problem described below: Have the user input test grades UNTIL user enters a negative number. The program will: list the number of grades entered, list the number of passing grades (>= 60) total all the grades, and then display the average of the grades. For example: Enter score 1: 88.8 Enter score 2: 50 Enter score 3: 100 Enter score 4: 100 Enter score 5: -1 Total number of grades: 4 Total number of passing grades: 3 Total: 338.8 Average: 84.7 (NOTE: Follow the steps below in creating your Algorithm and C++ Program. Remember, you may enter 3 grades, or 7 grades or 9 grades. Your program will keep adding the grades until it reads in a negative number) 3. The first thing you should enter in your source file is a comment block. Add a comment block at the top of the .cpp file (your program file), which includes the following: /* Name: Enter your full name here Lab: Chapter 5 Description: Enter the description of the program here Algorithm: Enter the Algorithm */
language is C++
Use Lastname First name, JonnyEnglish
Instructions
1. Using Visual Studio, or Xcode, create a new empty project in your working drive. Name the Project: 05LastFirst
(NOTE: where LastFirst is your actual Lastname and Firstname. For Example, if your name is Mary Smith then your
empty project folder will be named 02SmithMary)
2. You will be updating your 05CH3 program. You will develop an
problem described below:
Have the user input test grades UNTIL user enters a negative number. The program will:
list the number of grades entered,
list the number of passing grades (>= 60)
total all the grades, and
then display the average of the grades.
For example:
Enter score 1: 88.8
Enter score 2: 50
Enter score 3: 100
Enter score 4: 100
Enter score 5: -1
Total number of grades: 4
Total number of passing grades: 3
Total: 338.8
Average: 84.7
(NOTE: Follow the steps below in creating your Algorithm and C++ Program. Remember, you may enter 3 grades, or
7 grades or 9 grades. Your program will keep adding the grades until it reads in a negative number)
3. The first thing you should enter in your source file is a comment block. Add a comment block at the top of the .cpp file
(your program file), which includes the following:
/*
Name: Enter your full name here
Lab: Chapter 5
Description: Enter the description of the program here
Algorithm: Enter the Algorithm
*/
4. You will be typing an Algorithm for the problem described in step 2 in the comment section of the program (see
comment block in Step 3). Remember, an algorithm contains the steps necessary to solve the problem. Think of it as
the outline to your program! You will be creating a simple outline before you sit down and start writing the
code/program. You will be entering that outline in the comment block at the top of your program.
For example, your algorithm in the comment block could start like this:
a. Declare an (non-constant) integer variable for # of tests: num_tests=0
b. Declare an integer variable for # of passing grades: num_pass=0
c. Declare a double variable for an inputted test grade: inputtedTest=0
d. Declare a double variable for the running total of the grades: total=0
e. Declare a double variable for the average: average=0
f. Make sure and set all the above variables to 0 to start off
g. ------------------------------------------------------------
h. Create a while loop that runs until inputtedTest >= 0
a. Increment num_tests++ (it’ll be 1 the first time, 2 the second
time, etc...)
b. Prompt user to enter a test grade using:
cout << "Enter score " << num_tests << ": ";
c. Using cin >> inputtedTest read in the first test
d. Now add that inputtedTest to the running total:
total += inputtedTest;
e. Using an if statement check to see if inputtedTest is greater than
or equal to 0 and if so, increment num_pass++
i. NEED TO ADJUST THE num_tests variable and total variable. WHY???? Print
out those values. What do you notice??? You added the negative number to
the total, so you need to subtract that number from total and also adjust
the num_tests.
j.Calculate the average and save in averageand print it out using cout

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

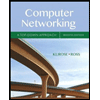
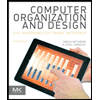
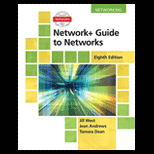
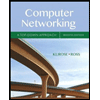
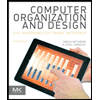
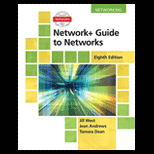
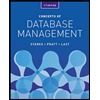
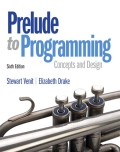
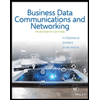