Python: Diet
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Python: Diet
![Meow' eats a lot, and she loves to know in gory detail what she eats in every meal. As her assistant, you
are going to implement a function
mealCal(meal: list[str], recipes: list[str], db: list[str]) -> float
that operates as follows:
• The parameter meal is a list of strings, listing the dishes she is having. There may be redundant
items: if Meow likes it enough, she may consume multiple servings of the same dish. For example,
meal
["T-Bone", "T-Bone", "Green Salad1"].
• The parameter recipes is a list of strings, representing a "book" of recipes. For example",
recipes =
["Pork Stew:Cabbage*5,Carrot*1,Fatty Pork*10",
"Green Salad1:Cabbage*10, Carrot*2,Pineapple*5",
"T-Bone:Carrot*2,Steak Meat*1"]
Each item is a string indicating the name of the dish, followed by a colon, then a comma-separated
list of ingredient names together with their quantities. In the example presented, the item
"T-Bone:Carrot*2,Steak Meat*1"](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48954d8a-c361-4523-b68b-ac12d6ced482%2Fe4158905-7726-411e-b8aa-ff90b4094ffb%2F4uldj9j_processed.png&w=3840&q=75)
Transcribed Image Text:Meow' eats a lot, and she loves to know in gory detail what she eats in every meal. As her assistant, you
are going to implement a function
mealCal(meal: list[str], recipes: list[str], db: list[str]) -> float
that operates as follows:
• The parameter meal is a list of strings, listing the dishes she is having. There may be redundant
items: if Meow likes it enough, she may consume multiple servings of the same dish. For example,
meal
["T-Bone", "T-Bone", "Green Salad1"].
• The parameter recipes is a list of strings, representing a "book" of recipes. For example",
recipes =
["Pork Stew:Cabbage*5,Carrot*1,Fatty Pork*10",
"Green Salad1:Cabbage*10, Carrot*2,Pineapple*5",
"T-Bone:Carrot*2,Steak Meat*1"]
Each item is a string indicating the name of the dish, followed by a colon, then a comma-separated
list of ingredient names together with their quantities. In the example presented, the item
"T-Bone:Carrot*2,Steak Meat*1"
![indicates that the dish "T-Bone" uses 2 units of Carrot and 1 unit of Steak Meat.
• The parameter db is a list of strings, representing a database of how much carbohydrate, protein,
and fat each ingredient contains. Each item is listed as the ingredient's name, followed by a colon,
and then three comma-separated numbers (int or float) denoting, respectively, the amounts of carb,
protein, and fat in grams. Here is an example:
["Cabbage:4,2 , 0", "Carrot:9,1,5", "Fatty Pork:431,1,5",
"Pineapple:7,1,0", "Steak Meat:5,20,10", "Rabbit Meat:7,2,20"].
db
As a specific example, the entry for Cabbage indicates that 1 unit of "Cabbage" has 4g of carbohy-
drate, 3g of protein, and 0g of fat.
• The function then returns the amount of calories resulted from eating this meal. Remember that
of carbohydrate yields 4 calories.
Each gram of protein yields 4 calories.
Each gram of fat yields 9 calories.
Each
gram
To give a complete example, plugging in the sample combination just described into mealCal, we
have that mealCal(meal, recipes, db) computes the following:
First, we derive the amount of calories Meow gets from a T-Bone dish. This dish has 2 carrots
and 1 unit of steak meat, so that's (9·4+1·4+5•9) × 2 = 85 × 2 = 170 calories from carrots and
5.4+ 20·4+ 10.9 = 190 calories from the meat. Hence, this dish has 170+ 190 = 360 calories.
- Then, we derive the amount of calories Meow gets from her other T-Bone dish. The amount is
the same: 360 calories.
- Then, we derive the amount of calories Meow gets from her salad dish: (4 ·4+2·4) × 10+ (9.
4+1.4+5.9) × 2+ (7 ·4+ 1·4+0•9) × 5 = 240+ 170+ 160 = 570.
Hence, mealCal, in this case, will return 1290.0.
Remarks:
• Your answers may be floating-point numbers; we'll accept any answers within 10-5 of our model
answers.
• The input ensures: (1) every dish in Meow's meal exists in the recipe; and (2) every ingredient
used exists in the db.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48954d8a-c361-4523-b68b-ac12d6ced482%2Fe4158905-7726-411e-b8aa-ff90b4094ffb%2F8ggyeec_processed.png&w=3840&q=75)
Transcribed Image Text:indicates that the dish "T-Bone" uses 2 units of Carrot and 1 unit of Steak Meat.
• The parameter db is a list of strings, representing a database of how much carbohydrate, protein,
and fat each ingredient contains. Each item is listed as the ingredient's name, followed by a colon,
and then three comma-separated numbers (int or float) denoting, respectively, the amounts of carb,
protein, and fat in grams. Here is an example:
["Cabbage:4,2 , 0", "Carrot:9,1,5", "Fatty Pork:431,1,5",
"Pineapple:7,1,0", "Steak Meat:5,20,10", "Rabbit Meat:7,2,20"].
db
As a specific example, the entry for Cabbage indicates that 1 unit of "Cabbage" has 4g of carbohy-
drate, 3g of protein, and 0g of fat.
• The function then returns the amount of calories resulted from eating this meal. Remember that
of carbohydrate yields 4 calories.
Each gram of protein yields 4 calories.
Each gram of fat yields 9 calories.
Each
gram
To give a complete example, plugging in the sample combination just described into mealCal, we
have that mealCal(meal, recipes, db) computes the following:
First, we derive the amount of calories Meow gets from a T-Bone dish. This dish has 2 carrots
and 1 unit of steak meat, so that's (9·4+1·4+5•9) × 2 = 85 × 2 = 170 calories from carrots and
5.4+ 20·4+ 10.9 = 190 calories from the meat. Hence, this dish has 170+ 190 = 360 calories.
- Then, we derive the amount of calories Meow gets from her other T-Bone dish. The amount is
the same: 360 calories.
- Then, we derive the amount of calories Meow gets from her salad dish: (4 ·4+2·4) × 10+ (9.
4+1.4+5.9) × 2+ (7 ·4+ 1·4+0•9) × 5 = 240+ 170+ 160 = 570.
Hence, mealCal, in this case, will return 1290.0.
Remarks:
• Your answers may be floating-point numbers; we'll accept any answers within 10-5 of our model
answers.
• The input ensures: (1) every dish in Meow's meal exists in the recipe; and (2) every ingredient
used exists in the db.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
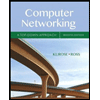
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
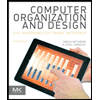
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
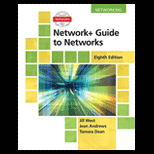
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
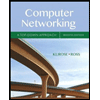
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
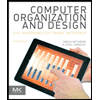
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
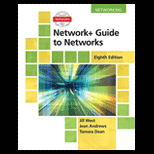
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
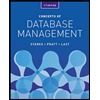
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
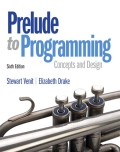
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
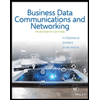
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY