public boolean bubbleIns ( double element ); /* Another form of ins operation. In this one, we look through the list to see if the element we want to add is already in the list. If it is, we take the first occurrance of it and move that element to the head of the list. If the element we want to add is NOT already in the list then we put the new element at the head. The net effect on size is this: if the element is already there, the size does not change; if the element is not already there, then we add it as a new head so the size goes up one. Return true if the add succeeds (it almost always will); return false if for some reason the add cannot happen (such as in Array Implementation, the array has no room for a new element to be stored). If the list is empty, just insert the element. example: list is 11.3, 45.1, -5.6, 17.3 bubbleIns(21.0) makes this list: 21.0, 11.3, 45.1, -5.6, 17.3 list is 11.3, 45.1, -5.6, 17.3 bubbleIns(-5.6) makes this list: -5.6, 11.3, 45.1, 17.3 list is 11.3, 45.1, -5.6, 17.3, 45.1, 11.3 bubbleIns(45.1) makes this list: 45.1, 11.3, -5.6, 17.3, 45.1, 11.3 */ }
java language
solve this method
*******************
public boolean bubbleIns (double element) {
//your code here
}
description and class in pictures
please and thank you!!!
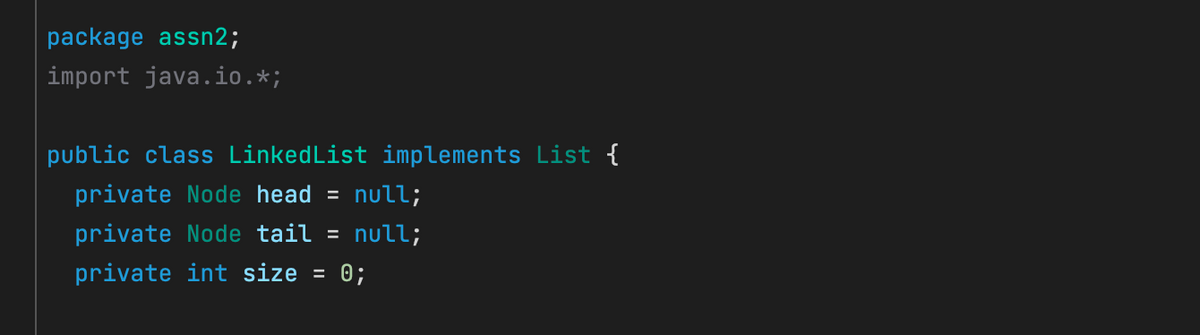
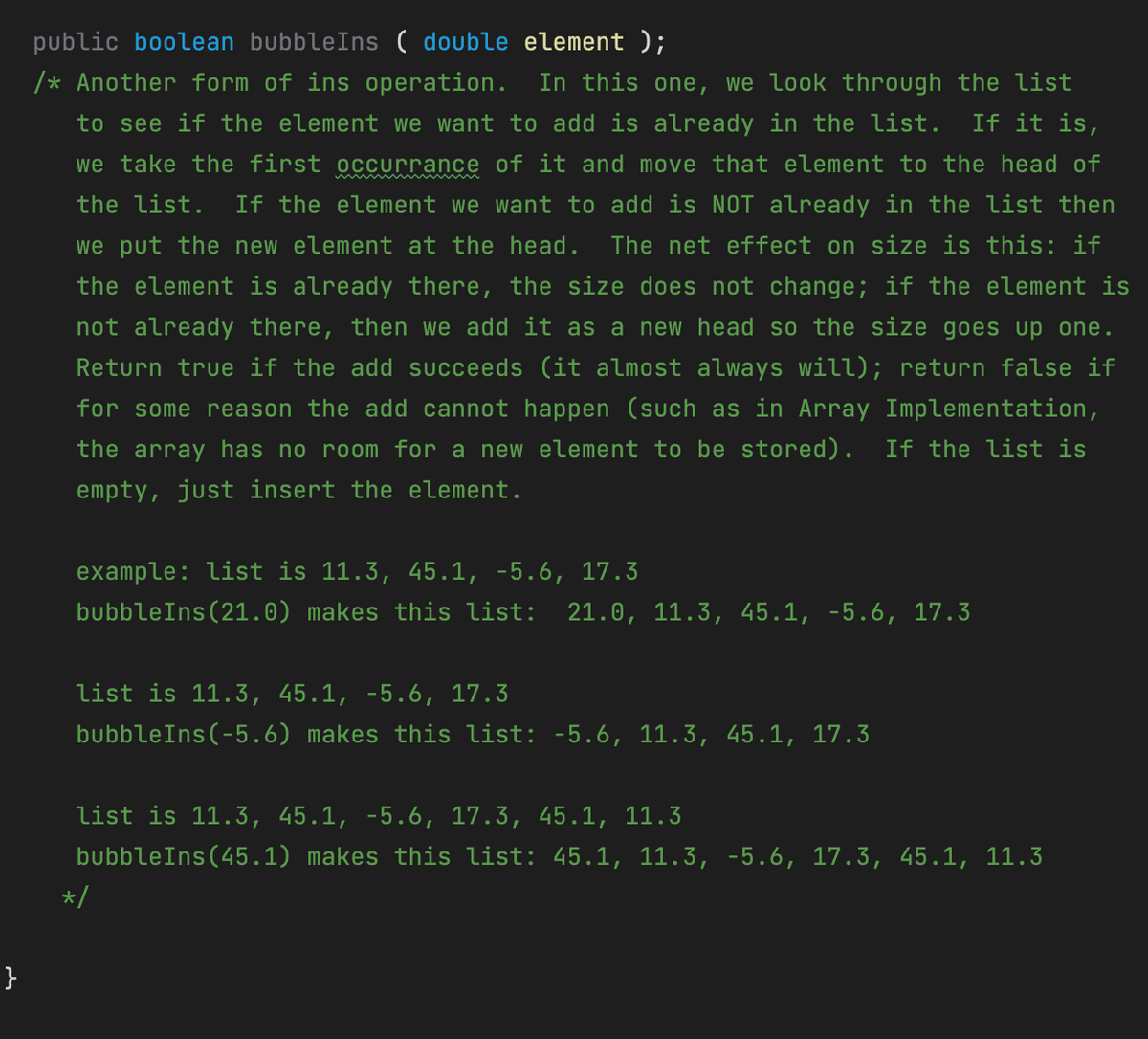

Step 1 : Start
Step 2 : Define an array in which the numbers are stored and a variable to hold the size of the array.
Step 3 : Define a method bubbleIns() which takes a number as argument and inserts it at the beginning position.
Step 4 : Using a for loop traverse the elements of the array.
Step 5 : Checking if the element is already present in the array, if so then note the index and shift all the elements by one place and inserting the element at the beginning of the array then removing the element already present in array from the index. Return True.
Step 6 : If the element is not present in the array already then shifting all the element of the list by one place and then Inserting the number at the beginning of the array.
Step 7 : Return false in none of the conditions match with the given criteria.
Step 8 : In the main method Initializing the array elements and set the size of the array.
Step 9 : Print the Array elements before insertion.
Step 10 : Calling the bubbleIns() method and passing the element to be inserted in the list. Storing the returned value in a variable.
Step 11 : If the method passes True then printing the list after the Insertion.
Step 12 : If false is returned then Printing an error message to the user.
Step 13 : Stop
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

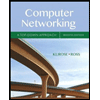
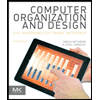
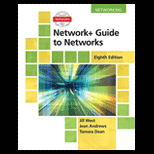
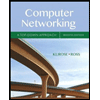
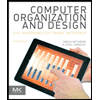
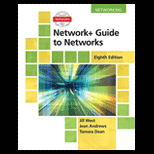
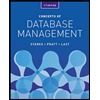
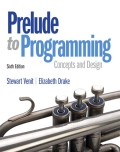
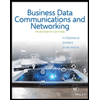