Programming Assignment: Write a method called mode() that returns the most frequently occurring element in an array of integers. All the integers in the array will be in the range 1-9999. Assume that the array contains at least one element. Break ties (if there are any) by choosing the smallest value. For example, if your array contains the values [27, 15, 15, 11, 27], mode() should return the value 15.
Programming Assignment: Write a method called mode() that returns the most frequently occurring element in an array of integers. All the integers in the array will be in the range 1-9999. Assume that the array contains at least one element. Break ties (if there are any) by choosing the smallest value. For example, if your array contains the values [27, 15, 15, 11, 27], mode() should return the value 15.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
How could I edit my code so that it breaks ties? JAVA
![import java.util.Arrays;
LO
11 public class FindMost FrequentInt {
130 public static void main(String[] args) {
// Setting parameters for random int array
final int random IntArrayLength = 20;
final int maxIntValue = 10;
// Produce the random int, array, given the above parameters
int[] myRandom IntArray = createRandomArrayOfInts (random IntArrayLength, maxIntValue);
// From the above random int, array, find the most frequently occurring integer
int most Frequent Int=mode (myRandom IntArray);
// Show the results to the user
System.out.println("The array is: \n" + Arrays.toString(myRandom IntArray));
System.out.println("The most frequent integer is: " + most FrequentInt);
29
// Program end
30
}
32
// Method to construct an array of random integers
830
34
public static int[] createRandomArrayOfInts (int arrayLength, int maxIntValue) {
// Initialize an array of the proper size
int[] random IntArray = new int[arrayLength];
// Fill it with random integers between 0 and maxIntValue
for (int i = 0; i < random IntArray.length; i++) {
random IntArray[i] = (int) (Math.random()* (maxIntValue + 1));
}
return random IntArray;
}
// Method to detect most frequent integer within an integer array
public static int mode (int[] arrayOfInts) {
// Initialize variables concerning the most frequent integer found
int most FrequentInt = -1;
int mostTimes Found = 1;
// For each integer in the array
for (int index = 0; index < arrayOfInts.length; index++) {
int intOf Interest = arrayOfInts[index];
int timesThisIntWas Found = 0;
// Look through the array for matches
for (int i = index; i < arrayOfInts.length; i++) {
if(arrayOfInts[i] == intOf Interest) {
timesThisIntWas Found++;
}
}
// If the number of matches for this integer is the greatest, remember it
if(timesThisIntWas Found > mostTimesFound) {
most FrequentInt = intOf Interest;
mostTimesFound = timesThis IntWas Found;
}
}
return mostFrequentInt;
470
19
50](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34667835-2039-4add-9a5a-accc82008018%2Fbd775b4c-6a96-4c93-b11e-40dc4517be61%2Fxqc96co_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import java.util.Arrays;
LO
11 public class FindMost FrequentInt {
130 public static void main(String[] args) {
// Setting parameters for random int array
final int random IntArrayLength = 20;
final int maxIntValue = 10;
// Produce the random int, array, given the above parameters
int[] myRandom IntArray = createRandomArrayOfInts (random IntArrayLength, maxIntValue);
// From the above random int, array, find the most frequently occurring integer
int most Frequent Int=mode (myRandom IntArray);
// Show the results to the user
System.out.println("The array is: \n" + Arrays.toString(myRandom IntArray));
System.out.println("The most frequent integer is: " + most FrequentInt);
29
// Program end
30
}
32
// Method to construct an array of random integers
830
34
public static int[] createRandomArrayOfInts (int arrayLength, int maxIntValue) {
// Initialize an array of the proper size
int[] random IntArray = new int[arrayLength];
// Fill it with random integers between 0 and maxIntValue
for (int i = 0; i < random IntArray.length; i++) {
random IntArray[i] = (int) (Math.random()* (maxIntValue + 1));
}
return random IntArray;
}
// Method to detect most frequent integer within an integer array
public static int mode (int[] arrayOfInts) {
// Initialize variables concerning the most frequent integer found
int most FrequentInt = -1;
int mostTimes Found = 1;
// For each integer in the array
for (int index = 0; index < arrayOfInts.length; index++) {
int intOf Interest = arrayOfInts[index];
int timesThisIntWas Found = 0;
// Look through the array for matches
for (int i = index; i < arrayOfInts.length; i++) {
if(arrayOfInts[i] == intOf Interest) {
timesThisIntWas Found++;
}
}
// If the number of matches for this integer is the greatest, remember it
if(timesThisIntWas Found > mostTimesFound) {
most FrequentInt = intOf Interest;
mostTimesFound = timesThis IntWas Found;
}
}
return mostFrequentInt;
470
19
50
![Programming Assignment: Write a method called mode() that returns the most frequently occurring element in
an array of integers. All the integers in the array will be in the range 1-9999. Assume that the array contains at
least one element. Break ties (if there are any) by choosing the smallest value. For example, if your array
contains the values [27, 15, 15, 11, 27], mode() should return the value 15.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34667835-2039-4add-9a5a-accc82008018%2Fbd775b4c-6a96-4c93-b11e-40dc4517be61%2F25r0tok_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Programming Assignment: Write a method called mode() that returns the most frequently occurring element in
an array of integers. All the integers in the array will be in the range 1-9999. Assume that the array contains at
least one element. Break ties (if there are any) by choosing the smallest value. For example, if your array
contains the values [27, 15, 15, 11, 27], mode() should return the value 15.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
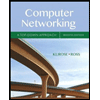
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
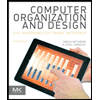
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
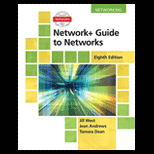
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
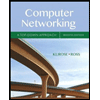
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
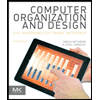
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
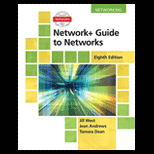
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
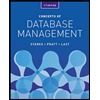
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
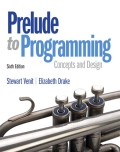
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
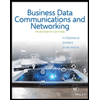
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY