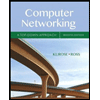
Problem solving using recursion
There are two skeleton programs, flesh them out following the suggestions given.
Recursive
Alternate (faster) algorithm: if n = 0, return 1.0 else if n is odd return x * xn-1 else { y = xn/2; return y * y;} (fast technique).
Run
Show all digits displayed by your program.
You could add a loop to your program and paste the screen shot for all input values mentioned here instead. Show all digits.
Base Exponent slow power fast power Math.pow
3.5 64
5 15
1.25 31
2 17
String matching
Finding needle in a haystack:
If needle is longer than the haystack then failure
else if needle matches the initial portion of haystack (use .startsWith() method) then success
else {
create a shorter haystack (haystack.substring(1))
return result from recursive call on the shorter haystack
}
You are allowed to use only the following methods from the Java String class: .length(), .startsWith(), .subString().
You are not allowed to use any other method from the String class. [The String class already has a find method. We are trying to implement that using simpler tools].
Fill results from power calculation in this page, copy paste source code and screen shots from the two finished programs in the following pages. Save the file with name yourUserid_ICA06.docx and submit through Isidore Assignment.
You could add a loop to your programs so you can show results from several inputs in one screen shot. Be sure to cover all possible situations for String Matching.
Submit this Word document filled in with source codes and screen shots in appropriate pages through Isidore assignments.
First code i solve it this is the second part
// define a scanner attached to keyboard available to all functions final static Scanner cin = new Scanner(System.in); /** * @param args the command line arguments */ public static void main(String[] args) { // TODO code application logic here out.print("CPS 151 ICA 04 String matching by YOUR NAME\n\n"); out.print("Enter the text (one word): "); String hayStack = cin.next(); out.print("Enter the pattern (one word): "); String needle = cin.next(); if (find(needle, hayStack)) out.println(needle + " was found in " + hayStack); else out.println(needle + " was not found in " + hayStack); } // end main private static boolean find(String needle, String hayStack) { int patLen = needle.length(); int textLen = hayStack.length(); // base case 1 if (patLen > textLen) { // failure } else if (needle.startsWith(needle)) { // base case 2 MODIFY!! // SUCCESS } else { // recurse } return false; // dummy to keep NetBeans happy } // end method
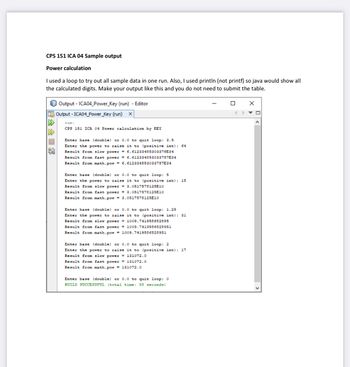
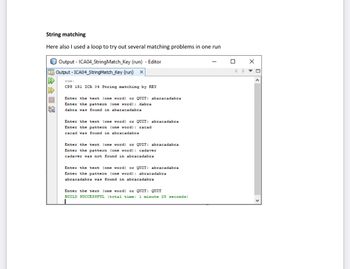

Java:
Java is a high level object oriented programming language.
It was developed by James Gosling at Sun Microsystem.
It is simple and easy to understand.
It is robust and secure.
It supports multithreading.
Note: As per the rules, I solve only first question.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- 8. Know how to do these, to trace functions like these and to debug functions like these: // recursive power , compute xn int exp(int x, int n){ if(n== return return *exp( ); } void main(){ int a,b; cin >>a>>b; cout>a; rev_print(a); }arrow_forwardGiven below is a recursive algorithm to compute r". The input r can be any real number. The input n is assumed to be a non-negative integer. Exponent ( r, n) #Input: Real number r and a non-negative integer n. +Output: r If (n-0), return (1) p: Exponent ( ? ) Return ( rp) //The base case 7/The recursive call What missing input values should be used in the recursive call? O (r, n) O (r, n-1) O (r-1, n) O (r-1. n-1)arrow_forwardDetermine the output of the following recursive function when n = 64. (show your working/steps) int func (int n) { if (n ==1) return 0 else return 2 + func ( ) }arrow_forward
- Simple MIPs Recursive Procedure Write a program that calls a recursive procedure. Inside this procedure, add 1 to a counter so you can verify the number of times it executes. Put a number in a MIPS counter that specifies the number of times you want to allow the recursion to continue. You can use a LOOPinstruction (or other conditional statements using MIPS), find a way for therecursive procedure to call itself a fixed number of times.arrow_forwardUsing recursion, write a function sum that takes a single argument n and computes the sum of all integers between 0 and n inclusive. Do not write this function using a while or for loop. Assume n is non-negative. def sum(n): """Using recursion, computes the sum of all integers between 1 and n, inclusive. Assume n is positive. >>> sum(1) 1 >>> sum(5) # 1 + 2 + 3+ 4+ 5 15 "*** YOUR CODE HERE ***"arrow_forwardRecursive algorithm always gives cleaner code with less cost. Group of answer choices True Falsearrow_forward
- Design and implement a recursive program(in java) to determine and print the Nth line of Pascal's triangle, as shown below. Each interior value is the sum of the two values above it. Hint: Use an array to store the values on each line.arrow_forwardWrite a recursive algorithm with the following prototype: int add (int x, int y); that returns x if y is 0; and adds x to y otherwise. THE FUNCTION MUST BE RECURSIVE. (hint: the base case should involve a test for y being 0; recursive case should reduce y towards 0)arrow_forward1.)I have to recursive with python language and was asked to do a buy 2 get 1 free where you have some amount of money(x) each item costs a given amount(y), for each 2 item you get 1 free, builda recursive solution to find out how many items you can buy for x amount of money. 2.) Buy M, get N free Then alter your first answer to allow for the user to enter any M and N for how many you need to buy to get free itemsarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
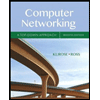
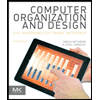
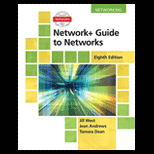
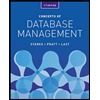
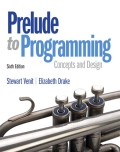
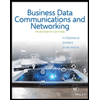