Project 2: Singly-Linked List (JAVA) The purpose of this is to assess your ability to: Implement sequential search algorithms for linked list structures Implement sequential abstract data types using linked data Analyze and compare algorithms for efficiency using Big-O notation I need to implement a singly-linked node class in JAVA. Use your singly-linked node to implement a singly-linked list class that maintains its elements in ascending order. The SinglyLinkedList class is defined by the following data: A node pointer to the front and the tail of the list Implement the following methods in your class: A default constructor list myList A copy constructor list myList(aList) Access to first element myList.front() Access to last element myList.back() Insert value myList.insert(val) Remove value at front myList.pop_front() Remove value at tail myList.pop_back() Determine if empty myList.empty() Return # of elements myList.size() Reverse order of elements in list myList.reverse() Merge with another ordered list myList.merge(aList) Write a thorough test program for this class. Time analysis should be included.
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Project 2: Singly-Linked List (JAVA)
The purpose of this is to assess your ability to:
- Implement sequential search
algorithms for linked list structures - Implement sequential abstract data types using linked data
- Analyze and compare algorithms for efficiency using Big-O notation
I need to implement a singly-linked node class in JAVA. Use your singly-linked node to implement a singly-linked list class that maintains its elements in ascending order.
The SinglyLinkedList class is defined by the following data:
- A node pointer to the front and the tail of the list
Implement the following methods in your class:
- A default constructor list<T> myList
- A copy constructor list<T> myList(aList)
- Access to first element myList.front()
- Access to last element myList.back()
- Insert value myList.insert(val)
- Remove value at front myList.pop_front()
- Remove value at tail myList.pop_back()
- Determine if empty myList.empty()
- Return # of elements myList.size()
- Reverse order of elements in list myList.reverse()
- Merge with another ordered list myList.merge(aList)
Write a thorough test program for this class. Time analysis should be included.

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

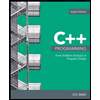
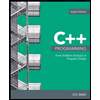