Provide a time complexity analysis for this code #include int main(){ //Required matrices and variables int matrix1[6][6]; int matrix2[6][6]; int matrix3[6][6]; int i,j,k,n,m,l; //Reads number from user printf("Enter a number : "); scanf("%d", &n);
Provide a time complexity analysis for this code
#include<stdio.h>
int main(){
//Required matrices and variables
int matrix1[6][6];
int matrix2[6][6];
int matrix3[6][6];
int i,j,k,n,m,l;
//Reads number from user
printf("Enter a number : ");
scanf("%d", &n);
//Value for diagonal
m = 2*n;
//Temperary variable of 'n'
l = n;
//Creates matrix 1
for(i = 0; i< 6; i++){
k = l;
for(j = 0; j<6; j++){
if(j == (6-i-1))
matrix1[i][j] = m;
else if(j > (6-i-1))
matrix1[i][j] = (k++ + 1);
else matrix1[i][j] = k--;
}
l--;
}
//Prints matrix 1
for(i = 0; i< 6; i++){
printf("[");
for(j = 0; j<6; j++)
printf("%c ", (char)matrix1[i][j]);
printf("]\n");
}
printf("\n\n");
//Creates matrix 2
l = n;
for(i = 0; i<6 ; i++)
for(j = 0; j<6; j++){
if(i < 3 && j < 3)
matrix2[i][j] = l;
else if(i < 3 && j >= 3)
matrix2[i][j] = l + 1;
else if(i >= 3 && j < 3)
matrix2[i][j] = l + 2;
else matrix2[i][j] = l + 3;
}
//Prints matrix 2
for(i = 0; i< 6; i++){
printf("[");
for(j = 0; j<6; j++)
printf("%c ", (char)matrix2[i][j]);
printf("]\n");
}
printf("\n\n");
//Determines the difference between firts digit and diagonal digit
if(n % 2 == 1)
l = n - 12;
else l = n - 10;
//Creates matrix 3
for(i = 0; i< 6; i++){
k = l;
for(j = 0; j<6; j++){
if(j == (6-i-1))
matrix3[i][j] = n;
else if(j > (6-i-1)){
k = k - 2;
matrix3[i][j] = k;
}
else {
matrix3[i][j] = k;
k = k + 2;
}
}
l = l + 2;
}
//Prints matrix 3
for(i = 0; i< 6; i++){
printf("[");
for(j = 0; j<6; j++)
printf("%c ", (char)matrix3[i][j]);
printf("]\n");
}
}

Step by step
Solved in 2 steps

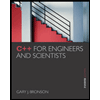
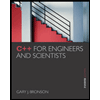