q.utoronto.ca/courses/249645/assignments/834167 We have provided two poetry form description files, poetry forms.txt and poetry forms small.txt, as example poetry form description files. The first is used by the Poetry Form Checker program poetry program. py while the second is used in doctest examples. We will test your code with these and other poetry form description files. er Note: Many poetry forms don't have a fixed number of lines. Instead, they specify what a stanza looks like, and then the poetry is ormation made up of as many stanzas as the poet likes. We will not consider stanza-based poems in this assignment. ng Software Data Representation We use the following Python definitions to create new types relevant to the problem domain. Read the comments in starter code file poetry constants.py for detailed descriptions with examples. ncements aments Type variables defined in poetry constants.. py POEM LINE str e Hours POEM List[POEM_LINE] PHONEMES Tuple(str] Us PRONUNCIATION DICT Dict [str, PHONEMES] hing Lab punts and nputer Labs POETRY_FORM DESCRIPTION Tuple [Tuple [int], Tuple[str]] POETRY_FORM DICT Dict (str, POETRY FORM DESCRIPTION] Valid Input earch Activities For all poetry samples used in this assignment, you should assume that all words in the poems will appear as keys in the pronunciation dictionary. We will test with other pronunciation dictionaries, but we will always follow this rule. hon Style Guide
from typing import List, Tuple, Dict
from poetry_constants import (POEM_LINE, POEM, PHONEMES, PRONUNCIATION_DICT,
POETRY_FORM_DESCRIPTION)
# ===================== Provided Helper Functions =====================
def transform_string(s: str) -> str:
"""Return a new string based on s in which all letters have been
converted to uppercase and punctuation characters have been stripped
from both ends. Inner punctuation is left untouched.
>>> transform_string('Birthday!!!')
'BIRTHDAY'
>>> transform_string('"Quoted?"')
'QUOTED'
>>> transform_string('To be? Or not to be?')
'TO BE? OR NOT TO BE'
"""
punctuation = """!"'`@$%^&_-+={}|\\/,;:.-?)([]<>*#\n\t\r"""
result = s.upper().strip(punctuation)
return result
def is_vowel_phoneme(phoneme: str) -> bool:
"""Return True if and only if phoneme is a vowel phoneme. That is, whether
phoneme ends in a 0, 1, or 2.
Precondition: len(phoneme) > 0 and phoneme.isupper()
>>> is_vowel_phoneme('AE0')
True
>>> is_vowel_phoneme('DH')
False
>>> is_vowel_phoneme('IH2')
True
"""
return phoneme[-1] in '012'
# ===================== Add Your Helper Functions Here =====================
# ===================== Required Functions =================================
# Functions related to syllable counts
def get_syllable_count(poem_line: POEM_LINE,
words_to_phonemes: PRONUNCIATION_DICT) -> int:
"""Return the number of syllables in poem_line by using the pronunciation
dictionary words_to_phonemes.
Precondition: len(poem_line) > 0
>>> line = 'Then! the #poem ends.'
>>> word_to_phonemes = {'THEN': ('DH', 'EH1', 'N'),
... 'ENDS': ('EH1', 'N', 'D', 'Z'),
... 'THE': ('DH', 'AH0'),
... 'POEM': ('P', 'OW1', 'AH0', 'M')}
>>> get_syllable_count(line, word_to_phonemes)
5
"""
def check_syllable_counts(poem_lines: POEM,
description: POETRY_FORM_DESCRIPTION,
word_to_phonemes: PRONUNCIATION_DICT) \
-> List[POEM_LINE]:
"""Return a list of lines from poem_lines that do NOT have the right
number of syllables as specified by the poetry form description, according
to the pronunciation dictionary word_to_phonemes. If all lines have the
right number of syllables, return the empty list.
Precondition: len(poem_lines) == len(description[0])
>>> poem_lines = ['The first line leads off,',
... 'With a gap before the next.', 'Then the poem ends.']
>>> description = ((5, 5, 4), ('*', '*', '*'))
>>> word_to_phonemes = {'NEXT': ('N', 'EH1', 'K', 'S', 'T'),
... 'GAP': ('G', 'AE1', 'P'),
... 'BEFORE': ('B', 'IH0', 'F', 'AO1', 'R'),
... 'LEADS': ('L', 'IY1', 'D', 'Z'),
... 'WITH': ('W', 'IH1', 'DH'),
... 'LINE': ('L', 'AY1', 'N'),
... 'THEN': ('DH', 'EH1', 'N'),
... 'THE': ('DH', 'AH0'),
... 'A': ('AH0'),
... 'FIRST': ('F', 'ER1', 'S', 'T'),
... 'ENDS': ('EH1', 'N', 'D', 'Z'),
... 'POEM': ('P', 'OW1', 'AH0', 'M'),
... 'OFF': ('AO1', 'F')}
>>> check_syllable_counts(poem_lines, description, word_to_phonemes)
['With a gap before the next.', 'Then the poem ends.']
>>> poem_lines = ['The first line leads off,']
>>> description = ((0,), ('*'))
>>> check_syllable_counts(poem_lines, description, word_to_phonemes)
[]
"""
# Functions related to rhyming
def get_last_syllable(word_phonemes: PHONEMES) -> PHONEMES:
"""Return the last syllable from word_phonemes.
The last syllable in word_phonemes is formed from the last vowel phoneme
and any subsequent consonant phoneme(s) in word_phonemes, in the same
order as they appear in word_phonemes.
>>> get_last_syllable(('AE1', 'B', 'S', 'IH0', 'N', 'TH'))
('IH0', 'N', 'TH')
>>> get_last_syllable(('IH0', 'N'))
('IH0', 'N')
>>> get_last_syllable(('B', 'S'))
()
"""
Constants:
from typing import Dict, List, Tuple
"""
A list of type definitions used in the Poetry Form Checker.
"""
POEM_LINE = str
POEM = List[POEM_LINE]
"""
For example:
('G', 'UW1', 'F', 'IY0')
"""
PHONEMES = Tuple[str]
"""
For example, here is a (small) pronunciation dictionary:
{'DANIEL': ('D', 'AE1', 'N', 'Y', 'AH0', 'L'),
'IS': ('IH1', 'Z'),
'GOOFY': ('G', 'UW1', 'F', 'IY0')}
"""
PRONUNCIATION_DICT = Dict[str, PHONEMES]
"""
For example, a limerick has this poetry form description:
((8, 8, 5, 5, 8), ('A', 'A', 'B', 'B', 'A'))
"""
POETRY_FORM_DESCRIPTION = Tuple[Tuple[int], Tuple[str]]
Here is an example:
{'Haiku': ((5, 7, 5), ('*', '*', '*')),
'Limerick': ((8, 8, 5, 5, 8), ('A', 'A', 'B', 'B', 'A'))}
"""
POETRY_FORMS_DICT = Dict[str, POETRY_FORM_DESCRIPTION]
![q.utoronto.ca/courses/249645/assignments/834167
Vinter
We have provided two poetry form description files, poetry_forms.txt and poetry forms small.txt, as example poetry form description
files. The first is used by the Poetry Form Checker program poetry program.py while the second is used in doctest examples. We will
test your code with these and other poetry form description files.
ous
Note: Many poetry forms don't have a fixed number of lines. Instead, they specify what a stanza looks like, and then the poetry is
Information
made up of as many stanzas as the poet likes. We will not consider stanza-based poems in this assignment.
lling Software
Data Representation
ouncements
We use the following Python definitions to create new types relevant to the problem domain. Read the comments in starter code file
ignments
poetry constants.py for detailed descriptions with examples.
zza
Type variables defined in poetry constants.py
POEM LINE
str
ice Hours
POEM
List [POEM LINE]
ERS
PHONEMES
Tuple[str]
arkUs
PRONUNCIATION DICT
Dict [str, PHONEMES]
POETRY FORM DESCRIPTION Tuple [Tuple[int], Tuple[str]]
eaching Lab
ccounts and
Computer Labs
POETRY FORM DICT
Dict [str, POETRY FORM DESCRIPTION]
Valid Input
Cesearch Activities
For all poetry samples used in this assignment, you should assume that all words in the poems will appear as keys in the pronunciation
dictionary. We will test with other pronunciation dictionaries, but we will always follow this rule.
Python Style Guide
Required Functions
In starter code file poetry functions.py, Complete the following function definitions. In addition, you may add some helper functions to
aid with the implementation of these required functions.
Function name:
(Parameter tvpes) -> Return
Full Description (paraphrase to get a proper docstring description)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa58df17a-0cc8-466f-a4cb-6a827b4be732%2F3e1ffff9-7a04-4b23-89a9-76fbcbcaabcf%2Fy2ij77_processed.jpeg&w=3840&q=75)
![Function name:
(Parameter types)-> Return
Full Description (paraphrase to get a proper docstring description)
type
The first parameter represents a non-empty line from a poem that has had leading and trailing
whitespace removed. The second parameter represents a pronunciation dictionary. This function is
to return the number of syllables in the line from the poem. The number of syllables in a poem line
get syllable_count:
is the same as the number of vowel phonemes in the line.
Assume that the pronunciation for every word in the line may be found in the pronunciation
dictionary.
HINT: Method str.split() and helper functions transform_string() and is vowel_phoneme() may be
re
(POEM LINE, PRONUNCIATION DICT)-
> int
helpful.
The first parameter represents a poem that has no blank lines and has had leading and trailing
whitespace removed. The second parameter represents a poetry form description. And the third
parameter represents a pronunciation dictionary. This function is to return a list of the lines from
that do not have the right number of syllables for the poetry form description. The lines
check syllable_counts:
the
poem
(POEM, POETRY_FORM DESCRIPTION,
should appear in the returned list in the same order as they appear in the poem. If all lines have the
right number of syllables, return the empty list. The number of syllables in a line is the same as the
number of vowel phonemes in the line.
Recall that every line whose required syllable count value is o has no syllable count requirement to
PRONUNCIATION DICT) ->
List[POEM LINE ]
meet.
The parameter represents a tuple of phonemes. The function is to return a tuple that contains the
last vowel phoneme and any consonant phoneme(s) that follow it in the given tuple of phonemes.
The ordering must be the same as in the given tuple. The empty tuple is to be returned if the tuple
of phonemes does not contain a vowel phoneme.
ities
uide
get last syllable:
(PHONEMES)-> PHONEMES
HINT: Helper function is_vowel_phoneme() may be helpful.
The first parameter represents a word, as does the second parameter. The third parameter
represents a pronunciation dictionary. The function is to return whether or not the two words
words rhyme:
(str, str, PRONUNCIATION_DICT) -> rhyme, according to the pronunciation dictionary.
bool
Assume that the pronunciation for both words may be found in the pronunciation dictionary.
Recall that two words rhyme if and only if they have the same last syllable.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa58df17a-0cc8-466f-a4cb-6a827b4be732%2F3e1ffff9-7a04-4b23-89a9-76fbcbcaabcf%2Fpqq12ud_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps

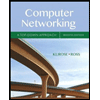
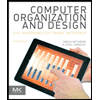
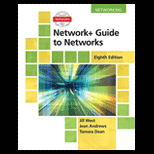
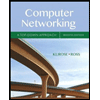
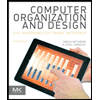
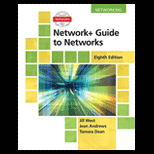
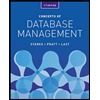
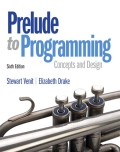
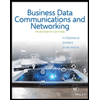