PYTHON: read.py from pathlib import Path import csv from sportclub import SportClub from typing import List, Tuple def readFile(file: Path) -> List[Tuple[str, str, str]]: """Read a CSV file and return its content. A good CSV file will have the header "City,Team Name,Sport" and appropriate content. Args: file: a path to the file to be read Returns: a list of tuples that each contain (city, name, sport) of the SportClub Raises: ValueError: if the reading csv has missing data (empty fields)""" # TODO: Complete the function def readAllFiles() -> List[SportClub]: """Read all the csv files in the current working directory to create a list of SportClubs that contain unique SportClubs with their corresponding counts. Take all the csv files in the current working directory, calls readFile(file) on each of them, and accumulates the data gathered into a list of SportClubs.Create a new file called "report.txt" in the current working directory containing the number of good files and good lines read. Create a new file called "error_log.txt" in the current working directory containing the name of the error/bad files read.Returns: a list of unique SportClub objects with their respective counts """ # TODO: Complete the function sportclub.py class SportClub: """A simple class to store and handle information about SportClubs. Attributes: city (str): The city the SportClub is based in. name (str): The name of the SportClub. sport (str): The sport the club plays. count (int): The amount of time the SportClub has been seen. Todo: complete the __eq__ and __lt__ functions of this class """ def __init__(self, city: str = "", name: str = "", sport: str= "", count: int = 0) -> None: self.setCity(city) self.setName(name) self.setSport(sport) self.count = count def setName(self, name: str) -> None: self.name = name def setCity(self, city: str) -> None: self.city = city def setSport(self, sport: str) -> None: self.sport = sport def getName(self) -> str: return self.name.title() def getCity(self): return self.city.title() def getSport(self): return self.sport.upper() def getCount(self): return self.count def incrementCount(self) -> None: self.count += 1 def __hash__(self) -> int: unique_identifier = (self.getCity(), self.getName(), self.getSport()) return hash(unique_identifier) def __str__(self) -> str: return f"Name: {self.getCity()} {self.getName()}, Sport: {self.getSport()}, Count: {self.getCount()}" def __eq__(self, o: object) -> bool: """Check if another object is equal to self. Returns: True if they are equal, False otherwise """ # TODO: Complete the function, which can be useful for ordering a List of Sportclub objects # hint: object o may not be of type SportClub. def __lt__(self, o: object) -> bool: """Check if self is less than another object.
PYTHON:
read.py
from pathlib import Path
import csv
from sportclub import SportClub
from typing import List, Tuple
def readFile(file: Path) -> List[Tuple[str, str, str]]:
"""Read a CSV file and return its content. A good CSV file will have the header "City,Team Name,Sport" and appropriate content. Args: file: a path to the file to be read Returns: a list of tuples that each contain (city, name, sport) of the SportClub Raises: ValueError: if the reading csv has missing data (empty fields)"""
# TODO: Complete the function
def readAllFiles() -> List[SportClub]:
"""Read all the csv files in the current working directory to create a list of SportClubs that contain unique SportClubs with their corresponding counts. Take all the csv files in the current working directory, calls readFile(file) on each of them, and accumulates the data gathered into a list of SportClubs.Create a new file called "report.txt" in the current working directory containing the number of good files and good lines read. Create a new file called "error_log.txt" in the current working directory containing the name of the error/bad files read.Returns: a list of unique SportClub objects with their respective counts
"""
# TODO: Complete the function
sportclub.py
class SportClub:
"""A simple class to store and handle information about SportClubs.
Attributes:
city (str): The city the SportClub is based in.
name (str): The name of the SportClub.
sport (str): The sport the club plays.
count (int): The amount of time the SportClub has been seen.
Todo:
complete the __eq__ and __lt__ functions of this class
"""
def __init__(self, city: str = "", name: str = "", sport: str= "", count: int = 0) -> None:
self.setCity(city)
self.setName(name)
self.setSport(sport)
self.count = count
def setName(self, name: str) -> None:
self.name = name
def setCity(self, city: str) -> None:
self.city = city
def setSport(self, sport: str) -> None:
self.sport = sport
def getName(self) -> str:
return self.name.title()
def getCity(self):
return self.city.title()
def getSport(self):
return self.sport.upper()
def getCount(self):
return self.count
def incrementCount(self) -> None:
self.count += 1
def __hash__(self) -> int:
unique_identifier = (self.getCity(), self.getName(), self.getSport())
return hash(unique_identifier)
def __str__(self) -> str:
return f"Name: {self.getCity()} {self.getName()}, Sport: {self.getSport()}, Count: {self.getCount()}"
def __eq__(self, o: object) -> bool:
"""Check if another object is equal to self.
Returns:
True if they are equal, False otherwise
"""
# TODO: Complete the function, which can be useful for ordering a List of Sportclub objects
# hint: object o may not be of type SportClub.
def __lt__(self, o: object) -> bool:
"""Check if self is less than another object.
Returns:
True if self is less than o, False otherwise
"""
# TODO: Complete the function, which can be useful for ordering a List of Sportclub objects
# hint: object o may not be of type SportClub.
write.py
import csv
from sportclub import SportClub
from typing import List, Iterable
def separateSports(all_clubs: List[SportClub]) -> Iterable[List[SportClub]]:
"""Separate a list of SportClubs into their own sports. For example, given the list [SportClub("LA", "Lakers", "NBA"), SportClub("Houston", "Rockets", "NBA"), SportClub("LA", "Angels", "MLB")],
return the iterable [[SportClub("LA", "Lakers", "NBA"), SportClub("Houston", "Rockets", "NBA")], [SportClub("LA", "Angels", "MLB")]] Args: all_clubs: A list of SportClubs that contain SportClubs of 1 or more sports. Returns: An iterable of lists of sportclubs that only contain clubs playing the same sport.
"""
# TODO: Complete the function
def sortSport(sport: List[SportClub]) -> List[SportClub]:
"""Sort a list of SportClubs by the inverse of their count and their name. For example, given the list [SportClub("Houston", "Rockets", "NBA", 80), SportClub("LA", "Warriors", "NBA", 130), SportClub("LA", "Lakers", "NBA", 130)] return the list [SportClub("LA", "Lakers", "NBA", 130), SportClub("LA", "Warriors", "NBA", 130), SportClub("Houston", "Rockets", "NBA", 80)] Args: sport: A list of SportClubs that only contain clubs playing the same sport Returns: A sorted list of the SportClubs
"""
# TODO: Complete the function
# hint: check documentation for sorting lists
def outputSports(sorted_sports: Iterable[List[SportClub]]) -> None:
"""Create the output csv given an iterable of list of sorted clubs. Create the csv "survey_database.csv" in the current working directory, and output the information: "City,Team Name,Sport,Number of Times Picked" for the top 3 teams in each sport. Args: sorted_sports: an Iterable of different sports, each already sorted correctly
"""
# TODO: Complete the function
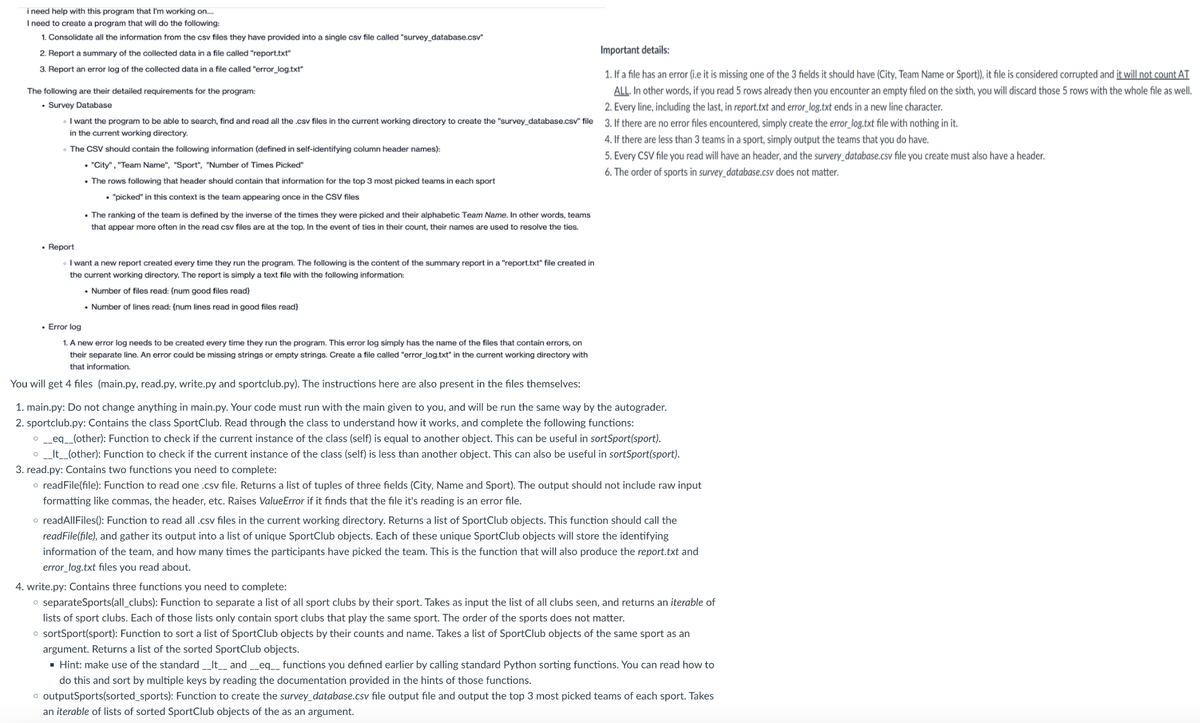
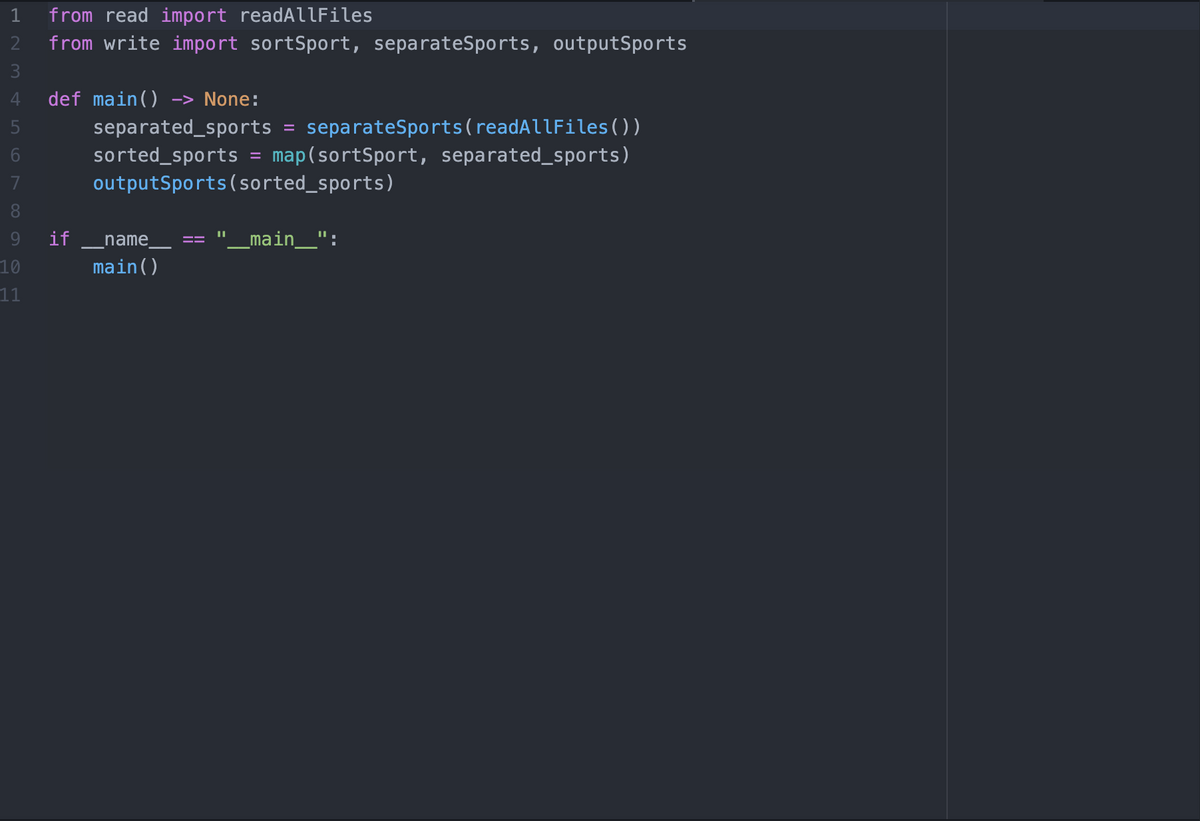

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

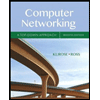
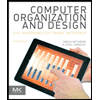
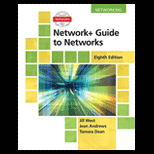
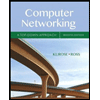
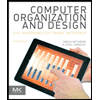
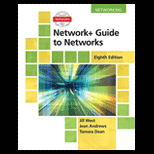
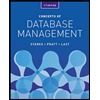
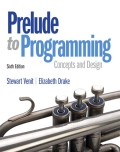
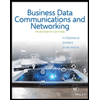