Put the client program in a separate file from the class, and divide the class into specification file (fraction.h) and implementation file (fraction.cpp), so your code will be in 3 separate files. Fraction.h #include using namespace std; int gcd(int a, int b) { if (a == 0) return b; if (b == 0) return a; if (a == b) return a; if (a > b) return gcd(a - b, b); return gcd(a, b - a); } class Fraction { private: // numerator and denominator int n, d; public: // simplifies the fraction void simplify() { int g = gcd(n, d); n /= g; d /= g; } // default constructor Fraction() { n = 0, d = 1; } // parameterised constructor Fraction(int a, int b) { n = a, d = b; simplify(); } // prints the fration as n/d void print() const { cout << n << "/" << d; } // multiplies 2 fractions and returns it Fraction multipliedBy(Fraction f) const { f.simplify(); Fraction r; r.n = n * f.n; r.d = d * f.d; r.simplify(); return r; } // divides two fraction and returns it Fraction dividedBy(Fraction& f) { Fraction r; r.n = n * f.d; r.d = d * f.n; r.simplify(); return r; } // adds two fractions and returns it Fraction addedTo(Fraction& f) { Fraction r; r.n = n * f.d + f.n * d; r.d = d * f.d; r.simplify(); return r; } // subtracts two fractions and returns it Fraction subtract(Fraction& f) { Fraction r; r.n = n * f.d - f.n * d; r.d = d * f.d; r.simplify(); return r; } // checks if both fractions are equal or not bool isEqualTo(Fraction& f) { f.simplify(); return (f.n == n && f.d == d); d); } }; Here is the client program. #include #include "Fraction.h" using namespace std; int main() { Fraction f1(9,8); Fraction f2(2,3); Fraction result; cout << "The result starts off at "; result.print(); cout << endl; cout << "The product of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.multipliedBy(f2); result.print(); cout << endl; cout << "The quotient of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.dividedBy(f2); result.print(); cout << endl; cout << "The sum of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.addedTo(f2); result.print(); cout << endl; cout << "The difference of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.subtract(f2); result.print(); cout << endl; if (f1.isEqualTo(f2)){ cout << "The two Fractions are equal." << endl; } else { cout << "The two Fractions are not equal." << endl; } const Fraction f3(12, 8); const Fraction f4(202, 303); result = f3.multipliedBy(f4); cout << "The product of "; f3.print(); cout << " and "; f4.print(); cout << " is "; result.print(); cout << endl; }
Put the client program in a separate file from the class, and divide the class into specification file (fraction.h) and implementation file (fraction.cpp), so your code will be in 3 separate files.
Fraction.h
#include <iostream>
using namespace std;
int gcd(int a, int b) {
if (a == 0) return b;
if (b == 0) return a;
if (a == b) return a;
if (a > b)
return gcd(a - b, b);
return gcd(a, b - a);
}
class Fraction {
private:
// numerator and denominator
int n, d;
public:
// simplifies the fraction
void simplify() {
int g = gcd(n, d);
n /= g;
d /= g;
}
// default constructor
Fraction() {
n = 0, d = 1;
}
// parameterised constructor
Fraction(int a, int b) {
n = a, d = b;
simplify();
}
// prints the fration as n/d
void print() const {
cout << n << "/" << d;
}
// multiplies 2 fractions and returns it
Fraction multipliedBy(Fraction f) const {
f.simplify();
Fraction r;
r.n = n * f.n;
r.d = d * f.d;
r.simplify();
return r;
}
// divides two fraction and returns it
Fraction dividedBy(Fraction& f) {
Fraction r;
r.n = n * f.d;
r.d = d * f.n;
r.simplify();
return r;
}
// adds two fractions and returns it
Fraction addedTo(Fraction& f) {
Fraction r;
r.n = n * f.d + f.n * d;
r.d = d * f.d;
r.simplify();
return r;
}
// subtracts two fractions and returns it
Fraction subtract(Fraction& f) {
Fraction r;
r.n = n * f.d - f.n * d;
r.d = d * f.d;
r.simplify();
return r;
}
// checks if both fractions are equal or not
bool isEqualTo(Fraction& f) {
f.simplify();
return (f.n == n && f.d == d);
d);
}
};
Here is the client program.
#include <iostream> #include "Fraction.h" using namespace std; int main() { Fraction f1(9,8); Fraction f2(2,3); Fraction result; cout << "The result starts off at "; result.print(); cout << endl; cout << "The product of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.multipliedBy(f2); result.print(); cout << endl; cout << "The quotient of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.dividedBy(f2); result.print(); cout << endl; cout << "The sum of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.addedTo(f2); result.print(); cout << endl; cout << "The difference of "; f1.print(); cout << " and "; f2.print(); cout << " is "; result = f1.subtract(f2); result.print(); cout << endl; if (f1.isEqualTo(f2)){ cout << "The two Fractions are equal." << endl; } else { cout << "The two Fractions are not equal." << endl; } const Fraction f3(12, 8); const Fraction f4(202, 303); result = f3.multipliedBy(f4); cout << "The product of "; f3.print(); cout << " and "; f4.print(); cout << " is "; result.print(); cout << endl; }

Step by step
Solved in 2 steps

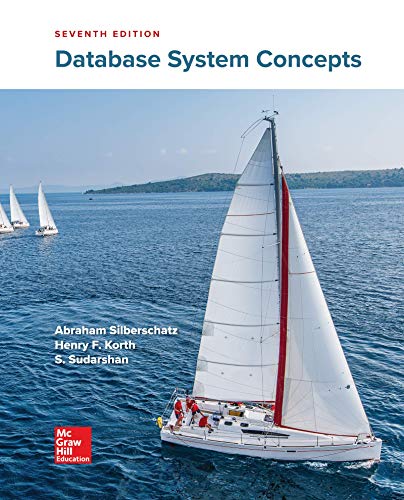
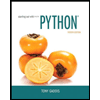
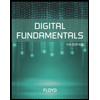
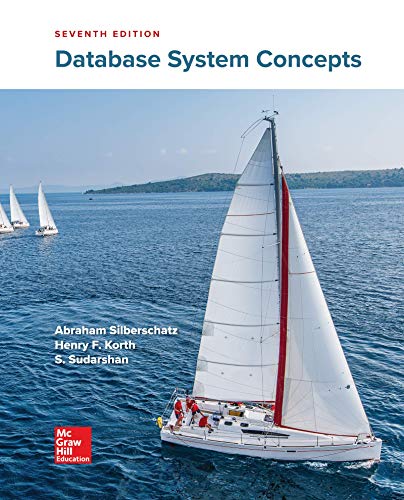
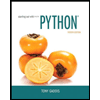
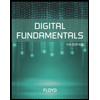
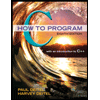
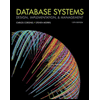
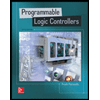