Problem Description: 1. Design a class Date. Each object represents information about a date. The class should contain the following data members and methods: Data members: • Day • Month • Year Methods • Constructors to initialize data members. Use default arguments so that users can omit some fields. • Methods to get/set all data members • Assignment operator (overload operator =) • Comparison operators (overload operator <, ==, >) • Input and output operators to read date field values from an input stream and to write the fields of an object to an output stream, nicely formatted. prompt a user to enter the field values as follows: Enter day, or to end:23 Enter month:8 Enter year:2022 The output of this date object may look like this: 8/23/2022 2. Design a class Student. Each object represents information about a single student. The class should contain the following data members and methods: Data members: • Identifier • First name, last name • Address • Date of first enrollment o Use Date class defined in item 1. • Number of credit hours completed Methods • Constructors to initialize data members. Use default arguments so that users can omit some fields. • Methods to get/set all data members • Assignment operator (overload operator =) • A method to increment the number of credit hours • Input and output operators to read student fields values from an input stream and to write the fields of an object to an output stream, nicely formatted. prompt a user to enter the field values as follows: Enter student ID, or to end:12345678 Enter first name: John Enter last name: Smith Enter address: 123 16th Street, University St Enter the date of first enrollment: Enter day, or to end:23 Enter month:8 Enter year:2022 Enter the number of credit hours completed: 88 The output of this student object may look like this: 12345678 John Smith 123 16th Street, University St , 665678 8/23/2022 88 3. Design a class CourseRegistration. Each object represents the enrollment of a student in a course. Data members: • Course identifier • Student identifier • Number of credit hours • Course grade Methods • Constructors to initialize data members. Use default arguments so that users can omit some fields. • Methods to get/set all data members • Input and output operators to read CourseRegistration fields values from an input stream and to write the fields of an object to an output stream, nicely formatted. prompt a user to enter the field values as follows Enter course ID, or to end: 33164 Enter student ID: 12345678 Enter number of credit hours: 3 Enter course grade: A The output of this courseRegistration object may look like this: 33164 12345678 3 A Requirements • Define each class in a header file (className.h) and implement the member functions in an implementation file (className.cpp), and compile it separately from a client program (test driver) . • Write a test driver for each class to demonstrate the correctness of all member functions and operators. Specifically, o In the test driver for the class Student, read a group of student data from the keyboard and output the data in a file o In the test driver for the class CourseRegistration, read course registration information for a group of students from the keyboard and output the data in a file.
Please provide full implementation of the three required classes( Date , Student, CourseRegistration) below in C++ language , don't copy from other chegg posts, you will get banned!
The output should be written to a file as a stream, see details below:
Problem Description:
1. Design a class Date. Each object represents information about a date. The class should contain the following data members and methods:
Data members: • Day • Month • Year Methods
• Constructors to initialize data members. Use default arguments so that users can omit some fields.
• Methods to get/set all data members
• Assignment operator (overload operator =)
• Comparison operators (overload operator <, ==, >)
• Input and output operators to read date field values from an input stream and to write the fields of an object to an output stream, nicely formatted.
prompt a user to enter the field values as follows:
Enter day, or to end:23
Enter month:8
Enter year:2022
The output of this date object may look like this: 8/23/2022
2. Design a class Student. Each object represents information about a single student. The class should contain the following data members and methods:
Data members: • Identifier • First name, last name • Address • Date of first enrollment
o Use Date class defined in item 1.
• Number of credit hours completed Methods
• Constructors to initialize data members. Use default arguments so that users can omit some fields.
• Methods to get/set all data members • Assignment operator (overload operator =)
• A method to increment the number of credit hours
• Input and output operators to read student fields values from an input stream and to write the fields of an object to an output stream, nicely formatted.
prompt a user to enter the field values as follows:
Enter student ID, or to end:12345678
Enter first name: John Enter last name: Smith
Enter address: 123 16th Street, University St
Enter the date of first enrollment: Enter day, or to end:23
Enter month:8 Enter year:2022 Enter the number of credit hours completed: 88
The output of this student object may look like this: 12345678 John Smith 123 16th Street, University St , 665678 8/23/2022 88
3. Design a class CourseRegistration. Each object represents the enrollment of a student in a course.
Data members:
• Course identifier
• Student identifier
• Number of credit hours
• Course grade Methods
• Constructors to initialize data members.
Use default arguments so that users can omit some fields.
• Methods to get/set all data members
• Input and output operators to read CourseRegistration fields values from an input stream and to write the fields of an object to an output stream, nicely formatted.
prompt a user to enter the field values as follows
Enter course ID, or to end: 33164
Enter student ID: 12345678
Enter number of credit hours: 3
Enter course grade: A
The output of this courseRegistration object may look like this: 33164 12345678 3 A
Requirements
• Define each class in a header file (className.h) and implement the member functions in an implementation file (className.cpp), and compile it separately from a client program (test driver)
. • Write a test driver for each class to demonstrate the correctness of all member functions and operators.
Specifically,
o In the test driver for the class Student, read a group of student data from the keyboard and output the data in a file
o In the test driver for the class CourseRegistration, read course registration information for a group of students from the keyboard and output the data in a file.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

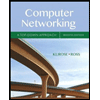
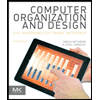
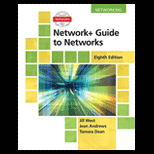
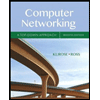
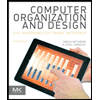
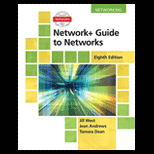
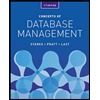
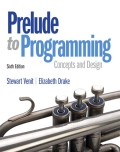
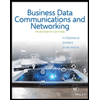