Python program Write a class named Library. A library has the following attributes: • name: the name of this library. • employees: a list of LibraryEmployees that work at the library. • books: a dictionary that contains Books as keys. The value paired with each key is either an empty string, or a string representing the name of a person who has checked out the Book. The Library class should have an initializer that MAY accept employees and books as arguments, but doesn’t have to do so. If provided, these values should be assigned to the object’s respective attributes. If not provided, employees and books should begin as an empty list and dictionary, respectively. The value for the name attribute is always provided. The class should also have the following methods: • hire_employee(): takes in a new LibraryEmployee, and adds it to this library’s list of employees. • add_book(): takes in a new Book, and adds it to this library’s books (unless the library already has this book). If the library already has the book, print out the name of the library followed by “ already has this book!”. • check_out_book(): takes in a Book and a person’s name. If this book is in the dictionary of books, change the value at that key in the books dictionary to the person’s name. Otherwise, print out “Sorry, we don’t have ” followed by the name of the book and a period. • return_book(): takes in a Book. If this book is in the dictionary of books, change the value at that key in the books dictionary to an empty string. Otherwise, print out “Sorry, wrong library.”. • __str__() : return the name of the library. Create a driver program to call 3 instances and set and eventually display information. Create a book file that will be read for the list of books available currently in the library. Create an employee output file for the employees added recently.
Python program
Write a class named Library. A library has the following attributes:
• name: the name of this library.
• employees: a list of LibraryEmployees that work at the library.
• books: a dictionary that contains Books as keys. The value paired with each key is either an
empty string, or a string representing the name of a person who has checked out the Book.
The Library class should have an initializer that MAY accept employees and books as arguments,
but doesn’t have to do so. If provided, these values should be assigned to the object’s
respective attributes. If not provided, employees and books should begin as an empty list and
dictionary, respectively. The value for the name attribute is always provided.
The class should also have the following methods:
• hire_employee(): takes in a new LibraryEmployee, and adds it to this library’s list of
employees.
• add_book(): takes in a new Book, and adds it to this library’s books (unless the library
already has this book). If the library already has the book, print out the name of the library
followed by “ already has this book!”.
• check_out_book(): takes in a Book and a person’s name. If this book is in the dictionary of
books, change the value at that key in the books dictionary to the person’s name. Otherwise,
print out “Sorry, we don’t have ” followed by the name of the book and a period.
• return_book(): takes in a Book. If this book is in the dictionary of books, change the value
at that key in the books dictionary to an empty string. Otherwise, print out “Sorry, wrong
library.”.
• __str__() : return the name of the library.
Create a driver program to call 3 instances and set and eventually display information.
Create a book file that will be read for the list of books available currently in the library.
Create an employee output file for the employees added recently.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

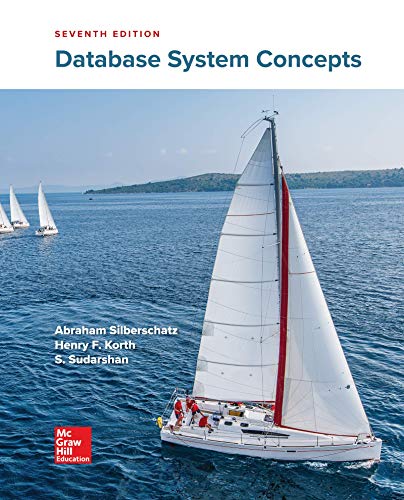
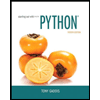
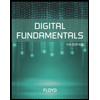
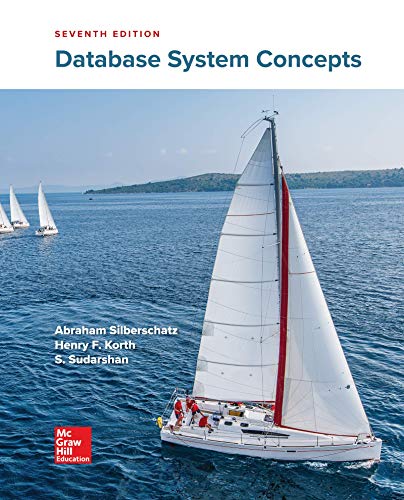
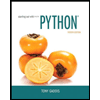
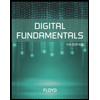
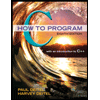
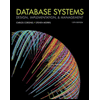
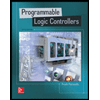