Problem Statement Create a class named Circle with a single private member variable called radius of type (int. Add getter, setter and area functions. Overload the operators and the stream operator <<. Write a program that creates two objects of type Circle of radius 15 and 10. Add both, subtract the second from the first and print out both results using the overloaded stream insertion operator <<. Partial Solution Please try to implement the full source code #include your IDE first. The solution is partially provided below. Fill in the blanks to complete the missing parts and make sure to not add an empty space before and after the answer.
Problem Statement Create a class named Circle with a single private member variable called radius of type (int. Add getter, setter and area functions. Overload the operators and the stream operator <<. Write a program that creates two objects of type Circle of radius 15 and 10. Add both, subtract the second from the first and print out both results using the overloaded stream insertion operator <<. Partial Solution Please try to implement the full source code #include your IDE first. The solution is partially provided below. Fill in the blanks to complete the missing parts and make sure to not add an empty space before and after the answer.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter13: Overloading And Templates
Section: Chapter Questions
Problem 14PE
Related questions
Question
C++
![Question 1
Problem Statement
Create a class named Circle with a single private member variable called radius of type (int. Add getter, setter and area functions.
Overload the operators
and the stream operator <<.
Write a program that creates two objects of type Circle of radius 15 and 10. Add both, subtract the second from the first and print out both results using the overloaded stream insertion operator <<.
Partial Solution
Please try to implement the full source code in your IDE first. The solution partially provided below. Fill in the blanks to complete the missing parts and make sure to not add an empty space before and after the answer.
#include <iostream>
using namespace std;
}
private:
int radius;
public:
}
//Constructor overloading
Circle(int radius);
//Member function area, it output the area of a circle
area();
}
//Getter and setter
}
//Operator overloading
Circle operator + (const Circle& c);
//Constructure overloading
Circle :: Circle(int radius) {
this->radius = radius;
}
// Member function
int Circle::getRadius() const {
double
//Friend function and operator overloading
return
}
void Circle :: setRadius(int r) {
this->radius=r;
getRadius() const;
setRadius(int);
}
int main()
{
operator (const Circle& c);
}
//operator overloading
Circle Circle :: operator
int newRadius = this->getRadius() + c.getRadius();
Circle
return newCircle;
ostream& operator<<(ostream &out, Circle &c);
Circle c20
//Friend function, operator overloading
Circle c1(15):
cout << "C1 is " << c1:
Circle Circle ::
- (const Circle& c) {
int newRadius = this->getRadius() - c.getRadius();
Circle newCircle(newRadius);
return [newCircle];
Circle
cout << "C2 is " << c2;
->radius:
3.14* (this->radius *this->radius);
[return] 0;
out << "Radius: " << c.getRadius() << " Area : " << c.area() << "\n";
return out;
area() {
& operator << (ostream &out, Circle &c) {
Circle add c1 + c2:
cout << "c1 + c2 is " <<
cout << "c1-c2 is " << sub;
(const Circle& c) {
|);
= c1-c2;
);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdd27f7e0-834e-40ed-ac12-9ff71783ae4a%2Fc634e571-9e88-430e-8de0-fde2048fa1c3%2Fxj10gz8_processed.png&w=3840&q=75)
Transcribed Image Text:Question 1
Problem Statement
Create a class named Circle with a single private member variable called radius of type (int. Add getter, setter and area functions.
Overload the operators
and the stream operator <<.
Write a program that creates two objects of type Circle of radius 15 and 10. Add both, subtract the second from the first and print out both results using the overloaded stream insertion operator <<.
Partial Solution
Please try to implement the full source code in your IDE first. The solution partially provided below. Fill in the blanks to complete the missing parts and make sure to not add an empty space before and after the answer.
#include <iostream>
using namespace std;
}
private:
int radius;
public:
}
//Constructor overloading
Circle(int radius);
//Member function area, it output the area of a circle
area();
}
//Getter and setter
}
//Operator overloading
Circle operator + (const Circle& c);
//Constructure overloading
Circle :: Circle(int radius) {
this->radius = radius;
}
// Member function
int Circle::getRadius() const {
double
//Friend function and operator overloading
return
}
void Circle :: setRadius(int r) {
this->radius=r;
getRadius() const;
setRadius(int);
}
int main()
{
operator (const Circle& c);
}
//operator overloading
Circle Circle :: operator
int newRadius = this->getRadius() + c.getRadius();
Circle
return newCircle;
ostream& operator<<(ostream &out, Circle &c);
Circle c20
//Friend function, operator overloading
Circle c1(15):
cout << "C1 is " << c1:
Circle Circle ::
- (const Circle& c) {
int newRadius = this->getRadius() - c.getRadius();
Circle newCircle(newRadius);
return [newCircle];
Circle
cout << "C2 is " << c2;
->radius:
3.14* (this->radius *this->radius);
[return] 0;
out << "Radius: " << c.getRadius() << " Area : " << c.area() << "\n";
return out;
area() {
& operator << (ostream &out, Circle &c) {
Circle add c1 + c2:
cout << "c1 + c2 is " <<
cout << "c1-c2 is " << sub;
(const Circle& c) {
|);
= c1-c2;
);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
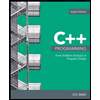
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
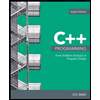
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning