Project 4: Maze Solver (JAVA) The purpose of this assignment is to assess your ability to: Implement stack and queue abstract data types in JAVA Utilize stack and queue structures in a computational problem. For this question, implement a stack and a queue data structure. After testing the class, complete the depth-first search method (provided). The method should indicate whether or not a path was found, and, in the case that a path is found, output the sequence of coordinates from start to end. The following code and related files are provided. Carefully ready the code and documentation before beginning. A MazeCell class that models a cell in the maze. Each MazeCell object contains data for the row and column position of the cell, the next direction to check, and a bool variable that indicates whether or not the cell has already been visited. A Source file that creates the maze and calls your depth-first search method. An input file, maze.in, that may be used for testing. You will write the following: a generic stack class, called MyStack, that supports the provided API (see file stackAPI.png) a generic queue class, called MyQueue, that supports the provided API (see file queueAPI.png) an implementation for the depth-first algorithm Your data structure choices for the stack and queue implementation. Your use of MyStack and MyQueue structures in your maze solution Your depth first algorithm
Project 4: Maze Solver (JAVA) The purpose of this assignment is to assess your ability to: Implement stack and queue abstract data types in JAVA Utilize stack and queue structures in a computational problem. For this question, implement a stack and a queue data structure. After testing the class, complete the depth-first search method (provided). The method should indicate whether or not a path was found, and, in the case that a path is found, output the sequence of coordinates from start to end. The following code and related files are provided. Carefully ready the code and documentation before beginning. A MazeCell class that models a cell in the maze. Each MazeCell object contains data for the row and column position of the cell, the next direction to check, and a bool variable that indicates whether or not the cell has already been visited. A Source file that creates the maze and calls your depth-first search method. An input file, maze.in, that may be used for testing. You will write the following: a generic stack class, called MyStack, that supports the provided API (see file stackAPI.png) a generic queue class, called MyQueue, that supports the provided API (see file queueAPI.png) an implementation for the depth-first algorithm Your data structure choices for the stack and queue implementation. Your use of MyStack and MyQueue structures in your maze solution Your depth first algorithm
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question
100%
Project 4: Maze Solver (JAVA)
The purpose of this assignment is to assess your ability to:
- Implement stack and queue abstract data types in JAVA
- Utilize stack and queue structures in a computational problem.
For this question, implement a stack and a queue data structure. After testing the class, complete the depth-first search method (provided). The method should indicate whether or not a path was found, and, in the case that a path is found, output the sequence of coordinates from start to end.
The following code and related files are provided. Carefully ready the code and documentation before beginning.
- A MazeCell class that models a cell in the maze. Each MazeCell object contains data for the row and column position of the cell, the next direction to check, and a bool variable that indicates whether or not the cell has already been visited.
- A Source file that creates the maze and calls your depth-first search method.
- An input file, maze.in, that may be used for testing.
You will write the following:
- a generic stack class, called MyStack, that supports the provided API (see file stackAPI.png)
- a generic queue class, called MyQueue, that supports the provided API (see file queueAPI.png)
- an implementation for the depth-first
algorithm - Your data structure choices for the stack and queue implementation.
- Your use of MyStack and MyQueue structures in your maze solution
- Your depth first algorithm
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
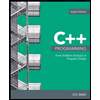
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
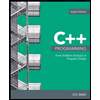
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning