-
comFile1.txt
10
20
25
25
30
35
comFile2.txt
25
35
10
15
30
20
_____________________
#include <fstream>
#include <iostream>
using namespace std;
bool compareTwoArrays(int arr1[],int arr2[],int size);
int main()
{
//defines an input stream for the data file
ifstream dataIn;
int num,arr1Size=0,arr2Size=0;
//Opening the input file
dataIn.open("comFile1.txt");
//checking whether the file name is valid or not
if(dataIn.fail())
{
cout<<"** File Not Found **";
return 1;
}
else
{
//Calculating the size of an array
while(dataIn>>num)
{
arr1Size++;
}
dataIn.close();
// Creating array dynamically
int* arr1 = new int[arr1Size];
int i=0;
//Opening the input file
dataIn.open("comFile1.txt");
//calculating the no of elements in the file#1
while(dataIn>>num)
{
*(arr1+i)=num;
i++;
}
dataIn.close();
//Opening the input file
dataIn.open("comFile2.txt");
//checking whether the file name is valid or not
if(dataIn.fail())
{
cout<<"** File Not Found **";
return 1;
}
else
{
//calculating the no of elements in the file#1
while(dataIn>>num)
{
arr2Size++;
}
dataIn.close();
/* if the two files are not having same
* no of elements display error message
*/
if(arr1Size!=arr2Size)
{
cout<<"The Two arrays are not same."<<endl;
exit(1);
}
// Creating array dynamically
int* arr2 = new int[arr2Size];
i=0;
//Opening the input file
dataIn.open("comFile2.txt");
while(dataIn>>num)
{
*(arr2+i)=num;
i++;
}
dataIn.close();
//Calling the function by passing the two arrays as arguments
bool boolean=compareTwoArrays(arr1,arr2,arr1Size);
if(boolean)
cout<<"The Two arrays are same elements and multiplicity"<<endl;
else
cout<<"The Two arrays are not same "<<endl;
}
}
return 0;
}
/* This function compares the elements
* in the arrray and its multiplicity
*/
bool compareTwoArrays(int arr1[],int arr2[],int size)
{
int count=0;
for(int i=0;i<8;i++)
{
for(int j=0;j<8;j++)
{
if(*(arr1+i)==*(arr1+j))
count++;
}
//cout<<"Count "<<count<<endl;
for(int k=0;k<8;k++)
{
if(*(arr1+i)==*(arr2+k))
count--;
}
if(count!=0)
{
return 0;
}
}
return 1;
}
_____________________
Output:
Question: Using good OOP, write a C++ program that will compare two arrays to test for the same elements and multiplicity. To begin, populate the arrays with the input files, comFile1.txt and comFile2.txt. The elements in the arrays do not need to be in the same order for them to be considered similar. For example:
Please add comments in lines
Question: Using good OOP, write a C++ program that will compare two arrays to test for the same elements and multiplicity. To begin, populate the arrays with the input files, comFile1.txt and comFile2.txt. The elements in the arrays do not need to be in the same order for them to be considered similar. For example:
comFile1.txt TO array 1: 121 144 19 161 19 144 19 11
comFile2.txt TO array 2: 11 121 144 19 161 19 144 19
NOTE: Professor mentioned that the array Size should be 300 as eh will be testing the code with different files other than these two he has provided for examples.
would be considered to have the same elements because 19 appears 3 times in each array, 144 appears twice in each array, and all other elements appear once in each array.
Use pointer notation instead of array notation whenever possible.
Display whether or not the arrays have the same elements and multiplicity.
Use private member functions and variables.
Use public member functions for a constructor (where appropriate) and a driver method only.
These specifications do not give a list of method names to be used. It is assumed the program will use several methods doing one task each.
NOTE: Any submission that uses global variables or does not use a class and object appropriately will result in a project grade of 0.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

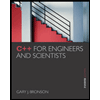
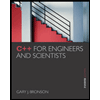