Programming Question: Write a program in MIPS assembly language that asks the users to enter a value for array size “n” and fill up the array with n integers. Then reverse the array and print it on the screen. You are required to do the following in your program: Create up to 80 bytes of space in your program “n”, the array size, should be greater than zero and less than 20 Each element of the array should be positive and divisible by 3 Array should be reversed within itself. i.e. you must not create another array to place the elements in reverse order You need to use following functions: int main( ) { Begin:arraysize= readNum() Ok=verifySize(arraySize) if (ok==1) creatArray(array-size) else Go to Begin printArray(arraySize) reverseArray(arraySize) printArray (arraySize) } // ------------------------------------------------------------ int readNum() { input an integer return the integer to main program } // ------------------------------------------------------------ int versifySize( int arraySize) { if (0<arraySize< 20) return 1 else return 0 } // ------------------------------------------------------------ void createArray(int arraySize) { counter = 0 making_array: arrayEntry = readNum() ok = checkNumPositive(arrayEntry) if (ok == 1) validNum = divisibleBy3(arrayEntry) if (validNum==1) insert arrayEntry into the array counter = counter + 1 else go to making_array else go to making_array if (counter < arraySize) go to making_array } // ------------------------------------------------------------ void reverseArray(int arraySize) { head = first index of the array tail = last index of the array swap: if (head <=tail) { swap the content of head and tail (array[head] and array[tail] with each other increment the head value by one word decrement tail by one word go to swap } } // ------------------------------------------------------------ void printArray( int arraySize) { .. .. } // ------------------------------------------------------------ int divisibleBy3(int arryEntry) { … … } // ------------------------------------------------------------ int checkNumPositive(int arrayEntry) { … … }
Write a program in MIPS assembly language that asks the users to enter a value for array size “n” and fill up the array with n integers. Then reverse the array and print it on the screen. You are required to do the following in your program:
- Create up to 80 bytes of space in your program
- “n”, the array size, should be greater than zero and less than 20
- Each element of the array should be positive and divisible by 3
- Array should be reversed within itself. i.e. you must not create another array to place the elements in reverse order
You need to use following functions:
int main( )
{
Begin:arraysize= readNum()
Ok=verifySize(arraySize)
if (ok==1)
creatArray(array-size)
else
Go to Begin
printArray(arraySize)
reverseArray(arraySize)
printArray (arraySize)
}
// ------------------------------------------------------------
int readNum()
{
input an integer
return the integer to main program
}
// ------------------------------------------------------------
int versifySize( int arraySize)
{
if (0<arraySize< 20)
return 1
else
return 0
}
// ------------------------------------------------------------
void createArray(int arraySize)
{
counter = 0
making_array: arrayEntry = readNum()
ok = checkNumPositive(arrayEntry)
if (ok == 1)
validNum = divisibleBy3(arrayEntry)
if (validNum==1)
insert arrayEntry into the array
counter = counter + 1
else
go to making_array
else
go to making_array
if (counter < arraySize)
go to making_array
}
// ------------------------------------------------------------
void reverseArray(int arraySize)
{
head = first index of the array
tail = last index of the array
swap: if (head <=tail)
{
swap the content of head and tail (array[head] and array[tail] with each other
increment the head value by one word
decrement tail by one word
go to swap
}
}
// ------------------------------------------------------------
void printArray( int arraySize)
{
..
..
}
// ------------------------------------------------------------
int divisibleBy3(int arryEntry)
{
…
…
}
// ------------------------------------------------------------
int checkNumPositive(int arrayEntry)
{
…
…
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

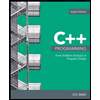
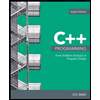