- Provide these 5 (screenshots)sample outputs and tell if each is a palindrome or not. Too bad--I hid a boot Some men interpret eight memos "Go Hang a Salami! I'm a Lasagna Hog" (title of a book on palindromes by Jon Agee, 1991) A man, a plan, a canal—Panama Gateman sees my name, garageman sees name tag LinkedStackADT.java public interface LinkedStackADT { boolean isEmptyStack(); void push(T val); T peek() throws StackUnderflowException; void pop() throws StackUnderflowException; } ============= StackUnderflowException.java public class StackUnderflowException extends Exception { public StackUnderflowException(){} public StackUnderflowException(String msg) { super(msg); } } ========= LinkedStackDS.java public class LinkedStackDS implements LinkedStackADT{ private class StackNode { T data; StackNode next; StackNode() { } StackNode(T data, StackNode next) { this.data = data; this.next = next; } public String toString() { return data.toString(); } } private StackNode top; public LinkedStackDS() { } @Override public boolean isEmptyStack() { return top == null; } @Override public void push(T val) { top = new StackNode(val, top); } @Override public T peek() throws StackUnderflowException { if(isEmptyStack()) throw new StackUnderflowException("stack is empty"); return top.data; } @Override public void pop() throws StackUnderflowException{ if(isEmptyStack()) throw new StackUnderflowException("stack is empty"); top = top.next; } } ============ PalindromeDemo.java import javax.swing.JOptionPane; public class PalindromeDemo { private static boolean isPalindrome(String input) { LinkedStackDS stack1 = new LinkedStackDS(); LinkedStackDS stack2 = new LinkedStackDS(); input = input.replaceAll("[^a-zA-Z]", "").toLowerCase(); //put the chars into stack1 for(int i = 0; i < input.length(); i++) { stack1.push(input.charAt(i)); } //now transfer all chars from stack1 to stack2, so stack2 is having original order fo chars from top while(!stack1.isEmptyStack()) { try { stack2.push(stack1.peek()); stack1.pop(); } catch (StackUnderflowException e) { System.out.println(e.getMessage()); } } //again push chars from input into stack1. it has reveseorder of chars from top for(int i = 0; i < input.length(); i++) { stack1.push(input.charAt(i)); } //compare the 2 stack tops till they are empty while(!stack1.isEmptyStack()) { try { if(!stack1.peek().equals(stack2.peek())) return false; stack1.pop(); stack2.pop(); } catch (StackUnderflowException e) { System.out.println(e.getMessage()); } } return true;//all matched } public static void main(String[] args) { String input; do { input = JOptionPane.showInputDialog("Input a string to check: "); if(input != null) { if(isPalindrome(input)) JOptionPane.showMessageDialog(null, input + " is a palindrome"); else JOptionPane.showMessageDialog(null, input + " is NOT a palindrome"); } }while(input != null); } }
- Provide these 5 (screenshots)sample outputs and tell if each is a palindrome or not.
Too bad--I hid a boot
Some men interpret eight memos
"Go Hang a Salami! I'm a Lasagna Hog"
(title of a book on palindromes by Jon Agee, 1991)
A man, a plan, a canal—Panama
Gateman sees my name, garageman sees name tag
LinkedStackADT.java
public interface LinkedStackADT<T> {
boolean isEmptyStack();
void push(T val);
T peek() throws StackUnderflowException;
void pop() throws StackUnderflowException;
}
=============
StackUnderflowException.java
public class StackUnderflowException extends Exception {
public StackUnderflowException(){}
public StackUnderflowException(String msg)
{
super(msg);
}
}
=========
LinkedStackDS.java
public class LinkedStackDS<T> implements LinkedStackADT<T>{
private class StackNode
{
T data;
StackNode next;
StackNode()
{
}
StackNode(T data, StackNode next)
{
this.data = data;
this.next = next;
}
public String toString()
{
return data.toString();
}
}
private StackNode top;
public LinkedStackDS() {
}
@Override
public boolean isEmptyStack() {
return top == null;
}
@Override
public void push(T val) {
top = new StackNode(val, top);
}
@Override
public T peek() throws StackUnderflowException {
if(isEmptyStack())
throw new StackUnderflowException("stack is empty");
return top.data;
}
@Override
public void pop() throws StackUnderflowException{
if(isEmptyStack())
throw new StackUnderflowException("stack is empty");
top = top.next;
}
}
============
PalindromeDemo.java
import javax.swing.JOptionPane;
public class PalindromeDemo {
private static boolean isPalindrome(String input)
{
LinkedStackDS<Character> stack1 = new LinkedStackDS<Character>();
LinkedStackDS<Character> stack2 = new LinkedStackDS<Character>();
input = input.replaceAll("[^a-zA-Z]", "").toLowerCase();
//put the chars into stack1
for(int i = 0; i < input.length(); i++)
{
stack1.push(input.charAt(i));
}
//now transfer all chars from stack1 to stack2, so stack2 is having original order fo chars from top
while(!stack1.isEmptyStack())
{
try {
stack2.push(stack1.peek());
stack1.pop();
} catch (StackUnderflowException e) {
System.out.println(e.getMessage());
}
}
//again push chars from input into stack1. it has reveseorder of chars from top
for(int i = 0; i < input.length(); i++)
{
stack1.push(input.charAt(i));
}
//compare the 2 stack tops till they are empty
while(!stack1.isEmptyStack())
{
try {
if(!stack1.peek().equals(stack2.peek()))
return false;
stack1.pop();
stack2.pop();
} catch (StackUnderflowException e) {
System.out.println(e.getMessage());
}
}
return true;//all matched
}
public static void main(String[] args) {
String input;
do
{
input = JOptionPane.showInputDialog("Input a string to check: ");
if(input != null)
{
if(isPalindrome(input))
JOptionPane.showMessageDialog(null, input + " is a palindrome");
else
JOptionPane.showMessageDialog(null, input + " is NOT a palindrome");
}
}while(input != null);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

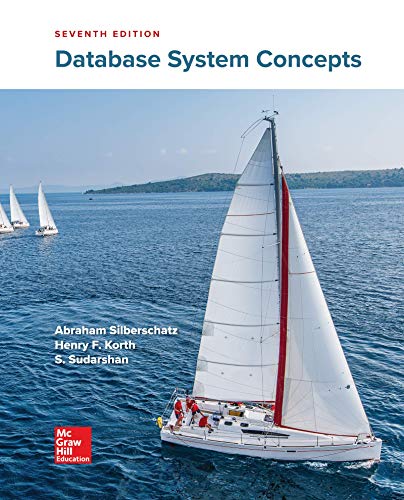
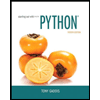
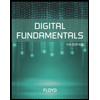
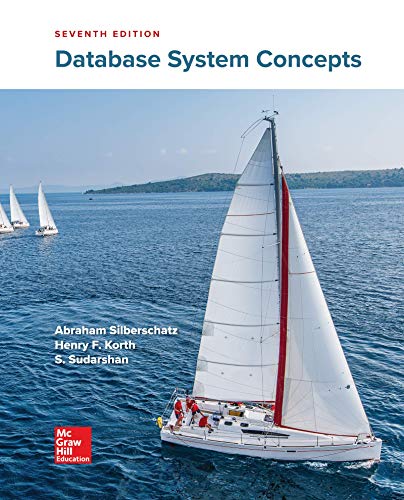
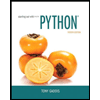
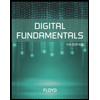
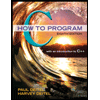
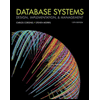
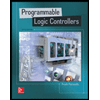