue(5); q.enqueue(5.2); q.enqueue(2.1); q.enqueue(-0.2); q.enqueue(7.88); q.enqueue(5.5); q.enqueue(1.1); q.printElements(); q.dequeue(); q.printElements(); q.dequeue(); q.dequeue(); q.printElements(); q.enqueue(10.666); q.printElements(); } } Creating a Queue: Capacity 5 Enqueue 5.2: Successful Enqueue 2.1: Successful Enqueue -0.2: Successful Enqueue 7.88: Successful Enqueue 5.5: Successful Error Enqueuing 1.1 Showing Elements of queue: 5.2 2.1 -0.2 7.88 5.5 Dequeuing: Successful Showing Elements of queue: 2.1 -0.2 7.88 5.5 Dequeuing: Successful Dequeuing: Successful Showing Elements of queue: 7.88 5.5 Enqueue 10.666: Successful Showing Elements of queue: 7.88 5.5 10.666
Problem 5: Implement Queue class
Queue is data structure which maintains FIFO (First IN First OUT) policy.
Create a class named Queue with following methods and attributes:
Class Queue:
double[] queueElements (public), int queueCapacity (private), int lastIndex (private)
public Queue(int capacity) {
//assign the member variables
}
void enqueue(double n) {
//add this n to end of the queueElements array, show error if no capacity to hold this value
}
void dequeue() {
//remove the first element of the queueElements array. Update the array accordingly.
}
void printElements() {
//show the elements of queueElements array.
}
See the following main method and observe the output. Complete the Queue class to get the output exactly shown in the table below:
QueueTest.java |
Ouput
|
public class QueueTest {
|
Creating a Queue: Capacity 5
Enqueue 5.2: Successful Enqueue 2.1: Successful Enqueue -0.2: Successful Enqueue 7.88: Successful Enqueue 5.5: Successful Error Enqueuing 1.1
Showing Elements of queue: 5.2 2.1 -0.2 7.88 5.5
Dequeuing: Successful Showing Elements of queue: 2.1 -0.2 7.88 5.5
Dequeuing: Successful Dequeuing: Successful Showing Elements of queue: 7.88 5.5
Enqueue 10.666: Successful Showing Elements of queue: 7.88 5.5 10.666
|

Step by step
Solved in 3 steps with 1 images

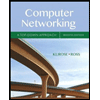
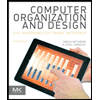
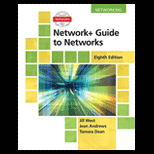
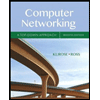
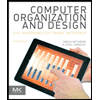
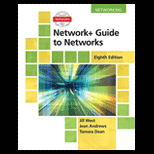
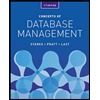
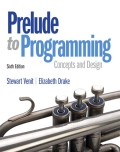
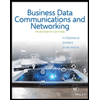