Programming ASsignment: Abstract Data Type – Matrix The purpose of this course is to provide you with experience in: 1. Identification of the appropriate ABSTRACT DATA TYPE (model and operations) to use for a given application. 2. Select of the best DATA STRUCTURE and corresponding ALGORITHMS to implement the ADT in a programming language. There are many applications in mathematics, economics, engineering, etc. in which the underlying model is a matrix. Consequently, it would be useful to implement this ADT. The special case of a 2x2 matrix will be considered. MODEL: the general form of a 2x2 matrix is: all a12 | 2.5 -3.7 example: a21 a22 4 Where: al1, al2, a21, and a22 are real numbers (scalars) that are the COMPONENTS (or ELEMENTS) of the matrix. OPERATIONS: the following are some of the common operations that are performed on such matrices: Matrix addition: b12 | al2 | | + al1 b11 all +b12 a12 + b12 L a21 а22 b21 b22 a21 + b21 a22 + b22 Matrix subtraction: all a12 b11 b12 all - b12 al2 - b12 а21 a22 b21 b22 I a21 - b21 a22 - b22 Scalar multiplication: all a12 | k x a12 k x al2 k X a21 a22 kx a21 kx a22
Programming ASsignment: Abstract Data Type – Matrix The purpose of this course is to provide you with experience in: 1. Identification of the appropriate ABSTRACT DATA TYPE (model and operations) to use for a given application. 2. Select of the best DATA STRUCTURE and corresponding ALGORITHMS to implement the ADT in a programming language. There are many applications in mathematics, economics, engineering, etc. in which the underlying model is a matrix. Consequently, it would be useful to implement this ADT. The special case of a 2x2 matrix will be considered. MODEL: the general form of a 2x2 matrix is: all a12 | 2.5 -3.7 example: a21 a22 4 Where: al1, al2, a21, and a22 are real numbers (scalars) that are the COMPONENTS (or ELEMENTS) of the matrix. OPERATIONS: the following are some of the common operations that are performed on such matrices: Matrix addition: b12 | al2 | | + al1 b11 all +b12 a12 + b12 L a21 а22 b21 b22 a21 + b21 a22 + b22 Matrix subtraction: all a12 b11 b12 all - b12 al2 - b12 а21 a22 b21 b22 I a21 - b21 a22 - b22 Scalar multiplication: all a12 | k x a12 k x al2 k X a21 a22 kx a21 kx a22
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Hey Guys!
I just want to know how to write this program in c++?
How to add menu where the user can choose what type of operations he/she want to calculate?
and outpur everything in an output text file.
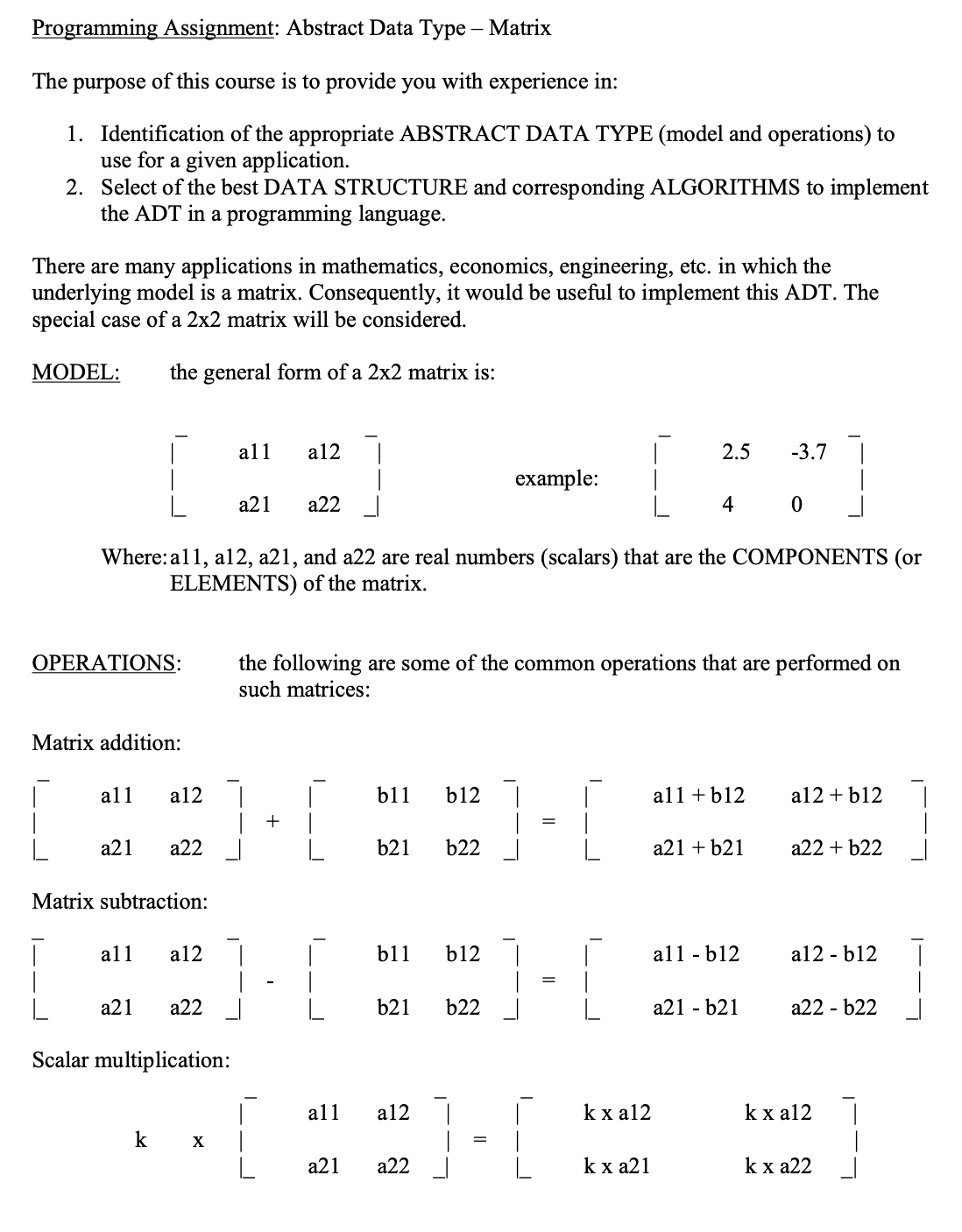
Transcribed Image Text:Programming Assignment: Abstract Data Type – Matrix
The purpose of this course is to provide you with experience in:
1. Identification of the appropriate ABSTRACT DATA TYPE (model and operations) to
use for a given application.
2. Select of the best DATA STRUCTURE and corresponding ALGORITHMS to implement
the ADT in a programming language.
There are many applications in mathematics, economics, engineering, etc. in which the
underlying model is a matrix. Consequently, it would be useful to implement this ADT. The
special case of a 2x2 matrix will be considered.
MODEL:
the general form of a 2x2 matrix is:
all
a12
2.5
-3.7
example:
а21
a22
4
Where: al1, al2, a21, and a22 are real numbers (scalars) that are the COMPONENTS (or
ELEMENTS) of the matrix.
the following are some of the common operations that are performed on
such matrices:
OPERATIONS:
Matrix addition:
all
a12
b11
b12 |
all + b12
a12 + b12
|
+
а21
а22
b21
b22
a21 + b21
a22 + b22
Matrix subtraction:
all
a12
b11
b12
all - b12
al2 - b12
а21
а22
b21
b22
a21 - b21
a22 - b22
Scalar multiplication:
all
a12 |
k x a12
k x al2
k
X
а21
а22
k x a21
k x a22
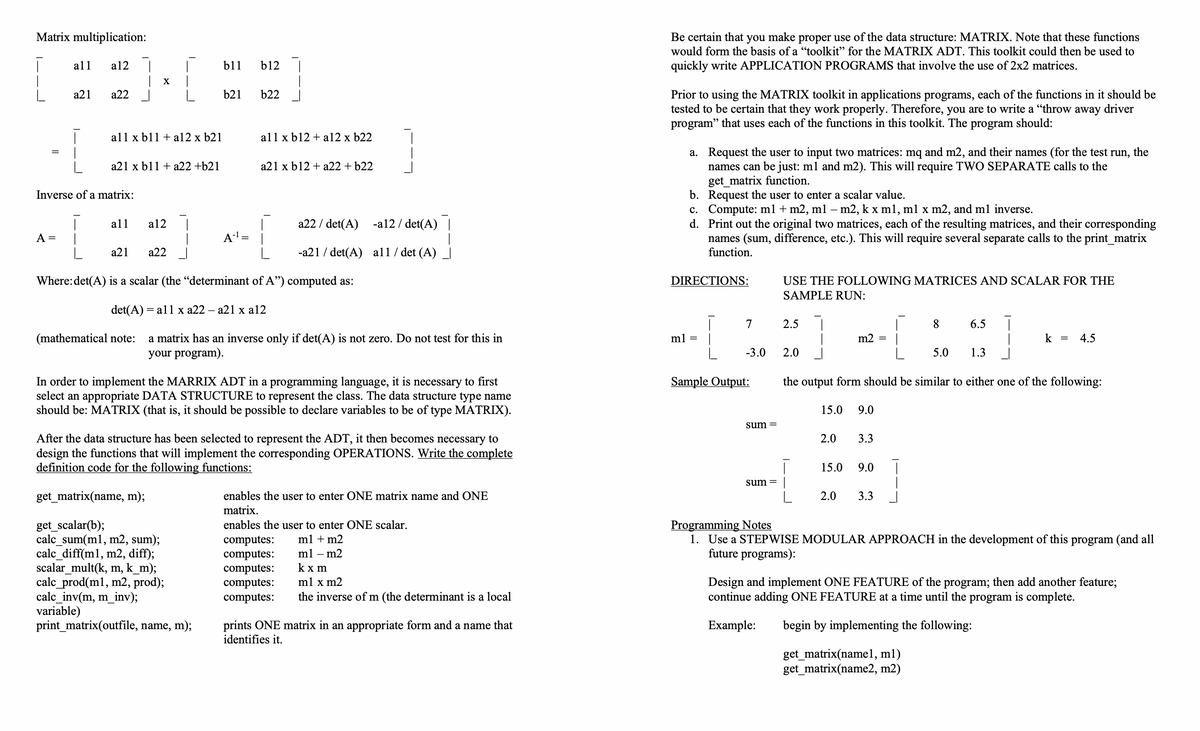
Transcribed Image Text:Matrix multiplication:
Be certain that you make proper use of the data structure: MATRIX. Note that these functions
would form the basis of a "toolkit" for the MATRIX ADT. This toolkit could then be used to
all
a12
b11
b12
quickly write APPLICATION PROGRAMS that involve the use of 2x2 matrices.
X
b22
Prior to using the MATRIX toolkit in applications programs, each of the functions in it should be
tested to be certain that they work properly. Therefore, you are to write a "throw away driver
program" that uses each of the functions in this toolkit. The program should:
а21
a22
b21
all x b11 + a12 x b21
all x b12 + a12 x b22
a. Request the user to input two matrices: mq and m2, and their names (for the test run, the
names can be just: ml and m2). This will require TWO SEPARATE calls to the
get_matrix function.
b. Request the user to enter a scalar value.
c. Compute: ml + m2, ml – m2, k x ml, ml x m2, and ml inverse.
d. Print out the original two matrices, each of the resulting matrices, and their corresponding
names (sum, difference, etc.). This will require several separate calls to the print_matrix
function.
a21 x b11 + a22 +b21
a21 x b12 + a22 + b22
Inverse of a matrix:
all
a12
a22 / det(A)
-a12 / det(A)
A =
A-'=
а21
а22
-a21 / det(A) all / det (A)
Where:det(A) is a scalar (the "determinant of A") computed as:
DIRECTIONS:
USE THE FOLLOWING MATRICES AND SCALAR FOR THE
SAMPLE RUN:
det(A) =
al1 x a22 – a21 x a12
7
2.5
8.
6.5
(mathematical note:
a matrix has an inverse only if det(A) is not zero. Do not test for this in
your program).
ml =
m2
k =
4.5
-3.0
2.0
5.0
1.3
Sample Output:
In order to implement the MARRIX ADT in a programming language, it is necessary to first
select an appropriate DATA STRUCTURE to represent the class. The data structure type name
should be: MATRIX (that is, it should be possible to declare variables to be of type MATRIX).
the output form should be similar to either one of the following:
15.0
9.0
sum =
After the data structure has been selected to represent the ADT, it then becomes necessary to
design the functions that will implement the corresponding OPERATIONS. Write the complete
definition code for the following functions:
2.0
3.3
15.0
9.0
sum =
get_matrix(name, m);
enables the user to enter ONE matrix name and ONE
2.0
3.3
matrix.
Programming Notes
1. Use a STEPWISE MODULAR APPROACH in the development of this program (and all
future programs):
get_scalar(b);
calc_sum(ml, m2, sum);
calc_diff(m1, m2, diff);
scalar_mult(k, m, k_m);
calc_prod(ml, m2, prod);
calc_inv(m, m_inv);
ple)
print_matrix(outfile, name, m);
enables the user to enter ONE scalar.
ml + m2
computes:
computes:
computes:
computes:
computes:
ml – m2
kx m
Design and implement ONE FEATURE of the program; then add another feature;
continue adding ONE FEATURE at a time until the program is complete.
ml x m2
the inverse ofm (the determinant is a local
var
prints ONE matrix in an appropriate form and a name that
identifies it.
Example:
begin by implementing the following:
get_matrix(name1, ml)
get_matrix(name2, m2)
---
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
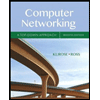
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
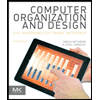
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
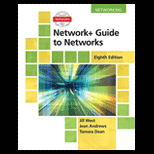
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
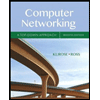
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
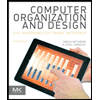
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
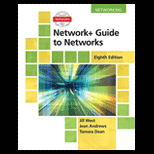
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
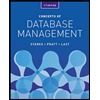
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
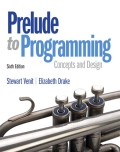
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
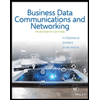
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY